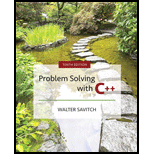
Concept explainers
Write a sorting function that is similar to Display 7.12 in Chapter 7 except that it has an argument for a

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (3rd Edition)
Introduction To Programming Using Visual Basic (11th Edition)
C Programming Language
Starting out with Visual C# (4th Edition)
Modern Database Management
Starting Out with Java: From Control Structures through Objects (6th Edition)
- write in html and scriptarrow_forwardJavaScript Q3. Create a function find(arr,value) which finds a value in the given array and returns its index, or-1 if not found. The given array is: arra[10,3,20,4)arrow_forwardNumPy includes a submodule numpy.linalg where many functions are defined to allow the user to do various things related to linear algebra. If A is a square matrix represented as an object of type ndarray , the function eigvals () will return the eigenvalues of A as an array. Which of the following code snippets would correctly import the module and call this function with the matrix (A as input? Note: There may be more than one correct answer! Select all that will work.arrow_forward
- Write a function numberOfOccurences() which takes an integer reference array of size 10 and a search value as parameters and returns the number of occurrences of the value inside the array. Test your function. Initialize an array that contains the following numbers: 4, 7, 2, 8, 8, 1, 4, 8, 9, 1. Then test it with search values 1, 8 and 6. Your program output should look like the following: [4 7 2 8 8 1 4 8 9 1 ]1 occurs 2 times in the array.8 occurs 3 times in the array.6 occurs 0 times in the array. C++ Nothing too advanced pleasearrow_forwardModify from the code below, add an additional input from the use input to get how many random integers to create. Use a vector<int> instead of an int array to store the random integers. Function prototypes: void showValues(const vector<int>&); void fillVector(vector<int>&, int, int); int maximum(const vector<int>&); int minimum(const vector<int>&); double average(const vector<int>&); bool searchValue(const vector<int>&, int); Code: #include <iostream>#include <iomanip>#include <cstdlib>#include <ctime>using namespace std; // Function prototypevoid showValues(const int[], int); void fillArray(int[], int, int, int); int maximum(const int[], int); int minimum(const int[], int); double average(const int[], int); int main(){const int ARRAY_SIZE = 10;int numbers[ARRAY_SIZE];srand(time(0));int low = 0, high = 1;cout << "**This program will generate 10 random integers between [low] and…arrow_forwardYou are working for a university to maintain a list of grades and some related statistics for a student. Class and Data members: Create a class called Student that stores a student’s grades (integers) in a vector (do not use an array). The class should have data members that store a student’s name and the course for which the grades are earned. Constructor(s): The class should have a 2-argument constructor that receives the student’s name and course as parameters and sets the appropriate data members to these values. Member Functions: The class should have functions as follows: Member functions to set and get the student’s name and course variables. A member function that adds a single grade to the vector. Only positive grades are allowed. Call this function AddGrade. A member function to sort the vector in ascending order. A member function to compute the average (x̄) of the grades in the vector. The formula for calculating an average is x̄ = ∑xi / n where xi is the value of each…arrow_forward
- You are working for a university to maintain a list of grades and some related statistics for a student. Class and Data members: Create a class called Student that stores a student’s grades (integers) in a vector (do not use an array). The class should have data members that store a student’s name and course for which the grades are earned. Constructor(s): The class should have a 2-argument constructor that receives the student’s name and course as parameters and sets the appropriate data members to these values. Member Functions: The class should have functions as follows: Member functions to set and get the student’s name and course variables. A member function that adds a single grade to the vector. Only positive grades are allowed. Call this function AddGrade A member function to sort the vector in ascending order. A member function to compute the average (x̄) of the grades in the vector. The formula for calculating an average is x̄ = ∑xi / n where xi is the value of each…arrow_forwardCreate a function that returns the frequency distribution of an array. This function should return an object, where the keys are the unique elements and the values are the frequency in which those elements occur. Examples get Frequencies (["A", "B", "A", "A", "A"]) → { A: 4, B: 1 } get Frequencies ([1, 2, 3, 3, 2]) → { "1": 1, "2": 2, "3": 2 } get Frequencies ([true, false, true, false, false]) { true: 2, fal →arrow_forwardUsing C++ Implement the ordered version of removeElement in the window below. Your function should validate inputs and remove the element in the given position by moving all of the other elements down. // removeElement preserves the order of the remaining elements in the array.// @param: char array that is ordered in some way.// @param: int numElems is the number of elements// @param: int position of the element being removed// @returns true if the element is removed, false otherwise.// be careful to use reference parameters where necessary!arrow_forward
- in c++arrow_forwardGetting the Concatenated Number In the code editor, you will see that there's a globally declared integer array named, dataset. Additionally, there's a function declaration for the function getConcatenatedNumber(int n). Your task is to define the getConcatenatedNumber function. This will accept an integer n and will perform some operations on the global array. If the passed integer n is an even number, you need to concatenate all the integers located in the even indices (special case: 0 index is considered as even here). If the passed integer n is an odd number, you need to concatenate all integers located in the odd indices. Since 0 is neither odd or even, you need to concatenate all the integers in the array if n is 0. Afterwards, you need to add the inputted integer n three times to the concatenated number and return the result. In the main(), ask the user to input the value of n, call the function getConcatenatedNumber and pass the value of n, and then print the result. SAMPLE:…arrow_forwardWrite a maxConsecutiveDiff function which returns the maximum difference between any two consecutive values in the array.Consecutive values are next to each other in an array (+/- 1 index)This function should accept all required data as parameters.ExamplesIf the array is 17 15 16 11 14 the maximum consecutive difference is 5 which is the difference between 16 and 11.If the array is 10 13 11 12 19 the maximum consecutive difference is 7 which is the difference between 12 and 19.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
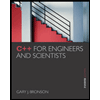
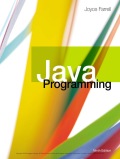