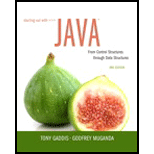
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 7.1, Problem 7.7CP
Explanation of Solution
Output of the given code:
The given code is developed into a complete
Program:
// Class definition
class Sample
{
// Main class definition
public static void main(String args[]) {
// Array declaration
int[] values = new int[5];
// Loop to get the values
for (int count = 0; count < 5; count++)
/* Increment the count value and store it in variable */
values [count] = count + 1 ;
// Read the values in the variable
for (int count = 0; count < 5; count++)
// Display the values
System...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
#include <stdio.h>
struct Single
{
int num;
};
void printSingle(int f)
{
int binaryNum[33];
int i = 0;
while(f>0)
{
binaryNum[i] = f % 2;
f = f/2;
i++;
}
for (int j=i-1; j>= 0; j--)
{
printf("%d",binaryNum[j]);
}
}
int main()
{
struct Single single;
single.num = 33;
printf("Number: %d\n",single.num);
printSingle(single.num);
return 0;
}
#include <stdio.h>
struct dna
{
int number;
char text;
char stringvalue[30];
};
int main()
{
struct dna dnavalue[5];
int i;
for(i=0; i<4; i++)
{
printf("Sample %d\n",i+1);
printf("Enter Number:\n");
scanf("%d", &dnavalue[i].number);
printf("Enter Text :\n");
scanf("%c",&dnavalue[i].text);
printf("Enter String :\n");
scanf("%s",dnavalue[i].stringvalue);
}
printf("Sample DNA Number Text String\n");
for(i=0; i<4; i++)
{
printf("%d\t\t", i+1);
printf("%d\t\t", dnavalue[i].number);
printf("%c\t\t", dnavalue[i].text);
printf("%s", dnavalue[i].stringvalue);
printf("\n");
}
return 0;
}
Write comments on every line of this program explaining what's happening briefly
a) FindMinIterative
public int FindMin(int[] arr) { int x = arr[0]; for(int i = 1; i < arr.Length; i++) { if(arr[i]< x) x = arr[i];
} return x; }
b) FindMinRecursive
public int FindMin(int[] arr, int length) { if(length == 1) return arr[0];
return Math.Min(arr[length - 1], Find(arr, length - 1));
}
What is the Big-O for this functions. Could you explain the recurisive more in details ?
Chapter 7 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 7.1 - Write statements that create the following arrays:...Ch. 7.1 - Whats wrong with the following array declarations?...Ch. 7.1 - Prob. 7.3CPCh. 7.1 - Prob. 7.4CPCh. 7.1 - Prob. 7.5CPCh. 7.1 - Prob. 7.6CPCh. 7.1 - Prob. 7.7CPCh. 7.1 - Prob. 7.8CPCh. 7.2 - Prob. 7.9CPCh. 7.2 - Prob. 7.10CP
Ch. 7.2 - A program has the following declaration: double[]...Ch. 7.2 - Look at the following statements: int[] a = { 1,...Ch. 7.3 - Prob. 7.13CPCh. 7.3 - Write a method named zero, which accepts an int...Ch. 7.6 - Prob. 7.15CPCh. 7.7 - Recall that we discussed a Rectangle class in...Ch. 7.10 - Prob. 7.17CPCh. 7.11 - What value in an array does the selection sort...Ch. 7.11 - How many times will the selection sort swap the...Ch. 7.11 - Prob. 7.20CPCh. 7.11 - Prob. 7.21CPCh. 7.11 - If a sequential search is performed on an array,...Ch. 7.13 - What import statement must you include in your...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Prob. 7.26CPCh. 7.13 - Prob. 7.27CPCh. 7.13 - Prob. 7.28CPCh. 7.13 - Prob. 7.29CPCh. 7.13 - Prob. 7.30CPCh. 7.13 - Prob. 7.31CPCh. 7 - Prob. 1MCCh. 7 - Prob. 2MCCh. 7 - Prob. 3MCCh. 7 - Prob. 4MCCh. 7 - Array bounds checking happens. a. when the program...Ch. 7 - Prob. 6MCCh. 7 - Prob. 7MCCh. 7 - Prob. 8MCCh. 7 - Prob. 9MCCh. 7 - Prob. 10MCCh. 7 - Prob. 11MCCh. 7 - To delete an item from an ArrayList object, you...Ch. 7 - Prob. 13MCCh. 7 - Prob. 14TFCh. 7 - Prob. 15TFCh. 7 - Prob. 16TFCh. 7 - Prob. 17TFCh. 7 - Prob. 18TFCh. 7 - True or False: The Java compiler does not display...Ch. 7 - Prob. 20TFCh. 7 - True or False: The first size declarator in the...Ch. 7 - Prob. 22TFCh. 7 - Prob. 23TFCh. 7 - int[] collection = new int[-20];Ch. 7 - Prob. 2FTECh. 