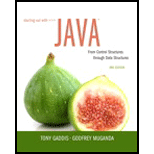
Payroll Class
Write a Payroll class that uses the following arrays as fields:
- employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489
- hours. An array of seven integers to hold the number of hours worked by each employee
- payRate. An array of seven doubles to hold each employee’s hourly pay rate
- wages. An array of seven doubles to hold each employee’s gross wages
The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array.
In addition to the appropriate accessor and mutator methods, the class should have a method that accepts an employee’s identification number as an argument and returns the gross pay for that employee.
Demonstrate the class in a complete program that displays each employee number and asks the user to enter that employee’s hours and pay rate. It should then display each employee’s identification number and gross wages.
Input Validation; Do not accept negative values for hours or numbers less than 6.00 for pay rate.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (8th Edition)
Concepts of Programming Languages (11th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Digital Fundamentals (11th Edition)
Starting Out with Python (3rd Edition)
Starting Out with C++: Early Objects
- Rainfall Type Create a RainFall class that keeps the total rainfall in an array of doubles for each of the 12 months. Methods in the programme should return the following values: • the total annual rainfall; • the average monthly rainfall • the wettest month of the year • the month with the least amount of rain Demonstrate the class in its entirety. Validation of Input: Accepting negative amounts for monthly rainfall data is not permitted.arrow_forwardWrite code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods: set: This method receives the array of person class and set the attribute. displayAll: This method will display values of all persons. sort: This method will sort the array according to age values of the persons with ascending order using Selection Sort. reverse: This method will reverse the order of the array. getPersons: This method will return the array of the persons (attribute). displayEvenAgePerson: This method will display all those persons values who have even age. displayOddAgePerson: This method will display all those persons values who have odd age. displayPrimeAgePerson: This method will display only those persons values who have prime number age. Following is the class of Person: public class Person { private String Name; public int age; public void set(String Name, int age) { this.Name =…arrow_forwardWrite code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods: set: This method receives the array of person class and set the attribute. displayAll: This method will display values of all persons. sort: This method will sort the array according to age values of the persons with ascending order using Selection Sort. reverse: This method will reverse the order of the array. getPersons: This method will return the array of the persons (attribute). displayEvenAgePerson: This method will display all those persons values who have even age. displayOddAgePerson: This method will display all those persons values who have odd age. displayPrimeAgePerson: This method will display only those persons values who have prime number age.arrow_forward
- Lotto Program C++Write a program that simulates the Powerball lottery. In Powerball, a ticket is comprised of 5 numbers between 1 and 69 that must be unique, and a Powerball number between 1 and 26. The Powerball does not have to be unique. Hint: You can use an array to represent the 5 unique numbers between 1 and 69, and an integer variable to represent the powerball. The program asks the player if they'd like to select numbers or do a 'quickpick', where the numbers are randomly generated for them. If they opt to select numbers, prompt them to type the numbers in and validate that they are unique (except the powerball), and in the correct range. The program then generates a 'drawing' of 5 unique numbers and a Powerball, and checks it against the user's lotto ticket. It assigns winnings accordingly. Because it is so rare to win the lottery, I suggest hard coding or printing values to when testing the part of the program that assigns winnings.arrow_forwardPseudocode for the student grade calculator program, please Create a program to enter grades and calculate averages and letter grades. Need a class which will contain: Student Name Student Id Student Grades (an array of 3 grades) A constructor that clears the student data (use -1 for unset grades) Get functions for items a, b, and c, average, and letter grade Set functions for items a, n, and c Note that the get and set functions for Student grades need an argument for the grade index. Need another class which will contain: An Array of Students (1 above) A count of number of students in use You need to create a menu interface that allows you to: Add new students Enter test grades Display all the students with their names, ids, test grades, average, and letter grade Exit the program Add comments and use proper indentation. Nice Features: I would like that system to accept a student with no grades, then later add one or more grades, and when all grades are entered, calculate the…arrow_forwardCASE STUDY#:Grade Register Write a grade register program to keep and process the grades of students. The program contain a class called GradeRegister. This class has two data members known as CourseName and an array of integer called Grades. The array Grades keep the grades of students of the same course. GradeRegister class have three methods as GetHighestGrade(), GetLowestGrade() and GetAverageGrade().The method GetHighestGrade return the maximum grade as integer from the array Grades and GetLowestGrade return the minimum grade value as integer from the array Grades. The GetAverageGrade method will return the average of grades from Grades array as float value. The parameterized constructor inside GradeRegister class will take two parameters to initialize the two data members of same class as mentioned before. Write a driver class GradeRegisterDriver to declare and initialize an integer array for grades and pass through the GradeRegister class constructor along with the course name.…arrow_forward
- C# application that will allow users to enter values store all the expenses on that month in an array clothes, food, cool drinks, water, meatarrow_forwardTrue or False Objects that are instances of a class can be stored in an array.arrow_forwardWrite code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods:1. set: This method receives the array of person class and set the attribute.2. displayAll: This method will display values of all persons.3. sort: This method will sort the array according to age values of the persons with ascending order using Selection Sort.4. reverse: This method will reverse the order of the array.5. getPersons: This method will return the array of the persons (attribute).6. displayEvenAgePerson: This method will display all those persons values who have even age.7. displayOddAgePerson: This method will display all those persons values who have odd age.8. displayPrimeAgePerson: This method will display only those persons values who have prime number age.Following is the class of Person: public class Person {private String Name;public int age;public void set(String Name, int age){this.Name = Name;if(age > 0 && age <=…arrow_forward
- javaarrow_forward8. Grade Book A teacher has five students who have taken four tests. The teacher uses the following grading scale to assign a letter grade to a student, based on the average of his or her four test scores: Test Score Letter Grade 90–100 A 80-89 B 70-79 C 60-69 D 0-59 Write a class that uses a String array or an ArrayList object to hold the five students' names, an array of five characters to hold the five students' letter grades, and five arrays of four doubles each to hold each student's set of test scores. The class should have methods that return a specific student's name, the average test score, and a letter grade based on the average. Demonstrate the class in a program that allows the user to enter each student's name and his or her four test scores. It should then display each student's average test score and letter grade. Input Validation: Do not accept test scores less than zero or greater than 100.arrow_forwardClass and Data members: Create a class called Temperature that stores temperature readings (integers) in a vector (do not use an array). The class should have data members that store the month name and year of when the temperature readings were collected. Constructor(s): The class should have a 2-argument constructor that receives the month name and year as parameters and sets the appropriate data members to these values. Member Functions: The class should have functions as follows: Member functions to set and get the month and year variables. A member function that adds a single temperature to the vector. Both negative and positive temperatures are allowed. Call this function AddTemperature. A member function to sort the vector in ascending order. Feel free to use the sort function that is available in the algorithm library for sorting vectors. Or, if you would prefer to write your own sort code, you may find this site to be helpful:…arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
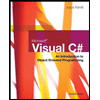
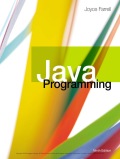