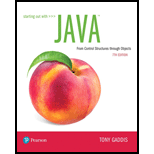
Concept explainers
Roulette Wheel Colors
On a roulette wheel, the pockets are numbered from 0 to 36. The colors of the pockets are as follows:
- Pocket 0 is green.
- For pockets 1 through 10, the odd numbered pockets are red and the even numbered pockets are black.
- For pockets 11 through 18, the odd numbered pockets are black and the even numbered pockets are red.
- For pockets 19 through 28, the odd numbered pockets are red and the even numbered pockets are black.
- For pockets 29 through 36, the odd numbered pockets are black and the even numbered pockets are red.
Write a class named RoulettePocket. The class’s constructor should accept a pocket number. The class should have a method named getPocketColor that returns the pocket’s color, as a string.
Demonstrate the class in a

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Electric Circuits. (11th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
Starting Out with Python (4th Edition)
- I would like to know the features of BranchCache, Metadata, and LPR Port Monitorarrow_forwardPlease answer the JAVA OOP questions below: How do arrays of objects differ from arrays of primitive types? Why and when would you use an array of objects? What are the different ways to initialize an array of objects? What is a static variable in Java? How does its scope differ from instance variables? When should you use static variables? Provide examples where static variables are beneficial over instance variables. What is the difference between static and non-static methods in the Data Definition Class? What are the benefits of using static methods?arrow_forwardAssume you are a loyal member of Costco. You have been expecting your membership reward from the company, and you just received a text message on your mobile phone. The message appears to be from Costco: Costco - Your 3% return is ready: costco.wholesaledividend.com Before clicking the link in the message, you consider whether this is genuinely from Costco or a phishing attack on you. How can you tell? If it is a phishing attack, what mechanism was most likely used to send the message to you? What actions can you take if you are not sure whether it is a genuine message from Costco? What actions can you take if you are sure this is a phishing attack?arrow_forward
- RSA and Diffie-Hellman are important algorithms in public-key cryptography. What are the differences between the two? Assume you intercept the ciphertext C = 105 sent to a user whose public key is e = 7, n = 403. Explain in detail how you will find the private key of the user and crack the ciphertext. What is the plaintext M?arrow_forwardCBC-Pad is a block cipher mode of operation commonly used in block ciphers. CBC-Pad handles plain text of any length. Padding is used to ensure that the plaintext input is a multiple of the block length. Hence, the ciphertext is longer than the plaintext by at most the size of a single block.Assume that the original plaintext is 556 bytes and the size of a cipher block is 28 bytes. What will be the padding? If the original plaintext is an integer multiple of the block size, will padding still be needed? Why or why not?arrow_forwardAbstract classes & Interfaces (Ch13) 5. See the code below and solve the following. class Circle { protected double radius; // Default constructor public Circle() ( } this(1.0); // Construct circle with specified radius public Circle(double radius) { } this.radius radius; // Getter method for radius public double getRadius() { } return radius; // Setter method for radius public void setRadius(double radius) { } this.radius = radius; // Implement the findArea method defined in GeometricObject public double findArea() { } return radius* radius * Math. PI; // Implement the find Perimeter method defined in GeometricObject public double findPerimeter() { } return 2*radius*Math.PI; // Override the equals() method defined in the Object class public boolean equals(Circlel circle) { } return this.radius == circle.getRadius(); // Override the toString() method defined in the Object class public String toString() { } } return "[Circle] radius = " + radius; 5-1. Define a class name…arrow_forward
- 6. What is Race condition? How to prevent it? [2 marks] 7. How many synchronization methods do you know and compare the differences. [2 marks] 8. Explain what are the “mutual exclusion”, “deadlock”, “livelock”, and “eventual entry”, with the traffic intersection as an example like dinning philosophy. [2 marks] 9. For memory allocation, what are the difference between internal fragmentation and external fragmentation. Explain with an example. [2 marks] 10. How can the virtual memory map to the physical memory. Explain with an example. [2 marks]arrow_forwardYour answers normally have 50 words. Less than 50 words will not get marks. 1. What is context switch between multiple processes? [2 marks] 2. Draw the memory layout for a C program. [2 marks] 3. How many states does a process has? [2 marks] 4. Compare the non-preemptitve scheduling and preemptive scheduling. [2 marks] 5. Given 4 process and their arrival times and next CPU burst times, what are the average times and average Turnaround time, for different scheduling algorithms including: a. First Come, First-Served (FCFS) Scheduling [2 marks] b. Shortest-Job-First (SJF) Scheduling [2 marks] c. Shortest-remaining-time-first [2 marks] d. Priority Scheduling [2 marks] e. Round Robin (RR) [2 marks] Process Arrival Time Burst Time P1 0 8 P2 1 9 P3 3 2 P4 5 4arrow_forwarda database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]arrow_forward
- a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]arrow_forwardIf a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? Order, Product, store, customer.arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
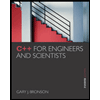
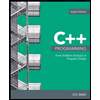
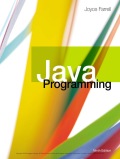
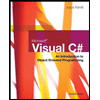
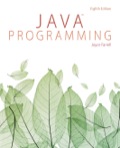