HELLO please solve in c++. SHOW THE OUTPUT. INSTRUCTIONS ARE DOWN BELOW PLEASE COMPLETE.
HELLO please solve in c++. SHOW THE OUTPUT. INSTRUCTIONS ARE DOWN BELOW PLEASE COMPLETE.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
HELLO please solve in c++. SHOW THE OUTPUT. INSTRUCTIONS ARE DOWN BELOW PLEASE COMPLETE.

Transcribed Image Text:1. Introduction
You will create a C++ program to solve a 2048 game. Read a file that has a matrix.
Then, by following the rules, you should find a movement sequence to merge the
numbers into one number. Output your movement sequence to a file.
2. Input and Output
1) Input file
The input file will never empty. It always contains two parts:
a) A number k (k>0, k is an integer) stands for the size
(size=width=height) of the matrix.
b) A group of numbers a; (aj >=0, a, is an integer, 1<=i<=k, 1<=j<=k),
stand for the cells of the matrix.
2) Output file
The output file contains the moves. It should not contain spaces in between
each direction number. If the input matrix cannot be merged to one number,
"Impossible" should be outputted (case sensitive).
3. The Rules and Operations
1) Create the matrix: By reading the input file, you should be able to create a
matrix filled with the numbers. Each number corresponds to one cell. After
reading the example 1 from below input11.txt, you should be able to create
a matrix as follow Table 1:
Table 1
4
2
8
2) Move the cells: You can move all the greater than 0 numbers (except for
2048) by applying one direction operation. The up, right, down and left
move were coded with numbers 1, 2. 3 and
If the initial matrix doesn't
need move (e.g., only one cell with a number greater than 0), use number
O as final output. After applying the number 2 (right) operation to the Table
1, the result should be as Table 2:
Table 2
2
4
0008
3) Merge operation: When moving numbers to one side, two identical
numbers can be merged to the double value of the numbers. The merge
only happens when one number is unmovable, while another identical
number still moving towards this unmovable number. For each move
operation, one number can only merge once. An example shows the
before and after of the number 2 (right) move operation as Table 3: The
two 4's in the right (after) matrix cannot be merged because the red 4 is
an immediate result of last merge of two number 2.
Table 3
2
2
4
4
4
32
16
32
16
before
after
4) The Walls: A special number 2048 will never move in the matrix. It can be
treated as a wall. It can block all the numbers along the coming direction.
The number 2048 doesn't represent a mergeable number, you don't need
to try to merge it. An example shows how it works after number 2 move
when a wall exists in Table 4:
Table 4
2048
0 8
2
2048
4
0 000
4
4
before
after
5) Requirement: You must use a stack to solve this problem.
6) Additional requirements: If you can solve the problem with multiple
sequences, you should output the sequence which with the smallest value.
For example, if the result has two achievable move sequences like 12345
and 132, you should output 132 only.
7) The total length of the move sequence will not exceed 12 movements.
4. Program specification and Examples
Your source code will be compiled and tested by the TAS. The result file should
be written to another text file (output file), provided with the command line.
Notice the input and output files are specified in the command line, not inside the
C++ code. All the necessary parameters will be in the command line when calling
your program, so you don't need to worry about the missing parameter problem.
When calling your program, the command format would be one of the two
standard types as
elov
Also notice
quotes in the program call.
The general call to the executable is as follows:
merge "input=input11.txt;output=Doutput11.txt"
Call example with another command line type.
merge input=input11.txt output=output11.txt
Example 1 of input and output
input11.txt
4
2400
2000
0000
0080
Command line:
merge input=input11.txt output3Doutput11.txt
output11.txt
122
Example 2 of input and output
input12.txt
5
00000
22 2048 4 4
00000
00000
04000
Command line:
merge "input=input12.txt;output=Doutput12.txt"
output12.txt
412
Example 3 of input and output
input13.txt
222
00
000
Command line:
merge input=input13.txt output3Doutput13.txt
output13.txt
Impossible
4.
2.
4-
2.
2.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 10 steps with 10 images

Recommended textbooks for you
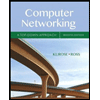
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
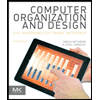
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
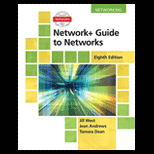
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
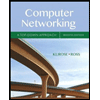
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
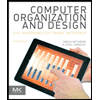
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
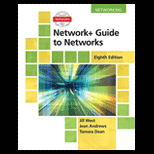
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
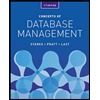
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
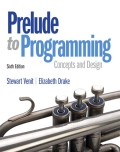
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
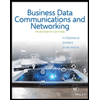
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY