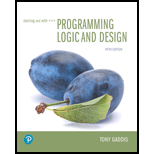
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
5th Edition
ISBN: 9780134801155
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 2AW
Program Plan Intro
Array:
Array is a data structure that stores the sequence of similar type of data. It contains the sequence of data with common variable name and similar data type.
Syntax (in C++):
datatypearrayName[size] = {data1, data2,…,dataN};
Here,
datatype – Data type name
arrayName – Name of the array
size – It indicates the size of the array
- Declare a constant variable.
- Declare an integer array with size “20”.
- Loop from “0” through “19”.
- Print the numbers.
- End “for” loop.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2. Lottery Number Generator
Design a program that generates a 7-digit lottery number. The program should have
an Integer array with 7 elements. Write a loop that steps through the array, ran-
domly generating a number in the range of 0 through 9 for each element. (Use the
random function that was discussed in Chapter 6.) Then write another loop that
displays the contents of the array.
Lottery Number Generator Problem
Use pseudocode to design a program that generates a 7-digit lottery number.
This program should have an integer array with 7 elements.
Then Write a loop that steps through the array, randomly generating a number in the range of 0 through 9 for each element.
Finally, write another loop that displays the contents of the array.
Lottery Number Generator Problem
Design a Flowchart that generates a 7-digit lottery number. The program should have an integer array with 7 elements. Write a loop that steps through the array, randomly generating a number in the range of 0 through 9 for each element. Then, write another loop that displays the contents of the array.
Chapter 8 Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Ch. 8.1 - Prob. 8.1CPCh. 8.1 - Prob. 8.2CPCh. 8.1 - Prob. 8.3CPCh. 8.1 - Prob. 8.4CPCh. 8.1 - Prob. 8.5CPCh. 8.1 - Prob. 8.6CPCh. 8.1 - Prob. 8.7CPCh. 8.1 - What does array bounds checking mean?Ch. 8.1 - Prob. 8.9CPCh. 8.2 - Prob. 8.10CP
Ch. 8.2 - Prob. 8.11CPCh. 8.2 - What does the loop do in the sequential search...Ch. 8.2 - Prob. 8.13CPCh. 8.2 - How do you look for a partial string match when...Ch. 8.3 - Prob. 8.15CPCh. 8.3 - Prob. 8.16CPCh. 8.3 - Describe the algorithm for finding the highest...Ch. 8.3 - Prob. 8.18CPCh. 8.3 - Prob. 8.19CPCh. 8.4 - Prob. 8.20CPCh. 8.4 - Prob. 8.21CPCh. 8.5 - Prob. 8.22CPCh. 8.5 - Write a pseudocode statement that assigns the...Ch. 8.5 - Prob. 8.24CPCh. 8.5 - Prob. 8.25CPCh. 8.6 - Prob. 8.26CPCh. 8 - Prob. 1MCCh. 8 - Prob. 2MCCh. 8 - This is an individual storage location in an...Ch. 8 - Prob. 4MCCh. 8 - Prob. 5MCCh. 8 - Prob. 6MCCh. 8 - Prob. 7MCCh. 8 - Prob. 8MCCh. 8 - Prob. 9MCCh. 8 - Prob. 10MCCh. 8 - Prob. 1TFCh. 8 - Prob. 2TFCh. 8 - Prob. 3TFCh. 8 - Prob. 4TFCh. 8 - Prob. 5TFCh. 8 - Prob. 1SACh. 8 - Prob. 2SACh. 8 - Look at the following pseudocode: Constant Integer...Ch. 8 - Prob. 4SACh. 8 - Prob. 5SACh. 8 - Prob. 1AWCh. 8 - Prob. 2AWCh. 8 - Prob. 3AWCh. 8 - Prob. 4AWCh. 8 - Prob. 5AWCh. 8 - Prob. 6AWCh. 8 - Assume the following declarations appear in a...Ch. 8 - Design an algorithm for a function that accepts an...Ch. 8 - Write a pseudocode algorithm that uses the For...Ch. 8 - Total Sales Design a program that asks the user to...Ch. 8 - Lottery Number Generator Design a program that...Ch. 8 - Prob. 3PECh. 8 - Prob. 4PECh. 8 - Charge Account Validation Design a program that...Ch. 8 - Days of Each Month Design a program that displays...Ch. 8 - Phone Number Lookup Design a program that has two...Ch. 8 - Payroll Design a program that uses the following...Ch. 8 - Drives License Exam The local drivers license...Ch. 8 - Saddle Points Design a program that has a...Ch. 8 - Tic-Tac-Toe Game Design a program that allows two...Ch. 8 - Lo Shu Magic Square The Lo Shu Magic Square is a...
Knowledge Booster
Similar questions
- (Electrical eng.) Write a program that specifies three one-dimensional arrays named current, resistance, and volts. Each array should be capable of holding 10 elements. Using a for loop, input values for the current and resistance arrays. The entries in the volts array should be the product of the corresponding values in the current and resistance arrays (sovolts[i]=current[i]resistance[i]). After all the data has been entered, display the following output, with the appropriate value under each column heading: CurrentResistance Voltsarrow_forward(Numerical) Write and test a function that returns the position of the largest and smallest values in an array of double-precision numbers.arrow_forward(File creation) Write a C++ program that creates an array containing the integer numbers 60, 40, 80, 90, 120, 150, 130, 160, 170, and 200. Your program should then write the data in the array to a text file. (Alternatively, you can create the file with a text editor.)arrow_forward
- A structure that allows repeated execution of a block of statements is a(n) _____________. sequence selection array looparrow_forwardAny loop statement can be used to traverse an array. True or Falsearrow_forwardpseudo code in problem solving and logic and flowchartTotal Sales (Arrays) Design a program that asks the user to enter a store’s sales for each day of the week (for one week). The amounts should be stored in an array. Use a loop to calculate the total sales for the week and display the result. Guideline: Rename the variables with your student number (e.g., amount_697) Use an array to solve the above problem Add comments with your name/student number Comment your codearrow_forward
- comprog true or falsearrow_forwardQuestion Write a statement that declares a string arrays initialized with the following strings: Write a loop that display the content of each element in the array declared in question 1 "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" and "Sunday"arrow_forwardThe code 'd' is used to create a array.arrow_forward
- Comprog Any loop statement can be used to traverse an array.arrow_forwardCh7 - Arrays java In a loop, ask the user to enter 10 integer values and store the values in an array. Pass the array to a method. In the method, use a loop to subtract 5 from each element and return the changed array to the main method.In the main method, use a loop to add the array values and display the result.arrow_forwardDate Printer Write a program that reads a char array from the user containinga date in the form mm/dd/yyyy. It should print the date in the form March 12, 2014.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
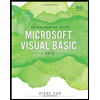
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
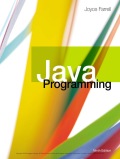
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
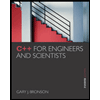
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
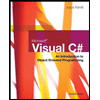
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
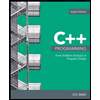
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning