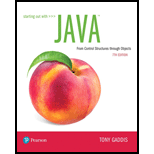
Concept explainers
SavingsAccount Class
Design a SavingsAccount class that stores a savings account’s annual interest rate and balance. The class constructor should accept the amount of the savings account’s starting balance. The class should also have methods for subtracting the amount of a withdrawal, adding the amount of a deposit, and adding the amount of monthly interest to the balance. The monthly interest rate is the annual interest rate divided by twelve. To add the monthly interest to the balance, multiply the monthly interest rate by the balance, and add the result to the balance.
Test the class in a
- a. Ask the user for the amount deposited into the account during the month. Use the class method to add this amount to the account balance.
- b. Ask the user for the amount withdrawn from the account during the month. Use the class method to subtract this amount from the account balance.
- c. Use the class method to calculate the monthly interest.
After the last iteration, the program should display the ending balance, the total amount of deposits, the total amount of withdrawals, and the total interest earned.

Learn your wayIncludes step-by-step video

Chapter 6 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Starting Out with Python (3rd Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Artificial Intelligence: A Modern Approach
Starting out with Visual C# (4th Edition)
Concepts of Programming Languages (11th Edition)
- C# Console Application for Minesweeper In this milestone, students will create three classes: Cell, Board, and Program. Create a class that models a game cell. A game cell should have the following properties: a.Its row and column. These should initially be set to -1. b.Its visited boolean value. This should initially be set to false. c.Live boolean value. This should initially be set to false. "Live" set to true will indicate that the cell is a "live bomb" cell. d.The number of neighbors that are "live." This should initially be set to 0. The Cell class should have a constructor, as well as getters and setters for all properties. 3.Create a class that models a game board. A game board should have the following properties: a.Size. The board will be square, where the size includes the dimensions of both the length and width of the board. b.Grid. The grid will be a 2-dimensional array of the type cell. c.Difficulty. A percentage of cells that will be set to "live" status. 4.The Board…arrow_forwardCould you please help me with this chapter 6 number 15 Programming challenge from Starting out with Java Gaddis 7th edition book. Thank youarrow_forward7)Circle Class Write a Circle class that has the following fields: radius: a double PI:a final double initialized with the value 3.14159 The class should have the following methods: Constructor. Accepts the radius of the circles as an argument. Constructor. A no-arg constructor that sets the radius field to 0.0. setRadius. A mutator method for the radius field. getRadius.An accessor method for the radius field. area. Returns the area of the circle, which is calculated as area. Returns the area of the circles, which is calculated as area= PI * radius * radius diameter. Returns the diameter of the cirlce, which is calculated as diameter = radius * 2 circumference. Returns the circumference of the circle, which is calculated as circumference = 2 * PI * radius Write a program that demonstrates the Circle class by asking the user for the circles's radius, creating a Circle object, and then reporting the cirlce's area, diameter, and circumference.arrow_forward
- Time This class is going to represent a 24-hour cycle clock time. That means 0:0:0 (Hour:Minute:Second) is the beginning of a day and 23:59:59 is the end of the day. This class has these data members. int sec int min int hourarrow_forwardTrue or False It is legal to write a class without any constructors.arrow_forwardTrue or FalseThe same rules for naming variables apply to naming classes.arrow_forward
- Month Class Exceptions Write a class named Month. The class should have an int field named monthNumber that holds the number of the month. For example, Janauary would be 1, February would be 2, and so forth. In addition, provide the following methods: A no-arg constructor that sets the monthNumber field to 1. A constructor that accepts the number of the month as an argument. It should set the monthNumber value to the value passed as the argument. If a value less than 1 or greater than 12 is passed, the constructor should throw an InvalidMonthNumberException. A constructor that accepts the name of the month, such as “January” or “February”, as an argument. It should set the monthNumber field to the correct corresponding value. If an invalid name is passed, the constructor should throw an InvalidMonthNameException. A setMonthNumber method that accepts an int argument, which is assigned to the monthNumber field. If a value less than 1 or greater than 12 is passed, throw an…arrow_forwardFreezing and Boiling PointsThe following table lists the freezing and boiling points of several substances.Substance Freezing Point || Boiling PointEthyl Alcohol -173 || 172Oxygen -362 || -306Water 32 || 212Design a class that stores a temperature in a temperature field and has the appropriate accessor and mutator method for the field. In addition to appropriate constructors, the class should have the following methods:• isEthylFreezing. This method should return the boolean value true if the tempera-ture stored in the temperature field is at or below the freezing point of ethyl alcohol. Otherwise, the method should return false.• isEthylBoiling. This method should return the boolean value true if the tempera-ture stored in the temperature field is at or above the boiling point of ethyl alcohol. Otherwise, the method should return false.• isOxygenFreezing. This method should return the boolean value true if the tem-perature stored in the temperature field is at or below the freezing…arrow_forwardC++ Language Activity 5: DERIVED CLASS • Create a SAVING ACCOUNT class that inherits the class BANK ACCOUNT. • Create a constructor for the derived class that accepts INTEREST RATE. • Create a method that displays the INTEREST RATE. • Create a method that computes the INTEREST (BALANCE * INTEREST RATE) and adds to the current BALANCE.arrow_forward
- Parking Ticket SimulatorFor this assignment you will design a set of classes that work together to simulate apolice officer issuing a parking ticket. The classes you should design are:• The Parkedcar Class: This class should simulate a parked car. The class's respon-sibilities are:To know the car's make, model, color, license number, and the number of min-utes that the car has been parkedThe BarkingMeter Class: This class should simulate a parking meter. The class'sonly responsibility is:- To know the number of minutes of parking time that has been purchased• The ParkingTicket Class: This class should simulate a parking ticket. The class'sresponsibilities areTo report the make, model, color, and license number of the illegally parked carTo report the amount of the fine, which is $2S for the first hour or part of anhour that the car is illegally parked, plus $10 for every additional hour or part ofan hour that the car is illegally parkedTo report the name and badge number of the police…arrow_forwardCruise Recreational Activities Example Recreational activities include things like aerobics, shuffle board, and swimming. Each activity is identified by an activity code and includes other information such as description. Classes are offered for each activity. A class is uniquely identified by a combination of the activity code and the day/time at which it is held. It is assumed that a specific class will never be offered for the same activity at the same day and time, although it could be offered on a different day and/or time. Other information about a class includes the enrollment limit and the current enrollment count. A class will never include more than one activity. A passenger can sign up for a class as long as there is sufficient room in the class. Passengers are identified by a unique passenger number. Other information stored about passengers includes name, address, and age. Passengers have no limit on the number and type of classes they can sign up for. When they…arrow_forwardPlease help me with this Java chapter 6 programming challenge. Thank youarrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
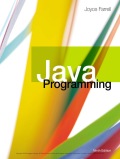
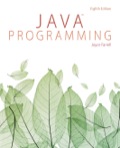
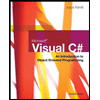
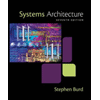