7 - Prob. 3FTECh. 7 - Prob. 4FTECh. 7 - Prob. 5FTECh. 7 - Prob. 1AWCh. 7 - Prob. 2AWCh. 7 - Prob. 3AWCh. 7 - In a program you need to store the populations of...Ch. 7 - In a program you need to store the identification...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Prob. 9AWCh. 7 - Prob. 10AWCh. 7 - Prob. 11AWCh. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - Prob. 3SACh. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 7SACh. 7 - Prob. 8SACh. 7 - Prob. 9SACh. 7 - Rainfall Class Write a RainFall class that stores...Ch. 7 - Payroll Class Write a Payroll class that uses the...Ch. 7 - Charge Account Validation Create a class with a...Ch. 7 - Charge Account Modification Modify the charge...Ch. 7 - Prob. 5PCCh. 7 - Drivers License Exam The local Drivers License...Ch. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Grade Book A teacher has five students who have...Ch. 7 - Grade Book Modification Modify the grade book...Ch. 7 - Average Steps Taken A Personal Fitness Tracker is...Ch. 7 - Array Operations Write a program with an array...Ch. 7 - 12.1994 Gas Prices In the student sample programs...Ch. 7 - Sorted List of 1994 Gas Prices Note: This...Ch. 7 - Name Search If you have downloaded this books...Ch. 7 - Population Data If you have downloaded this books...Ch. 7 - World Series Champions If you have downloaded this...Ch. 7 - 2D Array Operations Write a program that creates a...Ch. 7 - Prob. 18PCCh. 7 - Trivia Game In this programming challenge, you...Ch. 7 - Prob. 20PC
Knowledge Booster
Similar questions
- #include <stdio.h>#include <stdlib.h> int MAX_SIZE = 10;int n = 0; //A counter variable which will keep track of number of elements in arr. void append(int *arr, int element){ if(n == MAX_SIZE) { MAX_SIZE = MAX_SIZE * 2; int *ptr = (int*)malloc(MAX_SIZE * sizeof(int)); //Copy elements of existing array to a new array for(int i=0;i<n;i++) { ptr[i] = arr[i]; } arr = ptr; n++; } arr[n] = element;} int get(int *arr, int index){ return arr[index];}int main(){ int *arr = (int*)malloc(MAX_SIZE * sizeof(int)); n = 10; for(int i=0;i<n;i++) arr[i] = i+1; printf("\nArray size: %d", MAX_SIZE); printf("\nNumber of elements: %d", n); printf("\nArray: "); for(int i=0;i<n;i++) printf("%d ", arr[i]); printf("\n\nAdding an element"); append(arr, 11); printf("\nArray size: %d", MAX_SIZE); printf("\nNumber of elements: %d", n);…arrow_forward#include <stdio.h>#include <stdlib.h> int MAX_SIZE = 10;int n = 0; //A counter variable which will keep track of number of elements in arr. void append(int *arr, int element){ if(n == MAX_SIZE) { MAX_SIZE = MAX_SIZE * 2; int *ptr = (int*)malloc(MAX_SIZE * sizeof(int)); //Copy elements of existing array to a new array for(int i=0;i<n;i++) { ptr[i] = arr[i]; } arr = ptr; n++; } arr[n] = element;} int get(int *arr, int index){ return arr[index];}int main(){ int *arr = (int*)malloc(MAX_SIZE * sizeof(int)); n = 10; for(int i=0;i<n;i++) arr[i] = i+1; printf("\nArray size: %d", MAX_SIZE); printf("\nNumber of elements: %d", n); printf("\nArray: "); for(int i=0;i<n;i++) printf("%d ", arr[i]); printf("\n\nAdding an element"); append(arr, 11); printf("\nArray size: %d", MAX_SIZE); printf("\nNumber of elements: %d", n);…arrow_forward#include <stdio.h>#include <stdlib.h> int MAX_SIZE = 10;int n = 0; //A counter variable which will keep track of number of elements in arr. void append(int *arr, int element){ if(n == MAX_SIZE) { MAX_SIZE = MAX_SIZE * 2; int *ptr = (int*)malloc(MAX_SIZE * sizeof(int)); //Copy elements of existing array to a new array for(int i=0;i<n;i++) { ptr[i] = arr[i]; } arr = ptr; n++; } arr[n] = element;} int get(int *arr, int index){ return arr[index];}int main(){ int *arr = (int*)malloc(MAX_SIZE * sizeof(int)); n = 10; for(int i=0;i<n;i++) arr[i] = i+1; printf("\nArray size: %d", MAX_SIZE); printf("\nNumber of elements: %d", n); printf("\nArray: "); for(int i=0;i<n;i++) printf("%d ", arr[i]); printf("\n\nAdding an element"); append(arr, 11); printf("\nArray size: %d", MAX_SIZE); printf("\nNumber of elements: %d", n);…arrow_forward
