Part 2: Heap vs. Stack Allocation Trade-offs 1. Provide two modes for the Vector: 。 Heap Mode: Default mode where the data array is allocated on the heap. Stack Mode: If the Vector size is small (e.g., fewer than 10 elements), use a fixed-size array allocated on the stack instead of the heap. Use conditional compilation or a template-based design for this. Compare the performance of both modes in terms of memory access speed and overhead. Part 3: Testing and Benchmarks 1. Write a test suite that: 。 Initializes vectors with various sizes. 。 Demonstrates the use of copy and move constructors in operations like Vector v = anotherVector;. о Times operations like push_back and resize for both heap and stack modes. 。 Tests edge cases, e.g., exceeding stack allocation limits or repeatedly resizing. 2. Benchmark and analyze: 。 Heap vs. stack performance: Measure how each mode affects allocation and deallocation times. 。The cost of unnecessary copying if move semantics are not used. Bonus Challenges: 1. Short String Optimization (SSO): Implement a small buffer optimization similar to how std::string works, storing a small number of elements directly within the Vector object to reduce heap allocations. 2. Iterator Support: Add support for iterators so the custom Vector can be used in range-based for loops. 3. Exception Safety: Ensure strong exception safety during reallocation (use the copy- and-swap idiom for the copy assignment operator). Deliverables: Source Code: Well-documented Vector class with all requested features. Test Cases: Demonstrations of the Big Five in action and performance comparisons. Report: A short analysis of the heap vs. stack trade-offs observed during the benchmarks. Objective: 1. Implement a custom Vector class in C++ that manages dynamic memory efficiently. 2. Demonstrate an understanding of the Big Five by managing deep copies, move semantics, and resource cleanup. 3. Explore the performance trade-offs between heap and stack allocation. Task Description: Part 1: Custom Vector Implementation 1. Create a Vector class that manages a dynamically allocated array. 。 Member Variables: ° T✶ data; // Dynamically allocated array for storage. std::size_t size; // Number of elements currently in the vector. std::size_t capacity; // Maximum number of elements before reallocation is required. 2. Implement the following core member functions: Default Constructor: Initialize an empty vector with no allocated storage. 。 Destructor: Free any dynamically allocated memory. 。 Copy Constructor: Perform a deep copy of the data array. 。 Copy Assignment Operator: Free existing resources and perform a deep copy. Move Constructor: Transfer ownership of the data array without copying. 。 Move Assignment Operator: Release existing resources and take ownership. A push_back method to add elements dynamically, resizing the array if needed. 。 operator[]: Provide access to elements by index with bounds checking for safety. 。size Method: Return the current number of elements in the vector. Method signatures: // Default Constructor Vector(); // Destructor ~Vector (); // Copy Constructor Vector (const Vector& other); // Copy Assignment Operator Vector & operator (const Vectors other); // Move Constructor Vector (Vector&& other) noexcept; // Move Assignment Operator Vector & operator= (Vector&& other) noexcept; // Push an element to the vector void push_back(const T& value); // Access elements by index (non-const) T& operator [] (std::size_t index); // Access elements by index (const) const T& operator[] (std::size_t index) const; // Get the current size of the vector std::size_t size() const; // Optional: Get the capacity of the vector std::size_t capacity() const; 3. Efficiency Requirements: Use exponential growth for capacity (e.g., double the capacity when resizing). Use a strategy that minimizes the number of heap allocations.
