CODE to Copy & Paste #include #include #include using namespace std; void readStudData(ifstream &rss, int scores[], int id[], int &count, bool &tooMany); float mean(int scores[], int count); void printTable(int score[], int ID[], int count); void printGrade(int oneScore, float average); // Only accept MAX_SIZE entries, this will be the size of our arrays const int MAX_SIZE = 10; int main() { // variables. Need to create two int arrays: scores and ID. // Need to create one int: count. Need to create one bool: tooMany int scores[MAX_SIZE]; int ID[MAX_SIZE]; int count; bool tooMany; // Call readStudData to read data from the file that was opened above // inFile is the name of the file opened ifstream inFile; inFile.open("scores.txt"); readStudData(inFile, scores, ID, count, tooMany); // Don't forget to close the file inFile.close(); // If there are more than MAX_SIZE records in the file, then tooMany should be // set to true. We need to warn the user that not all the records were used if( tooMany ) cout << "\nWarning! Some data is missing\n"; // Print the data printTable(scores, ID, count); cout << endl; system("Pause"); return 0; } void readStudData(ifstream &rss, int scores[], int id[], int &count, bool &tooMany) { // Set tooMany to false and count to 0 tooMany = false; count = 0; while(!rss.eof()) { if(count == MAX_SIZE) break; rss >> id[count] >> scores[count]; count++; } // Determine if we have too many records in the file if( !rss.eof() && count == MAX_SIZE ) tooMany = true; } float mean(int scores[], int count) { // Store the sum of the scores here int sum = 0; // Loop through and accumulate the scores from the array for(int i = 0; i < count; i++) { sum += scores[i]; } // Return the average return (float)sum/count; } void printTable(int score[], int ID[], int count) { // Need the average float average = mean(score, count); // Display the average to the user cout << "Average: " << average << endl; // Display the header for the table cout << "ID\t" << "Score\t" << "Grade" << endl; for(int i = 0; i < count; i++) { cout << ID[i] << '\t' << score[i] << '\t'; printGrade(score[i], average); } } void printGrade(int oneScore, float average) { if(oneScore < average + 10 && oneScore > average - 10) cout << "Satisfactory\n"; else if(oneScore > average + 10) cout << "Outstanding\n"; else cout << "Unsatisfactory\n"; } TASK Redo using a struct to store each student’s data and an array of structs to store the whole class. The struct should have data members for id, score, and grade.
CODE to Copy & Paste
#include <iostream>
#include <iomanip>
#include <fstream>
using namespace std;
void readStudData(ifstream &rss, int scores[], int id[], int &count, bool &tooMany);
float mean(int scores[], int count);
void printTable(int score[], int ID[], int count);
void printGrade(int oneScore, float average);
// Only accept MAX_SIZE entries, this will be the size of our arrays
const int MAX_SIZE = 10;
int main()
{
// variables. Need to create two int arrays: scores and ID.
// Need to create one int: count. Need to create one bool: tooMany
int scores[MAX_SIZE];
int ID[MAX_SIZE];
int count;
bool tooMany;
// Call readStudData to read data from the file that was opened above
// inFile is the name of the file opened
ifstream inFile;
inFile.open("scores.txt");
readStudData(inFile, scores, ID, count, tooMany);
// Don't forget to close the file
inFile.close();
// If there are more than MAX_SIZE records in the file, then tooMany should be
// set to true. We need to warn the user that not all the records were used
if( tooMany ) cout << "\nWarning! Some data is missing\n";
// Print the data
printTable(scores, ID, count);
cout << endl;
system("Pause");
return 0;
}
void readStudData(ifstream &rss, int scores[], int id[], int &count, bool &tooMany)
{
// Set tooMany to false and count to 0
tooMany = false;
count = 0;
while(!rss.eof())
{
if(count == MAX_SIZE) break;
rss >> id[count] >> scores[count];
count++;
}
// Determine if we have too many records in the file
if( !rss.eof() && count == MAX_SIZE ) tooMany = true;
}
float mean(int scores[], int count)
{
// Store the sum of the scores here
int sum = 0;
// Loop through and accumulate the scores from the array
for(int i = 0; i < count; i++)
{
sum += scores[i];
}
// Return the average
return (float)sum/count;
}
void printTable(int score[], int ID[], int count)
{
// Need the average
float average = mean(score, count);
// Display the average to the user
cout << "Average: " << average << endl;
// Display the header for the table
cout << "ID\t" << "Score\t" << "Grade" << endl;
for(int i = 0; i < count; i++)
{
cout << ID[i] << '\t' << score[i] << '\t';
printGrade(score[i], average);
}
}
void printGrade(int oneScore, float average)
{
if(oneScore < average + 10 && oneScore > average - 10) cout << "Satisfactory\n";
else if(oneScore > average + 10) cout << "Outstanding\n";
else cout << "Unsatisfactory\n";
}
TASK
Redo using a struct to store each student’s data and an array of structs to store the whole class. The struct should have data members for id, score, and grade.

C++ Language
Python is slower than C++ because C++ is statically typed, which speeds up code compilation. Python employs the interpreter, which slows down compilation, and it supports dynamic typing, which makes it slower than C++.
Step by step
Solved in 3 steps with 1 images

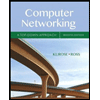
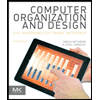
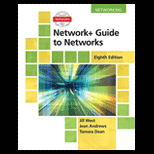
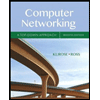
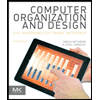
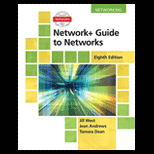
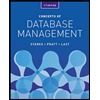
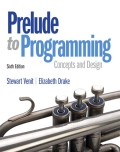
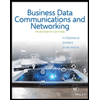