Start with the code in the File IO lecture for writing a file (creating a list of degrees and their radian equivalent in 10° steps). We will expand that code to calculate the radians in 2° steps. There will be five calculations per line and each line will represent one decade (steps of 10°). Below is the format I would like to see. Note there are header lines to so the table is labeled. You will have to use the printf/fprintf formatting codes to get the data to line up appropriately. Degrees to Radians in 2-degree steps Degrees 1-digit 0 4 8. 0.000 0.035 0.070 0.105 0.140 10 0.175 0.209 0.244 0.279 0.314 20 0.349 0.384 0.419 0.454 0.489 30 0.524 0.559 0.593 0.628 0.663 350 Submit the Engineering Development Work, code, and the file with your results.
Code in C
Code in the file IO:
/*************************************************************
This program prints a degree-to-radian table using a for-
loop structure. The results are printed to a file and the
the screen.
*************************************************************/
#include <stdio.h>
#define PI 3.141593
#define FILENAME "tableD2R.dat"
int main(void)
{
/* Declare variables. */
double radians;
FILE *fileout;
/* Open file. */
fileout = fopen(FILENAME,"w");
if (fileout == NULL)
printf("Error opening input file. \n");
else
{
/* Print radians and degrees in a loop. */
printf("Degrees to Radians \n");
for (int degrees=0; degrees<=360; degrees+=10)
{
radians = degrees*PI/180;
printf("%6i %9.6f \n",degrees,radians);
fprintf(fileout,"%6i %9.6f \n",degrees,radians);
}
/* Exit program. */
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

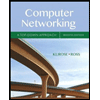
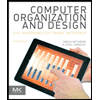
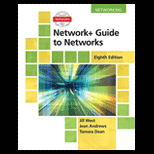
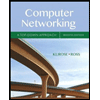
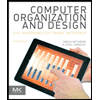
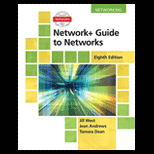
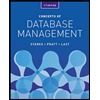
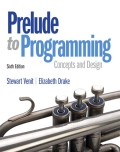
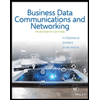