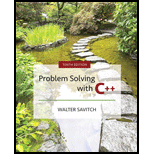
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 2P
Program Plan Intro
- Include the necessary header files.
- Declare the namespace.
- Define the “main()” function.
- Declare the necessary variables.
- Initialize the string.
- Use the functions “substr()” to return the newly constructed string.
- Use the function “find()” to find the space
- Print the result.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Create a function that accepts a string (of a person's first and last name) and returns a string with the first and last name swapped.
Examples
NameShuffle("Donald Trump") - "Trump Donald"
NameShuffle ("Rosie O'Donnell") - "O'Donnell Rosie"
NameShuffle ("Seymour Butts") - "Butts Seymour"
• There will be exactly one space between the first and last name.
In c#
Use C++ language Avoid cryptic variable names and poor indentations.
In this assignment you will be responsible for writing several string validation and manipulationfunctions. The assumption will be that you are writing functions that will take input from a form andmanipulate or validate the information entered before it is processed into a database. A database is acollection of information but the information in the database must always be entered in a specificformat. Your responsibility will be to take data entered into a program and be sure it is writtencorrectly before it is entered into the database.Each function will have to have a specific name and heading. Each function will also need to includea docstring, the correct logic, and the proper return. Each function will be given a specific set ofpreconditions and postconditions/returns. A precondition is something you may assume to be truewhen the function is executed. A post condition is something you need to be sure is completed whenthe function is executed. Be sure to test each one of your…
Chapter 8 Solutions
Problem Solving with C++ (10th Edition)
Ch. 8.1 - Prob. 1STECh. 8.1 - What C string will be stored in singingString...Ch. 8.1 - What (if anything) is wrong with the following...Ch. 8.1 - Suppose the function strlen (which returns the...Ch. 8.1 - Prob. 5STECh. 8.1 - How many characters are in each of the following...Ch. 8.1 - Prob. 7STECh. 8.1 - Given the following declaration and initialization...Ch. 8.1 - Given the declaration of a C-string variable,...Ch. 8.1 - Write code using a library function to copy the...
Ch. 8.1 - What string will be output when this code is run?...Ch. 8.1 - Prob. 12STECh. 8.1 - Consider the following code (and assume it is...Ch. 8.1 - Consider the following code (and assume it is...Ch. 8.2 - Consider the following code (and assume that it is...Ch. 8.2 - Prob. 16STECh. 8.2 - Consider the following code: string s1, s2...Ch. 8.2 - What is the output produced by the following code?...Ch. 8.3 - Is the following program legal? If so, what is the...Ch. 8.3 - What is the difference between the size and the...Ch. 8 - Create a C-string variable that contains a name,...Ch. 8 - Prob. 2PCh. 8 - Write a program that inputs a first and last name,...Ch. 8 - Write a function named firstLast2 that takes as...Ch. 8 - Write a function named swapFrontBack that takes as...Ch. 8 - Prob. 6PCh. 8 - Write a program that inputs two string variables,...Ch. 8 - Solution to Programming Project 8.1 Write a...Ch. 8 - Write a program that will read in a line of text...Ch. 8 - Give the function definition for the function with...Ch. 8 - Write a program that reads a persons name in the...Ch. 8 - Write a program that reads in a line of text and...Ch. 8 - Write a program that reads in a line of text and...Ch. 8 - Write a program that can be used to train the user...Ch. 8 - Write a sorting function that is similar to...Ch. 8 - Redo Programming Project 6 from Chapter 7, but...Ch. 8 - Redo Programming Project 5 from Chapter 7, but...Ch. 8 - Prob. 11PPCh. 8 - Write a program that inputs a time from the...Ch. 8 - Solution to Programming Project 8.14 Given the...Ch. 8 - Write a function that determines if two strings...Ch. 8 - Write a program that inputs two strings (either...Ch. 8 - Write a program that manages a list of up to 10...
