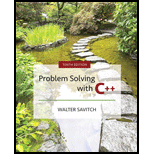
Write a function that determines if two strings are anagrams. The function should not be case sensitive and should disregard any punctuation or spaces. Two strings are anagrams if the letters can be rearranged to form each other. For example, “Eleven plus two” is an anagram of “Twelve plus one.” Each string contains one “v”, three “e’s”, two “l’s”, etc. Test your function with several strings that are anagrams and non-anagrams. You may use either the string class or a C-style string.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Starting Out with Python (4th Edition)
SURVEY OF OPERATING SYSTEMS
Degarmo's Materials And Processes In Manufacturing
Starting Out With Visual Basic (8th Edition)
- Modern life has been impacted immensely by computers. Computers have penetrated every aspect of oursociety, either for better or for worse. From supermarket scanners calculating our shopping transactionswhile keeping store inventory; robots that handle highly specialized tasks or even simple human tasks,computers do much more than just computing. But where did all this technology come from and whereis it heading? Does the future look promising or should we worry about computers taking over theworld? Or are they just a necessary evil? Provide three references with your answer.arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forwardYou are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forward
- (A) A cellular system has 12 microcells with 10 channels per cell. The microcells are split into 3 microcells, and each microcell is further split into 4 picocells. Determine the number of channels available in system after splitting into picocelles.arrow_forwardQuestion 8 (10 points) Produce a relational schema diagram that corresponds to the following ER diagram for a Vacation Property Rentals database. Your relational schema diagram should include primary & foreign keys. Upload your relational schema diagram as a PDF document. Don't forget that the relation schemas for "Beach Property" and "Mountain Property" should each have primary keys. FYI: "d" in this notation denotes a subclass. Figure 2: ER Diagram for Question 8 id first RENTER name middle last address phone email 1 signs N id begin date RENTAL AGREEMENT end date amount N street address books city id 1 state address num. rooms PROPERTY zip code base rate type propertyType blocks to beach activity "B" "M" BEACH PROPERTY MOUNTAIN PROPERTYarrow_forwardNotes: 1) Answer All Question, 2) 25 points for each question QI Figurel shows the creation of the Frequency Reuse Pattern Using the Cluster Size K: (A) illustrates how i and j can be used to locate a co-channel cell. huster 3 Cluster Cluster 2 X=7(i=2,j1)arrow_forward
- You are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following taking the database offline is not allowed since people are connected to it and how personal data might be bridged and not secured.Provide three references with you answer.arrow_forwardYou are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forwardYou are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answer from websitesarrow_forward
- Modern life has been impacted immensely by computers. Computers have penetrated every aspect of oursociety, either for better or for worse. From supermarket scanners calculating our shopping transactionswhile keeping store inventory; robots that handle highly specialized tasks or even simple human tasks,computers do much more than just computing. But where did all this technology come from and whereis it heading? Does the future look promising or should we worry about computers taking over theworld? Or are they just a necessary evil? Provide three references with your answer.arrow_forwardObjective: 1. Implement a custom Vector class in C++ that manages dynamic memory efficiently. 2. Demonstrate an understanding of the Big Five by managing deep copies, move semantics, and resource cleanup. 3. Explore the performance trade-offs between heap and stack allocation. Task Description: Part 1: Custom Vector Implementation 1. Create a Vector class that manages a dynamically allocated array. 。 Member Variables: ° T✶ data; // Dynamically allocated array for storage. std::size_t size; // Number of elements currently in the vector. std::size_t capacity; // Maximum number of elements before reallocation is required. 2. Implement the following core member functions: Default Constructor: Initialize an empty vector with no allocated storage. 。 Destructor: Free any dynamically allocated memory. 。 Copy Constructor: Perform a deep copy of the data array. 。 Copy Assignment Operator: Free existing resources and perform a deep copy. Move Constructor: Transfer ownership of the data array…arrow_forward2.68♦♦ Write code for a function with the following prototype: * Mask with least signficant n bits set to 1 * Examples: n = 6 -> 0x3F, n = 17-> 0x1FFFF * Assume 1 <= n <= w int lower_one_mask (int n); Your function should follow the bit-level integer coding rules Be careful of the case n = W.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
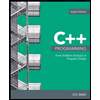
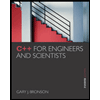