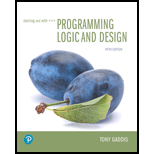
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
5th Edition
ISBN: 9780134801155
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 2SA
Program Plan Intro
Array:
- Array is a data structure that stores the sequence of similar type of data.
- It contains the sequence of data with common variable name and similar data type.
Syntax (in C++):
datatype arrayName[size] = {data1, data2,…,dataN};
Here,
datatype – Data type name
arrayName – Name of the array
size – It indicates the size of the array
Subscripts:
- Each and every element in an array is assigned to a special value called as subscripts or indexes.
- The starting number of subscript or index is “[0]”.
- Indexes are used to find out specific value from the array.
- The first element in an array is assigned to the index value “[0]”.
- The last element in an array is assigned to the index value “[n-1]” where “n” is the number of elements in an array.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Which one of the 4 Entities mention in the diagram can have a recursive relationship?
If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?
Please answer the JAVA OOP Programming Assignment scenario below:
Patriot Ships is a new cruise line company which has a fleet of 10 cruise ships, each with a capacity of 300 passengers. To manage its operations efficiently, the company is looking for a program that can help track its fleet, manage bookings, and calculate revenue for each cruise.
Each cruise is tracked by a Cruise Identifier (must be 5 characters long), cruise route (e.g. Miami to Nassau), and ticket price. The program should also track how many tickets have been sold for each cruise.
Create an object-oriented solution with a menu that allows a user to select one of the following options:
1. Create Cruise – This option allows a user to create a new cruise by entering all necessary details (Cruise ID, route, ticket price). If the maximum number of cruises has already been created, display an error message.
2. Search Cruise – This option allows to search a cruise by the user provided cruise ID.
3. Remove Cruise – This op…
I need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.
Chapter 8 Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Ch. 8.1 - Prob. 8.1CPCh. 8.1 - Prob. 8.2CPCh. 8.1 - Prob. 8.3CPCh. 8.1 - Prob. 8.4CPCh. 8.1 - Prob. 8.5CPCh. 8.1 - Prob. 8.6CPCh. 8.1 - Prob. 8.7CPCh. 8.1 - What does array bounds checking mean?Ch. 8.1 - Prob. 8.9CPCh. 8.2 - Prob. 8.10CP
Ch. 8.2 - Prob. 8.11CPCh. 8.2 - What does the loop do in the sequential search...Ch. 8.2 - Prob. 8.13CPCh. 8.2 - How do you look for a partial string match when...Ch. 8.3 - Prob. 8.15CPCh. 8.3 - Prob. 8.16CPCh. 8.3 - Describe the algorithm for finding the highest...Ch. 8.3 - Prob. 8.18CPCh. 8.3 - Prob. 8.19CPCh. 8.4 - Prob. 8.20CPCh. 8.4 - Prob. 8.21CPCh. 8.5 - Prob. 8.22CPCh. 8.5 - Write a pseudocode statement that assigns the...Ch. 8.5 - Prob. 8.24CPCh. 8.5 - Prob. 8.25CPCh. 8.6 - Prob. 8.26CPCh. 8 - Prob. 1MCCh. 8 - Prob. 2MCCh. 8 - This is an individual storage location in an...Ch. 8 - Prob. 4MCCh. 8 - Prob. 5MCCh. 8 - Prob. 6MCCh. 8 - Prob. 7MCCh. 8 - Prob. 8MCCh. 8 - Prob. 9MCCh. 8 - Prob. 10MCCh. 8 - Prob. 1TFCh. 8 - Prob. 2TFCh. 8 - Prob. 3TFCh. 8 - Prob. 4TFCh. 8 - Prob. 5TFCh. 8 - Prob. 1SACh. 8 - Prob. 2SACh. 8 - Look at the following pseudocode: Constant Integer...Ch. 8 - Prob. 4SACh. 8 - Prob. 5SACh. 8 - Prob. 1AWCh. 8 - Prob. 2AWCh. 8 - Prob. 3AWCh. 8 - Prob. 4AWCh. 8 - Prob. 5AWCh. 8 - Prob. 6AWCh. 8 - Assume the following declarations appear in a...Ch. 8 - Design an algorithm for a function that accepts an...Ch. 8 - Write a pseudocode algorithm that uses the For...Ch. 8 - Total Sales Design a program that asks the user to...Ch. 8 - Lottery Number Generator Design a program that...Ch. 8 - Prob. 3PECh. 8 - Prob. 4PECh. 8 - Charge Account Validation Design a program that...Ch. 8 - Days of Each Month Design a program that displays...Ch. 8 - Phone Number Lookup Design a program that has two...Ch. 8 - Payroll Design a program that uses the following...Ch. 8 - Drives License Exam The local drivers license...Ch. 8 - Saddle Points Design a program that has a...Ch. 8 - Tic-Tac-Toe Game Design a program that allows two...Ch. 8 - Lo Shu Magic Square The Lo Shu Magic Square is a...
Knowledge Booster
Similar questions
- Southern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not). The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15. A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots): The average daily utilization of the aircraft (percentage of total flights that fly) The…arrow_forwardWhy is JAVA OOP is really difficult to study?arrow_forwardMy daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forward
- Q4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forwarda. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 1 1 0 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report.arrow_forwardUsing XML, design a simple user interface for a fictional app. Your UI should include at least three different UI components (e.g., TextView, Button, EditText). Explain the purpose of each component in your design-you need to add screenshots of your work with your name as part of the code to appear on the interface-. Screenshot is needed.arrow_forward
- Q4) A thin ring of radius 5 cm is placed on plane z = 1 cm so that its center is at (0,0,1 cm). If the ring carries 50 mA along a^, find H at (0,0,a).arrow_forward4. [15 pts] A logic function F of four variables a; b; c; d is described by the following K-map. Derive the fully minimized SOP logic expression form of F. cd ab 00 01 11 10 00 0 0 0 1 01 1 0 0 1 11 1 0 1 1 10 0 0 1 1arrow_forward2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisimarrow_forward
- How would I go about creating this computer database in MariaDB with sql? Create a database name "dbXXXXXX" Select the database using the "use [database name]" command. Now you are in the database. Based on the above schema from Enrolment System database, create all the tables with the last 6 digits of "123456", then the table name for table Lecturer should be "123456_Lecturer". Refer to basic SQL lecture note to create table that has primary keys and Foreign Keys. Provide the datatype of each attributes. Add a column called "Department" with datatype "VARCHAR(12)" to the table "Lecturer". Shows the metadata of the updated "Lecturer" table. (Use Describe command) Drop the "Department" column from the table "Lecturer", and show the metadata of the updated "Lecturer" table. Insert three (3) data to each of the table in the tables created. Note: If you have foreign key issues, please disable foreign key constraints before inserting the data, see below SET FOREIGN_KEY_CHECKS=0;…arrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 3. Consider the real number x = (3.395) 10 (a) (b) Convert the decimal number x into binary format up to 7 binary places (7 binary digits after decimal) Convert the calculated value into denormalized form and calculate fl(x) for m=4 Don't use any Al tool show answer in pen a nd paper then take pi ctures and sendarrow_forwardSimplify the following expressions by means of a four-variable K-Map. AD+BD+ BC + ABDarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
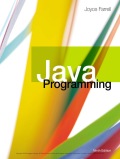
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
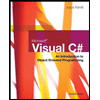
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
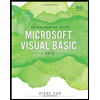
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
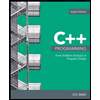
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
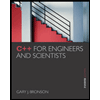
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr