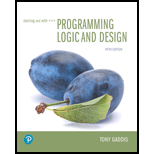
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
5th Edition
ISBN: 9780134801155
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 5SA
Program Plan Intro
Array: Array is a data structure that stores the sequence of similar type of data. It contains the sequence of data with common variable name and similar data type.
Syntax (in C++):
datatypearrayName[size] = {data1, data2,…,dataN};
Here,
datatype – Data type name
arrayName – Name of the array
size – It indicates the size of the array
Two dimensional arrays:
Two dimensional arrays contain multiple sets of data. It contains rows and columns. The syntax for two dimensional array is given below.
Syntax (in C++):
datatypearrayName[ROW_SIZE][COLUMN_SIZE];
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Refer to page 80 for problems on white-box testing.
Instructions:
•
Perform control flow testing for the given program, drawing the control flow graph (CFG).
• Design test cases to achieve statement, branch, and path coverage.
• Justify the adequacy of your test cases using the CFG.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]
Refer to page 10 for problems on parsing.
Instructions:
•
Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)).
• Compute the FIRST and FOLLOW sets and construct the parsing table if applicable.
• Parse a sample input string and explain the derivation step-by-step.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]
Refer to page 20 for problems related to finite automata.
Instructions:
•
Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the
given language.
• Minimize the DFA and show all steps, including state merging.
•
Verify that the automaton accepts the correct language by testing with sample strings.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]
Chapter 8 Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Ch. 8.1 - Prob. 8.1CPCh. 8.1 - Prob. 8.2CPCh. 8.1 - Prob. 8.3CPCh. 8.1 - Prob. 8.4CPCh. 8.1 - Prob. 8.5CPCh. 8.1 - Prob. 8.6CPCh. 8.1 - Prob. 8.7CPCh. 8.1 - What does array bounds checking mean?Ch. 8.1 - Prob. 8.9CPCh. 8.2 - Prob. 8.10CP
Ch. 8.2 - Prob. 8.11CPCh. 8.2 - What does the loop do in the sequential search...Ch. 8.2 - Prob. 8.13CPCh. 8.2 - How do you look for a partial string match when...Ch. 8.3 - Prob. 8.15CPCh. 8.3 - Prob. 8.16CPCh. 8.3 - Describe the algorithm for finding the highest...Ch. 8.3 - Prob. 8.18CPCh. 8.3 - Prob. 8.19CPCh. 8.4 - Prob. 8.20CPCh. 8.4 - Prob. 8.21CPCh. 8.5 - Prob. 8.22CPCh. 8.5 - Write a pseudocode statement that assigns the...Ch. 8.5 - Prob. 8.24CPCh. 8.5 - Prob. 8.25CPCh. 8.6 - Prob. 8.26CPCh. 8 - Prob. 1MCCh. 8 - Prob. 2MCCh. 8 - This is an individual storage location in an...Ch. 8 - Prob. 4MCCh. 8 - Prob. 5MCCh. 8 - Prob. 6MCCh. 8 - Prob. 7MCCh. 8 - Prob. 8MCCh. 8 - Prob. 9MCCh. 8 - Prob. 10MCCh. 8 - Prob. 1TFCh. 8 - Prob. 2TFCh. 8 - Prob. 3TFCh. 8 - Prob. 4TFCh. 8 - Prob. 5TFCh. 8 - Prob. 1SACh. 8 - Prob. 2SACh. 8 - Look at the following pseudocode: Constant Integer...Ch. 8 - Prob. 4SACh. 8 - Prob. 5SACh. 8 - Prob. 1AWCh. 8 - Prob. 2AWCh. 8 - Prob. 3AWCh. 8 - Prob. 4AWCh. 8 - Prob. 5AWCh. 8 - Prob. 6AWCh. 8 - Assume the following declarations appear in a...Ch. 8 - Design an algorithm for a function that accepts an...Ch. 8 - Write a pseudocode algorithm that uses the For...Ch. 8 - Total Sales Design a program that asks the user to...Ch. 8 - Lottery Number Generator Design a program that...Ch. 8 - Prob. 3PECh. 8 - Prob. 4PECh. 8 - Charge Account Validation Design a program that...Ch. 8 - Days of Each Month Design a program that displays...Ch. 8 - Phone Number Lookup Design a program that has two...Ch. 8 - Payroll Design a program that uses the following...Ch. 8 - Drives License Exam The local drivers license...Ch. 8 - Saddle Points Design a program that has a...Ch. 8 - Tic-Tac-Toe Game Design a program that allows two...Ch. 8 - Lo Shu Magic Square The Lo Shu Magic Square is a...
Knowledge Booster
Similar questions
- Refer to page 60 for solving the Knapsack problem using dynamic programming. Instructions: • Implement the dynamic programming approach for the 0/1 Knapsack problem. Clearly define the recurrence relation and show the construction of the DP table. Verify your solution by tracing the selected items for a given weight limit. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 70 for problems related to process synchronization. Instructions: • • Solve a synchronization problem using semaphores or monitors (e.g., Producer-Consumer, Readers-Writers). Write pseudocode for the solution and explain the critical section management. • Ensure the solution avoids deadlock and starvation. Test with an example scenario. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward15 points Save ARS Consider the following scenario in which host 10.0.0.1 is communicating with an external SMTP mail server at IP address 128.119.40.186. NAT translation table WAN side addr LAN side addr (c), 5051 (d), 3031 S: (e),5051 SMTP B D (f.(g) 10.0.0.4 server 138.76.29.7 128.119.40.186 (a) is the source IP address at A, and its value. S: (a),3031 D: (b), 25 10.0.0.1 A 10.0.0.2. 1. 138.76.29.7 10.0.0.3arrow_forward
- 6.3A-3. Multiple Access protocols (3). Consider the figure below, which shows the arrival of 6 messages for transmission at different multiple access wireless nodes at times t=0.1, 1.4, 1.8, 3.2, 3.3, 4.1. Each transmission requires exactly one time unit. 1 t=0.0 2 3 45 t=1.0 t-2.0 t-3.0 6 t=4.0 t-5.0 For the CSMA protocol (without collision detection), indicate which packets are successfully transmitted. You should assume that it takes .2 time units for a signal to propagate from one node to each of the other nodes. You can assume that if a packet experiences a collision or senses the channel busy, then that node will not attempt a retransmission of that packet until sometime after t=5. Hint: consider propagation times carefully here. (Note: You can find more examples of problems similar to this here B.] ☐ U ப 5 - 3 1 4 6 2arrow_forwardJust wanted to know, if you had a scene graph, how do you get multiple components from a specific scene node within a scene graph? Like if I wanted to get a component from wheel from the scene graph, does that require traversing still? Like if a physics component requires a transform component and these two component are part of the same scene node. How does the physics component knows how to get the scene object's transform it is attached to, this being in a scene graph?arrow_forwardHow to develop a C program that receives the message sent by the provided program and displays the name and email included in the message on the screen?Here is the code of the program that sends the message for reference: typedef struct { long tipo; struct { char nome[50]; char email[40]; } dados;} MsgStruct; int main() { int msg_id, status; msg_id = msgget(1000, 0600 | IPC_CREAT); exit_on_error(msg_id, "Creation/Connection"); MsgStruct msg; msg.tipo = 5; strcpy(msg.dados.nome, "Pedro Silva"); strcpy(msg.dados.email, "pedro@sapo.pt"); status = msgsnd(msg_id, &msg, sizeof(msg.dados), 0); exit_on_error(status, "Send"); printf("Message sent!\n");}arrow_forward
- 9. Let L₁=L(ab*aa), L₂=L(a*bba*). Find a regular expression for (L₁ UL2)*L2. 10. Show that the language is not regular. L= {a":n≥1} 11. Show a derivation tree for the string aabbbb with the grammar S→ABλ, A→aB, B→Sb. Give a verbal description of the language generated by this grammar.arrow_forward14. Show that the language L= {wna (w) < Nь (w) < Nc (w)} is not context free.arrow_forward7. What language is accepted by the following generalized transition graph? a+b a+b* a a+b+c a+b 8. Construct a right-linear grammar for the language L ((aaab*ab)*).arrow_forward
- 5. Find an nfa with three states that accepts the language L = {a^ : n≥1} U {b³a* : m≥0, k≥0}. 6. Find a regular expression for L = {vwv: v, wЄ {a, b}*, |v|≤4}.arrow_forward15. The below figure (sequence of moves) shows several stages of the process for a simple initial configuration. 90 a a 90 b a 90 91 b b b b Represent the action of the Turing machine (a) move from one configuration to another, and also (b) represent in the form of arbitrary number of moves.arrow_forward12. Eliminate useless productions from Sa aA BC, AaBλ, B→ Aa, C CCD, D→ ddd Cd. Also, eliminate all unit-productions from the grammar. 13. Construct an npda that accepts the language L = {a"b":n≥0,n‡m}.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
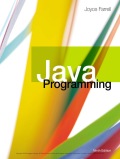
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
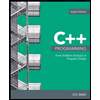
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
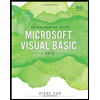
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
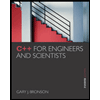
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
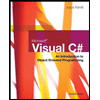
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,