- #include <stdio.h>#include <stdlib.h> int MAX_SIZE = 10;int n = 0; //A counter variable which will keep track of number of elements in arr. void append(int *arr, int element){ if(n == MAX_SIZE) { MAX_SIZE = MAX_SIZE * 2; int *ptr = (int*)malloc(MAX_SIZE * sizeof(int)); //Copy elements of existing array to a new array for(int i=0;i<n;i++) { ptr[i] = arr[i]; } arr = ptr; n++; } arr[n] = element;} int get(int *arr, int index){ return arr[index];}int main(){ int *arr = (int*)malloc(MAX_SIZE * sizeof(int)); n = 10; for(int i=0;i<n;i++) arr[i] = i+1; printf("\nArray size: %d", MAX_SIZE); printf("\nNumber of elements: %d", n); printf("\nArray: "); for(int i=0;i<n;i++) printf("%d ", arr[i]); printf("\n\nAdding an element"); append(arr, 11); printf("\nArray size: %d", MAX_SIZE); printf("\nNumber of elements: %d", n);…arrow_forward#include <stdio.h>#include <string.h> int findRepeat(char* s){int p , i , j; p = -1; for (i = 0 ; i < strlen(s) ; i++){for (j = i + 1; j < strlen(s); j++){if (s[i] == s[j]){p = i;break;}}if (p != -1)break;}return p;} int main(){char str[] = "Hello World";int pos = findRepeat(str);if (pos == -1)printf("Not found");elseprintf("%c", str[pos]);return 0;}can you please explain this code for mearrow_forwardint* p: int a[3]{1, 2, 3}; p = a; What is the value of *(p+2)?arrow_forward
- int X[10]={2,0,6,11,4,5,9,11,-2,-1); From the code above, what is the value of X[8] ?arrow_forwardint a[ 10] = {1,5,3,8,4,2,6,9,7}; int *p; p = &a[2]; p[0] = 10; p[1] = 15; What are the values of a? Select one: O a. {10,15,3,8,4,2,6,9,7} O b. {1,15,10,8,4,2,6,9,7} O c. {1,5,10,15,4,2,6,9,7} O d. {1,10,15,8,4,2,6,9,7}arrow_forward#include using namespace std; int find(int arr[], int value, int left, int right) { int midpt = (left+right)/2; if (left > right) return -1; if ( arr[midpt] return midpt; else if (arr[midpt] < value) == value) return else return find(arr,value, left,midpt-1); } void main(void) { int arr[] ={4,5,6,9,11}; cout<arrow_forward#include using namespace std; const int y = 1; int main () { int static y = 2; int i = 3, j = 4, m = 5, n = 6; %3D int a = [] (int x, int i = 1) { return x * i; } (y, 3) ; %3D int b = [=] (int x) { return [=] (int b) { return b + j; } (x) % 7; } (a); int c = [=] (int x) mutable ->int { m = 6; return [6] (int j) mutable { y = a * b; return y / j; } (x) -m; } (b) ; cout << a << endl; cout << b << endl; cout << c << endl; cout << m << endl; cout << y < endl; return 0; This program outputs 5 lines. What are they? Please explain your work and your answer.arrow_forwardWhat is the output of the following code? int *intArrayPtr;int *temp;intArrayPtr = new int[5];*intArrayPtr = 7;temp = intArrayPtr;for (int i = 1; i < 5; i++){intArrayPtr++;*intArrayPtr = *(intArrayPtr - 1) 1 2 * i;}intArrayPtr = temp;for (int i = 0; i < 5; i++){cout << *intArrayPtr << " ";intArrayPtr++;}cout << endl;arrow_forwardWhat the following code does? int * foo = new int [5]; if (!foo) { ..}arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
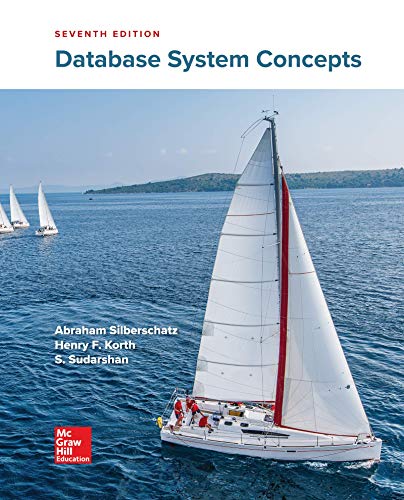
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
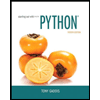
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
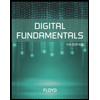
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
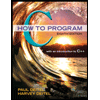
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
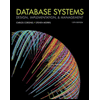
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
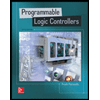
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education