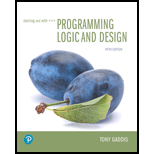
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
5th Edition
ISBN: 9780134801155
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 4AW
Program Plan Intro
Array:
- Array is a data structure that stores the sequence of similar type of data.
- It contains the sequence of data with common variable name and similar data type.
Syntax (in C++):
datatype arrayName[size] = {data1, data2,…,dataN};
Here,
datatype – Data type name
arrayName – Name of the array
size – It indicates the size of the array
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Suppose your computer is responding very slowly to information requests from the Internet. You observe that your network gateway shows high levels of network activity even though you have closed your e-mail client, Web browser, and all other programs that access the Internet.
What types of malwares could cause such symptoms? What steps can you take to check whether malware has gained access to your system? What tools can you use at each step? If you identify malware, what ways might it have entered your system? How can you restore your PC to safe operation, including the special software tools you may use?
R language
Using R language
Chapter 8 Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Ch. 8.1 - Prob. 8.1CPCh. 8.1 - Prob. 8.2CPCh. 8.1 - Prob. 8.3CPCh. 8.1 - Prob. 8.4CPCh. 8.1 - Prob. 8.5CPCh. 8.1 - Prob. 8.6CPCh. 8.1 - Prob. 8.7CPCh. 8.1 - What does array bounds checking mean?Ch. 8.1 - Prob. 8.9CPCh. 8.2 - Prob. 8.10CP
Ch. 8.2 - Prob. 8.11CPCh. 8.2 - What does the loop do in the sequential search...Ch. 8.2 - Prob. 8.13CPCh. 8.2 - How do you look for a partial string match when...Ch. 8.3 - Prob. 8.15CPCh. 8.3 - Prob. 8.16CPCh. 8.3 - Describe the algorithm for finding the highest...Ch. 8.3 - Prob. 8.18CPCh. 8.3 - Prob. 8.19CPCh. 8.4 - Prob. 8.20CPCh. 8.4 - Prob. 8.21CPCh. 8.5 - Prob. 8.22CPCh. 8.5 - Write a pseudocode statement that assigns the...Ch. 8.5 - Prob. 8.24CPCh. 8.5 - Prob. 8.25CPCh. 8.6 - Prob. 8.26CPCh. 8 - Prob. 1MCCh. 8 - Prob. 2MCCh. 8 - This is an individual storage location in an...Ch. 8 - Prob. 4MCCh. 8 - Prob. 5MCCh. 8 - Prob. 6MCCh. 8 - Prob. 7MCCh. 8 - Prob. 8MCCh. 8 - Prob. 9MCCh. 8 - Prob. 10MCCh. 8 - Prob. 1TFCh. 8 - Prob. 2TFCh. 8 - Prob. 3TFCh. 8 - Prob. 4TFCh. 8 - Prob. 5TFCh. 8 - Prob. 1SACh. 8 - Prob. 2SACh. 8 - Look at the following pseudocode: Constant Integer...Ch. 8 - Prob. 4SACh. 8 - Prob. 5SACh. 8 - Prob. 1AWCh. 8 - Prob. 2AWCh. 8 - Prob. 3AWCh. 8 - Prob. 4AWCh. 8 - Prob. 5AWCh. 8 - Prob. 6AWCh. 8 - Assume the following declarations appear in a...Ch. 8 - Design an algorithm for a function that accepts an...Ch. 8 - Write a pseudocode algorithm that uses the For...Ch. 8 - Total Sales Design a program that asks the user to...Ch. 8 - Lottery Number Generator Design a program that...Ch. 8 - Prob. 3PECh. 8 - Prob. 4PECh. 8 - Charge Account Validation Design a program that...Ch. 8 - Days of Each Month Design a program that displays...Ch. 8 - Phone Number Lookup Design a program that has two...Ch. 8 - Payroll Design a program that uses the following...Ch. 8 - Drives License Exam The local drivers license...Ch. 8 - Saddle Points Design a program that has a...Ch. 8 - Tic-Tac-Toe Game Design a program that allows two...Ch. 8 - Lo Shu Magic Square The Lo Shu Magic Square is a...
Knowledge Booster
Similar questions
- Compare the security services provided by a digital signature (DS) with those of a message authentication code (MAC). Assume that Oscar can observe all messages sent between Rina and Naseem. Oscar has no knowledge of any keys but the public one, in the case of DS. State whether DS and MAC protect against each attack and, if they do, how. The value auth(x) is computed with a DS or a MAC algorithm. In each scenario, assume the message M = x#####auth(x). (Message integrity) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message M, and then sends M in cleartext to Naseem. Oscar intercepts the message and replaces “Mark” with “Oscar.” Will Naseem detect this in the case of either DS or MAC? If yes, how will Naseem detect it? If not, why? (Replay) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message…arrow_forwardI need to resolve the following....You are trying to convince your boss that your company needs to invest in a license for MS-Project (project management software from Microsoft) before beginning a systems project. What arguments would you give her?arrow_forwardWhat are the four types of feasibility? what is the issues addressed by each feasibility component.arrow_forward
- I would like to get ab example of a situation where Agile Methods might be preferable versus the traditional SDLC? What are the characteristics of this situation that give Agile Methods an advantage?arrow_forwardWhat is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).arrow_forwardWhat are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?arrow_forward
- 3. Problem Description: Define the Circle2D class that contains: Two double data fields named x and y that specify the center of the circle with get methods. • A data field radius with a get method. • A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius. • A constructor that creates a circle with the specified x, y, and radius. • A method getArea() that returns the area of the circle. • A method getPerimeter() that returns the perimeter of the circle. • • • A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See Figure (a). A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See Figure (b). A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this circle. See the figure below. р O со (a) (b) (c)< Figure (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another…arrow_forward1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forwardSecurity in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forward
- Please include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forwardObject-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
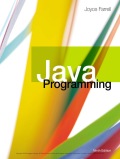
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
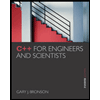
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
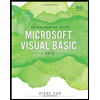
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
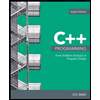
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
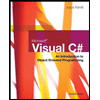
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,