Knowledge Booster
Similar questions
- About beginner python programming: - Make a function in Python called SIGA, which receives a tuple containing 4 pieces of information: the nameof the student and his three respective grades. Your function should return a tuple, whose first element is the student's name,the second element is the grades average, and the third element is the student's situation, which must be representedby a string. If the average of the three grades of the student is greater than or equal to 7 (inclusive), the function should bereturn: (<name>, <average>,’approved’, ’Congratulations!́). If the student's average grades is less than 7 but greater than or equal to 5, your function should return: (<name>, <average>,’approved’). If the average is smallerthan 5, the function should return (<name>, <media>,’failed’). This function should return the averagewith one decimal place only.arrow_forwardWrite a program that reads in a line consisting of a students name, Social Security number, user ID, and password. The program outputs the string in which all the digits of the Social Security number and all the characters in the password are replaced by x. (The Social Security number is in the form 000-00-0000, and the user ID and the password do not contain any spaces.) Your program should not use the operator [] to access a string element. Use the appropriate functions described in Table 7-1.arrow_forwardCode using c++ 3. From Person to People by CodeChum Admin Now that we have created a Person, it's time to create more Person and this tech universe shall be filled with people! Instructions: In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age and character value for its gender. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. In the main() function, there's a pre-created array of 5 Persons. Your task is to ask the user for the values of the age and gender of these Persons. Then, once you've set their ages and genders, call the displayPerson() function and pass them one by one. Input 1. A series of ages and genders of the 5 Persons Output Person·#1 Enter·Person's·age:·24 Enter·Person's·gender:·M Person·#2 Enter·Person's·age:·21 Enter·Person's·gender:·F Person·#3 Enter·Person's·age:·22 Enter·Person's·gender:·F Person·#4…arrow_forward
- Q3 Write a program in C ++, with a row in the name (Shape), to calculate the total area of the figure below. For each geometry, use a function to calculate its participation area, noting that the area of the triangle = 1/2 (base * height)arrow_forwardC++ Languagearrow_forwardB4- Declare a string variable in Python with any three words as its value. Describe three functions to change the case of the words stored in the variable with examples.arrow_forward
- Task description: Use regular expression to implement a function which accepts a passed string and check if it is a valid email address. A program accepts user's input and calls the email validation function. If the email is valid, print out the email, username and host. (Rule: word characters (a-zA-Z0-9_ or "\w"), dot, and hyphen are considered as valid characters in an email address, besides of one & only one "@'.) (Sample output as shown in the following figure is for demonstration purposes only.) In [24]: runfile('C:/tmp/units/2020/SIT384-2020-1/portfolio/week2/ Task2.3P.py', wdir='C:/tmp/units/2020/SIT384-2020-1/portfolio/week2') Please input your email address:teste Not a valid email. Please input your email address:shang.gao@test2.server.com email: shang.gao@test2.server.com, username: shang.gao , host: test2.server.com Submission: Submit the following files to OnTrack: 1. Your program source code (e.g. task2-3.py) 2. A screen shot of your program running Check the following…arrow_forwardCreate a function that removes the first and last characters from a string. In c# Examples RemoveFirstLast("hello") - "ell" RemoveFirstLast ("maybe") - "ayb" RemoveFirstLast("benefit") - "enefi" RemoveFirstLast("a") - "a" Note If the string is 2 or fewer characters long, return the string itself (See example # 4).arrow_forwardC++. Strings and extended characters. String handling is standard functions - connecting lines, comparing, searching for characters, parts of lines search, change and delete. Task : Create a program that converts the given number in the binary system to the decimal system . A binary number is given as a string, and the result is a numeric value.arrow_forward
- Create a function called reverse() that has a string parameter. The function reverses the characters of the string locally. ( in C language)arrow_forwardIn C programming language. Objectives:• Use of functions and passing by value and reference.• Use of selection constructs.• Use of repetition constructs (loops).• The use of the random number generator and seeding.• Display formatted output using input/outputstatements.• Use format control strings to format text output. Description: For this project you are tasked with building a user application that will select sets of random numbers. Your application must use functions and pass values and pointers. Your program will pick sets of 6 random numbers with values of 1 to 53. The user should be able to choose how many sets to produce. The challenge will be to ensure that no number in a set of 6 numbers is a repeat. The number can be in another set just not a duplicate in the same set of 6. Your program should prompt the user and ask them how many number sets they wish to have generated. The program should not exit unless the user enters a value indicating they wish to quit. Minimum…arrow_forwardC++ program Create program and prompt me to enter a dollar amount between 0 and 999.99999 as a C-string or X to exit. Loop unit I enter X to exit. Use a C-string for the input amount and related method processing. Review section 10.4. This implies you will have the following: #include <cstring> #include <string> may be needed in Visual Studio, but still use C-String functions. Create a method that will use the functions described in Chapter 10. It is OK to use a globally defined char array. You must call this method to produce the formatted results. The program must modify or build a C-String in this format. Display the C-String created. 1. Include leading $ sign. 2. Must have a single decimal point with 2 digits after the decimal. If the amount had extra digits, then the result is a truncated value. 100.99 = $100.99 150.00999 = $150.0050 = $50.0048.1 = $48.10This program is not simple. This exercise will help you understand some of the limitations of…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
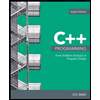
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning