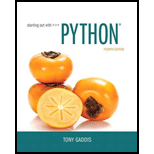
Word Index
Write a
▪ Key. The keys are the individual words found in the file.
▪ Values. Each value is a list that contains the line numbers in the file where the word (the key) is found.
For example, suppose the word “robot” is found in lines 7, 18, 94, and 138. The dictionary would contain an element in which the key was the string “robot", and the value was a list containing the numbers 7, 18, 94, and 138.
Once the dictionary is built, the program should create another text file, known as a word index, listing the contents of the dictionary. The word index file should contain an alphabetical listing of the words that are stored as keys in the dictionary, along with the line numbers where the words appear in the original file. Figure 9-1 shows an example of an original text file (Kennedy.txt) and its index file (index.txt).
Figure 9-1 Example of original file and index file

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Starting Out with Python (4th Edition)
- I have a few questions I need help with Statement: When we build a nearest neighbor model, we shall not remove the redundant dummies when coding a categorical variable. True or False Statement: One reason why a neural network model often requires a significant number of data observations to train is that it often has a significant number of model parameters to estimate even if there are only a few predictors. True or False. Which of the following statements about confusion matrix is wrong A) Confusion matrix is a performance measure for probability prediction techniques B) Confusion matrix is derived based on classification rules with cut-off value 0.5 C) Confusion matrix is derived based on training partition to measure a model’s predictive performance D) None of the abovearrow_forwardStudent ID is 24241357arrow_forwardWhich of the following methods help when a model suffers from high variance? a. Increase training data. b. Increase model size. c. Decrease the amount of regularization. d. Perform feature selection.arrow_forward
- 57 Formula 1 point Use shift folding, length 3, on the following value to calculate the Hash Value. 114184121 Type your answer...arrow_forwardWrite a program that reads a list of 10 integers, and outputs those integers in reverse. For coding simplicity, follow each output integer by a space, including the last one. Then, output a newline. Ex: If the input is: 2 4 6 8 10 12 14 16 18 20 the output is: 20 18 16 14 12 10 8 642 To achieve the above result, first read the integers into an array. Then output the array in reverse. 623802 1031906 nx3zmv7.arrow_forward6.3B-2. Multiple Access protocols (2). Consider the figure below, which shows the arrival of 6 messages for transmission at different multiple access nodes at times t=0.1, 0.8, 1.35, 2.6, 3.9, 4.2. Each transmission requires exactly one time unit. 1 2 3 4 t=0.0 t=1.0 t=2.0 t=3.0 5 6 t=4.0 t=5.0 For the slotted ALOHA protocol, indicate which packets are successfully transmitted. You can assume that if a packet experiences a collision, a node will not attempt a retransmission of that packet until sometime after t=5. 1 2 3 4 5 Karrow_forward
- Problem of checking Compile errors Runtime errors ======== } ng; } You have the following IQueue interface. Implement a Queue class derived from IQueue. You can use STL containers discussed in class, such as vector, queue, stack, deque, map. #include using namespace std; class IQueue { public: }; virtual void Enqueue(int val) virtual int Dequeue() = 0; virtual int Size() const = 0; int main() = 0; { Queue q; ===== } cout << q.Size() << endl; q. Enqueue(10); q.Enqueue(20); q. Enqueue(30); cout << q.Size() << endl; cout << q.Dequeue() << endl; cout << q.Size() << endl; cout << q.Dequeue() << endl; cout << q.Size() << endl; cout << q.Dequeue() << endl; cout << q.Size() << endl; ==== ====arrow_forwardlogicarrow_forwardQ1: For the Figure Below if the input to the first tank is step with magnitude 2 find 1. What type of relation between (tanks 1 and 2) and tank 3 2. Initial real value of H2, if the steady state value is 10 3. Final Value of H3 4. H1 at t=1.5 5. For the system tank1 and tank 2 only which case is applied to them (overdamping, underdamped or critically damping) A₁=1 A₂=1 Tank 1 R₁ = 2 * Tank 2 R₁₂=2 A3=0.5 hy R₁=4 Tank 3arrow_forward
- Please original work Talk about the most common challenges encountered in a data warehouse What are some creative ways to overcome those challenges What is for one real world example where your method would be effective Please cite in text references and add weblinksarrow_forwardWhat is the differences between mobile website navigation and traditional website navigation?arrow_forwardPoint 10:26 Explain P 10:26 10:25arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
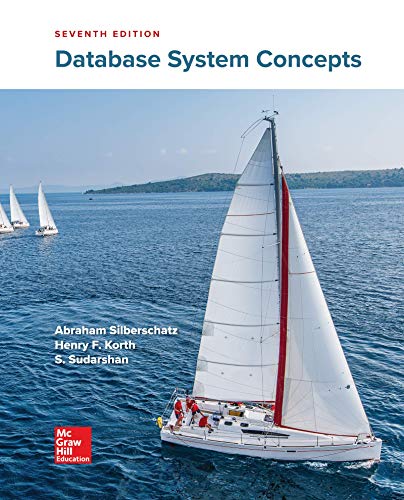
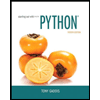
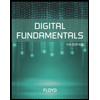
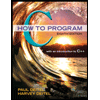
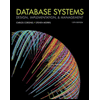
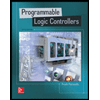