Part 2: Heap vs. Stack Allocation Trade-offs 1. Provide two modes for the Vector: 。 Heap Mode: Default mode where the data array is allocated on the heap. Stack Mode: If the Vector size is small (e.g., fewer than 10 elements), use a fixed-size array allocated on the stack instead of the heap. Use conditional compilation or a template-based design for this. Compare the performance of both modes in terms of memory access speed and overhead. Part 3: Testing and Benchmarks 1. Write a test suite that: 。 Initializes vectors with various sizes. 。 Demonstrates the use of copy and move constructors in operations like Vector v = anotherVector;. о Times operations like push_back and resize for both heap and stack modes. 。 Tests edge cases, e.g., exceeding stack allocation limits or repeatedly resizing. 2. Benchmark and analyze: 。 Heap vs. stack performance: Measure how each mode affects allocation and deallocation times. 。The cost of unnecessary copying if move semantics are not used. Bonus Challenges: 1. Short String Optimization (SSO): Implement a small buffer optimization similar to how std::string works, storing a small number of elements directly within the Vector object to reduce heap allocations. 2. Iterator Support: Add support for iterators so the custom Vector can be used in range-based for loops. 3. Exception Safety: Ensure strong exception safety during reallocation (use the copy- and-swap idiom for the copy assignment operator). Deliverables: Source Code: Well-documented Vector class with all requested features. Test Cases: Demonstrations of the Big Five in action and performance comparisons. Report: A short analysis of the heap vs. stack trade-offs observed during the benchmarks. Objective: 1. Implement a custom Vector class in C++ that manages dynamic memory efficiently. 2. Demonstrate an understanding of the Big Five by managing deep copies, move semantics, and resource cleanup. 3. Explore the performance trade-offs between heap and stack allocation. Task Description: Part 1: Custom Vector Implementation 1. Create a Vector class that manages a dynamically allocated array. 。 Member Variables: ° T✶ data; // Dynamically allocated array for storage. std::size_t size; // Number of elements currently in the vector. std::size_t capacity; // Maximum number of elements before reallocation is required. 2. Implement the following core member functions: Default Constructor: Initialize an empty vector with no allocated storage. 。 Destructor: Free any dynamically allocated memory. 。 Copy Constructor: Perform a deep copy of the data array. 。 Copy Assignment Operator: Free existing resources and perform a deep copy. Move Constructor: Transfer ownership of the data array without copying. 。 Move Assignment Operator: Release existing resources and take ownership. A push_back method to add elements dynamically, resizing the array if needed. 。 operator[]: Provide access to elements by index with bounds checking for safety. 。size Method: Return the current number of elements in the vector. Method signatures: // Default Constructor Vector(); // Destructor ~Vector (); // Copy Constructor Vector (const Vector& other); // Copy Assignment Operator Vector & operator (const Vectors other); // Move Constructor Vector (Vector&& other) noexcept; // Move Assignment Operator Vector & operator= (Vector&& other) noexcept; // Push an element to the vector void push_back(const T& value); // Access elements by index (non-const) T& operator [] (std::size_t index); // Access elements by index (const) const T& operator[] (std::size_t index) const; // Get the current size of the vector std::size_t size() const; // Optional: Get the capacity of the vector std::size_t capacity() const; 3. Efficiency Requirements: Use exponential growth for capacity (e.g., double the capacity when resizing). Use a strategy that minimizes the number of heap allocations.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 18PE
Related questions
Question

Transcribed Image Text:Part 2: Heap vs. Stack Allocation Trade-offs
1. Provide two modes for the Vector:
。 Heap Mode: Default mode where the data array is allocated on the heap.
Stack Mode: If the Vector size is small (e.g., fewer than 10 elements), use a
fixed-size array allocated on the stack instead of the heap. Use conditional
compilation or a template-based design for this.
Compare the performance of both modes in terms of memory access
speed and overhead.
Part 3: Testing and Benchmarks
1. Write a test suite that:
。 Initializes vectors with various sizes.
。 Demonstrates the use of copy and move constructors in operations like
Vector v = anotherVector;.
о
Times operations like push_back and resize for both heap and stack modes.
。 Tests edge cases, e.g., exceeding stack allocation limits or repeatedly
resizing.
2. Benchmark and analyze:
。 Heap vs. stack performance: Measure how each mode affects allocation
and deallocation times.
。The cost of unnecessary copying if move semantics are not used.
Bonus Challenges:
1. Short String Optimization (SSO): Implement a small buffer optimization similar to
how std::string works, storing a small number of elements directly within the Vector
object to reduce heap allocations.
2. Iterator Support: Add support for iterators so the custom Vector can be used in
range-based for loops.
3. Exception Safety: Ensure strong exception safety during reallocation (use the copy-
and-swap idiom for the copy assignment operator).
Deliverables:
Source Code: Well-documented Vector class with all requested features.
Test Cases: Demonstrations of the Big Five in action and performance
comparisons.
Report: A short analysis of the heap vs. stack trade-offs observed during the
benchmarks.
![Objective:
1. Implement a custom Vector class in C++ that manages dynamic memory efficiently.
2. Demonstrate an understanding of the Big Five by managing deep copies, move
semantics, and resource cleanup.
