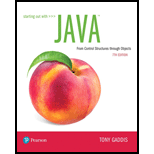
Temperature Class
Write a Temperature class that will hold a temperature in Fahrenheit, and provide methods to get the temperature in Fahrenheit, Celsius, and Kelvin. The class should have the following field:
- fahrenheit – A double that holds a Fahrenheit temperature.
The class should have the following methods:
- Constructor – The constructor accepts a Fahrenheit temperature (as a double) and stores it in the ftemp field.
- setFahrenheit – The setFahrenheit method accepts a Fahrenheit temperature (as a double) and stores it in the ftemp field.
- getFahrenheit – Returns the value of the ftemp field, as a Fahrenheit temperature (no conversion required).
- celsius – Returns the value of the ftemp field converted to Celsius.
- kelvin – Returns the value of the ftemp field converted to Kelvin.
Use the following formula to convert the Fahrenheit temperature to Celsius:
Use the following formula to convert the Fahrenheit temperature to Kelvin:
Demonstrate the Temperature class by writing a separate

Learn your wayIncludes step-by-step video

Chapter 6 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Electric Circuits. (11th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Mechanics of Materials (10th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
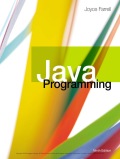
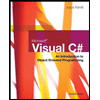