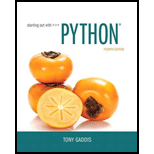
Concept explainers
Blackjack Simulation
Previously in this chapter you saw the card_dealer.py
▪ Numeric cards are assigned the value they have printed on them. For example, the value of the 2 of spades is 2, and the value of the 5 of diamonds is 5.
▪ Jacks, queens, and kings are valued at 10.
▪ Aces are valued at 1 or 11, depending on the player's choice.
The program should deal cards to each player until one player’s hand is worth more than 21 points. When that happens, the other player is the winner. (It is possible that both players’ hands will simultaneously exceed 21 points, in which case neither player wins.) The program should repeat until all the cards have been dealt from the deck. If a player is dealt an ace, the program should decide the value of the card according to the following rule: The ace will be worth 11 points, unless that makes the player’s hand exceed 21 points. In that case, the ace will be worth 1 point.

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Starting Out with Python (4th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out with C++ from Control Structures to Objects (9th Edition)
SURVEY OF OPERATING SYSTEMS
Management Information Systems: Managing The Digital Firm (16th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
- No aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forwardWhat are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward
- 2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forwardGiven the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NFarrow_forwardConsider the ER diagram of online sales system above. Based on the diagram answer the questions below, 1. Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key 2. Is there a direct relationship that exists between Store and Customer entities? AnswerYes/No?arrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer thequestions below, 1. Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M 2. Which one of the 4 Entities mention in the diagram can have a recursive relationship? 3. If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)? NO AI use pencil and paperarrow_forwardSTEP 1: The skeleton Let's start by creating a skeleton for some of the classes you will need. • Write a class called Tile. You can think of a tile as a square on the board on which the game will be played. We will come back to this class later. For the moment you can leave it empty while you work on creating classes that represents characters in the game. • Write an abstract class Fighter which has the following private fields: - A Tile field named position, representing the fighter's position in the game. A double field named health, representing the fighter's health points (HP). An int field named weaponType, representing the type of weapon the fighter is using. This value is used to rank different weapon types: higher values indicate higher weapon ranks. -An int field named attackDamage, representing the fighter's attack power. The class must also have the following public methods: 3 A constructor that takes as input a Tile indicating the position of the fighter, a double…arrow_forwardA company database needs to store information about employees (identified by SIN, with salary and phone as attributes), departments (identified by DID, with dname and budget as attributes), and children of employees (with name and age as attributes). Employees work in departments; each department is managed by an employee; a child must be identified uniquely by name when the parent (who is an employee; assume that only one parent works for the company) is known. We are not interested in information about a child once the parent leaves the company. Draw an ER diagram using Crows Foot notation that captures this information. Important: Must submit both ER Diagram and Relational Schema images in your solution here.arrow_forward
- Given the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined. Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]arrow_forward1. Using one of the method described in class and/or textbook (Section 9.1) convert the following regular expression into a state transition diagram: (0+ 10*1)* (01 + 10) Indicate in your answer how did you arrive at the result as follows: Write down all the state transition diagrams that you constructed for all the subexpressions and clearly indicate which diagram corresponds to which expression. Do not simplify any state transition diagram. 2. Consider the following state transition diagram over Σ = {a,b}: b A a a C b B a a b D За a Using the method described in class and in the textbook (Section 9.2) convert the diagram into an equivalent regular expression. Include all the intermediate steps in your answer. 3. Are the languages L1, L2, and L3 below over the alphabet Σ = {a, b, c} regular or non-regular? Justify your answer carefully. (a) L₁ = {a¹b2jc²i : i ≥ 0, j > 2} (b) L₂ = L₁n {akbm c³p: k,m,p≥ 0} (c) L3 = {a²ib²j+1 : i,j ≥ 0}^{akbm c³p : k,m,p ≥ 0}arrow_forward(1 point) By dragging statements from the left column to the right column below, give a proof by induction of the following statement: an = = 9" - 1 is a solution to the recurrence relation an = 9an-18 with ao = : 0. The correct proof will use 8 of the statements below. Statements to choose from: Note that a₁ = 9a0 + 8. Now assume that P(n) is true for all n ≥ 0. Your Proof: Put chosen statements in order in this column and press the Submit Answers button. Let P(n) be the predicate, "a = 9″ – 1". απ = 90 − 1 = Note that Let P(n) be the predicate, "an 9" - 1 is a solution to the recurrence relation an = 9an-1 +8 with ao = 0." - Now assume that P(k + 1) is true. Thus P(k) is true for all k. Thus P(k+1) is true. Then ak+1 = 9ak +8, so P(k + 1) is true. = 1 − 1 = 0, as required. Then = 9k — 1. ak Now assume that P(k) is true for an arbitrary integer k ≥ 1. By the recurrence relation, we have ak+1 = ak+1 = = 9ak + 8 = 9(9k − 1) + 8 This simplifies to 9k+19+8 = 9k+1 − 1 Then 9k+1 − 1 = 9(9*…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
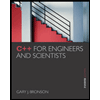
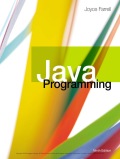
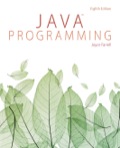
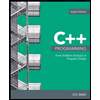
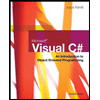