A linked list is built in this lab. Make sure to keep track of the head node. ContactNode.java - Class definition ContactList.java - Contains main() method (2) Build the ContactNode class per the following specifications: Private fields String contactName String contactPhoneNumber ContactNode nextNodePtr Constructor with parameters for name followed by phone number Public member methods getName() - Accessor getPhoneNumber() - Accessor insertAfter() getNext() - Accessor printContactNode() Ex: If the name is Roxanne Hughes and the phone number is 443-555-2864, printContactNode() outputs: Name: Roxanne Hughes Phone number: 443-555-2864 (3) Define main() to read the name and phone number for three contacts and output each contact. Create three ContactNodes and use the nodes to build a linked list. Ex: If the input is: Roxanne Hughes 443-555-2864 Juan Alberto Jr. 410-555-9385 Rachel Phillips 310-555-6610 the output is: Person 1: Roxanne Hughes, 443-555-2864 Person 2: Juan Alberto Jr., 410-555-9385 Person 3: Rachel Phillips, 310-555-6610 (4) Output the linked list using a loop to output contacts one at a time. Ex: CONTACT LIST Name: Roxanne Hughes Phone number: 443-555-2864 Name: Juan Alberto Jr. Phone number: 410-555-9385 Name: Rachel Phillips Phone number: 310-555-6610 import java.util.Scanner; public class ContactList { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); ContactNode head = null; ContactNode current = null; // Read the name and phone number for three contacts and build a linked list. for (int i = 1; i <= 3; i++) { System.out.print(" " + i + ": "); String name = scnr.next(); System.out.print(" " + i + ": "); String phoneNumber = scnr.next(); ContactNode newContact = new ContactNode(name, phoneNumber); if (head == null) { head = newContact; current = head; } else { current.insertAfter(newContact); current = newContact; } } // Output each contact. current = head; for (int i = 1; current != null; i++) { System.out.print("Person " + i + ": "); current.printContactNode(); current = current.getNext(); } } } class ContactNode { private String contactName; private String contactPhoneNumber; private ContactNode nextNodePtr; public ContactNode(String name, String phoneNumber) { this.contactName = name; this.contactPhoneNumber = phoneNumber; this.nextNodePtr = null; } public String getName() { return contactName; } public String getPhoneNumber() { return contactPhoneNumber; } public ContactNode getNext() { return nextNodePtr; } public void insertAfter(ContactNode node) { ContactNode temp = this.nextNodePtr; this.nextNodePtr = node; node.nextNodePtr = temp; } public void printContactNode() { System.out.println("Name: " + contactName + " Phone number: " + contactPhoneNumber); } }
A linked list is built in this lab. Make sure to keep track of the head node.
- ContactNode.java - Class definition
- ContactList.java - Contains main() method
(2) Build the ContactNode class per the following specifications:
- Private fields
- String contactName
- String contactPhoneNumber
- ContactNode nextNodePtr
- Constructor with parameters for name followed by phone number
- Public member methods
- getName() - Accessor
- getPhoneNumber() - Accessor
- insertAfter()
- getNext() - Accessor
- printContactNode()
Ex: If the name is Roxanne Hughes and the phone number is 443-555-2864, printContactNode() outputs:
Name: Roxanne Hughes Phone number: 443-555-2864
(3) Define main() to read the name and phone number for three contacts and output each contact. Create three ContactNodes and use the nodes to build a linked list.
Ex: If the input is:
Roxanne Hughes 443-555-2864 Juan Alberto Jr. 410-555-9385 Rachel Phillips 310-555-6610
the output is:
Person 1: Roxanne Hughes, 443-555-2864 Person 2: Juan Alberto Jr., 410-555-9385 Person 3: Rachel Phillips, 310-555-6610
(4) Output the linked list using a loop to output contacts one at a time.
Ex:
CONTACT LIST Name: Roxanne Hughes Phone number: 443-555-2864 Name: Juan Alberto Jr. Phone number: 410-555-9385 Name: Rachel Phillips Phone number: 310-555-6610
import java.util.Scanner;
public class ContactList {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
ContactNode head = null;
ContactNode current = null;
// Read the name and phone number for three contacts and build a linked list.
for (int i = 1; i <= 3; i++) {
System.out.print(" " + i + ": ");
String name = scnr.next();
System.out.print(" " + i + ": ");
String phoneNumber = scnr.next();
ContactNode newContact = new ContactNode(name, phoneNumber);
if (head == null) {
head = newContact;
current = head;
} else {
current.insertAfter(newContact);
current = newContact;
}
}
// Output each contact.
current = head;
for (int i = 1; current != null; i++) {
System.out.print("Person " + i + ": ");
current.printContactNode();
current = current.getNext();
}
}
}
class ContactNode {
private String contactName;
private String contactPhoneNumber;
private ContactNode nextNodePtr;
public ContactNode(String name, String phoneNumber) {
this.contactName = name;
this.contactPhoneNumber = phoneNumber;
this.nextNodePtr = null;
}
public String getName() {
return contactName;
}
public String getPhoneNumber() {
return contactPhoneNumber;
}
public ContactNode getNext() {
return nextNodePtr;
}
public void insertAfter(ContactNode node) {
ContactNode temp = this.nextNodePtr;
this.nextNodePtr = node;
node.nextNodePtr = temp;
}
public void printContactNode() {
System.out.println("Name: " + contactName + " Phone number: " + contactPhoneNumber);
}
}



Step by step
Solved in 5 steps with 3 images

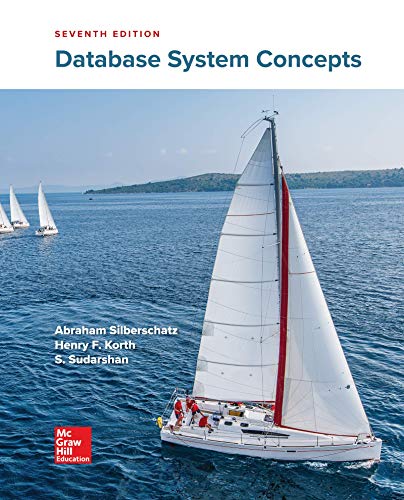
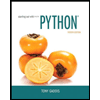
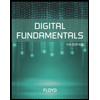
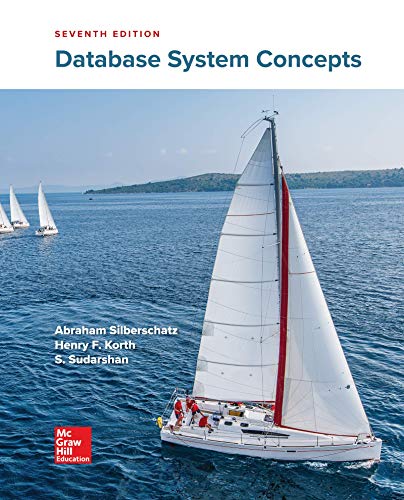
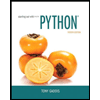
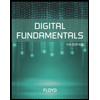
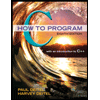
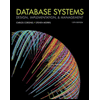
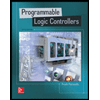