3. Explore the performance trade-offs between heap and stack allocation.
Task Description:
Part 1: Custom Vector Implementation
1. Create a Vector class that manages a dynamically allocated array.
。 Member Variables:
°
T✶ data; // Dynamically allocated array for
storage.
std::size_t size; // Number of elements currently
in the vector.
std::size_t capacity; // Maximum number of
elements before reallocation is required.
2. Implement the following core member functions:
Default Constructor: Initialize an empty vector with no allocated storage.
。 Destructor: Free any dynamically allocated memory.
。 Copy Constructor: Perform a deep copy of the data array.
。 Copy Assignment Operator: Free existing resources and perform a deep
copy.
Move Constructor: Transfer ownership of the data array without copying.
。 Move Assignment Operator: Release existing resources and take
ownership.
A push_back method to add elements dynamically, resizing the array if
needed.
。 operator[]: Provide access to elements by index with bounds checking for
safety.
。size Method: Return the current number of elements in the vector.
Method signatures:
// Default Constructor
Vector();
// Destructor
~Vector ();
// Copy Constructor
Vector (const Vector& other);
// Copy Assignment Operator
Vector & operator (const Vectors other);
// Move Constructor
Vector (Vector&& other) noexcept;
// Move Assignment Operator
Vector & operator= (Vector&& other) noexcept;
// Push an element to the vector
void push_back(const T& value);
// Access elements by index (non-const)
T& operator [] (std::size_t index);
// Access elements by index (const)
const T& operator[] (std::size_t index) const;
// Get the current size of the vector
std::size_t size() const;
// Optional: Get the capacity of the vector
std::size_t capacity() const;
3. Efficiency Requirements:
Use exponential growth for capacity (e.g., double the capacity when
resizing).
Use a strategy that minimizes the number of heap allocations.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F802524ce-0929-4bf2-904f-06915ae04c1f%2F372f6b07-ef24-4325-84a6-d6ba0bb4a7b8%2Fmgmjrx3_processed.png&w=3840&q=75)
Transcribed Image Text:Objective:
1. Implement a custom Vector class in C++ that manages dynamic memory efficiently.
2. Demonstrate an understanding of the Big Five by managing deep copies, move
semantics, and resource cleanup.
3. Explore the performance trade-offs between heap and stack allocation.
Task Description:
Part 1: Custom Vector Implementation
1. Create a Vector class that manages a dynamically allocated array.
。 Member Variables:
°
T✶ data; // Dynamically allocated array for
storage.
std::size_t size; // Number of elements currently
in the vector.
std::size_t capacity; // Maximum number of
elements before reallocation is required.
2. Implement the following core member functions:
Default Constructor: Initialize an empty vector with no allocated storage.
。 Destructor: Free any dynamically allocated memory.
。 Copy Constructor: Perform a deep copy of the data array.
。 Copy Assignment Operator: Free existing resources and perform a deep
copy.
Move Constructor: Transfer ownership of the data array without copying.
。 Move Assignment Operator: Release existing resources and take
ownership.
A push_back method to add elements dynamically, resizing the array if
needed.
。 operator[]: Provide access to elements by index with bounds checking for
safety.
。size Method: Return the current number of elements in the vector.
Method signatures:
// Default Constructor
Vector();
// Destructor
~Vector ();
// Copy Constructor
Vector (const Vector& other);
// Copy Assignment Operator
Vector & operator (const Vectors other);
// Move Constructor
Vector (Vector&& other) noexcept;
// Move Assignment Operator
Vector & operator= (Vector&& other) noexcept;
// Push an element to the vector
void push_back(const T& value);
// Access elements by index (non-const)
T& operator [] (std::size_t index);
// Access elements by index (const)
const T& operator[] (std::size_t index) const;
// Get the current size of the vector
std::size_t size() const;
// Optional: Get the capacity of the vector
std::size_t capacity() const;
3. Efficiency Requirements:
Use exponential growth for capacity (e.g., double the capacity when
resizing).
Use a strategy that minimizes the number of heap allocations.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
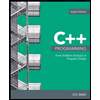
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
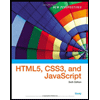
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
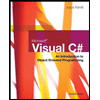
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
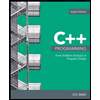
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
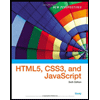
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
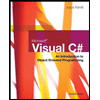
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
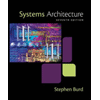
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
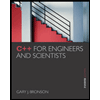
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage