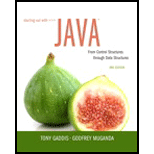
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7, Problem 2FTE
Program Plan Intro
Array:
An array is defined as a group that consists of similar data types.
- The array size must be specified by an “int” value and not long or short.
- In Java, all arrays are allocated dynamically.
- An array is always indexed, starting from 0.
Declaration of an array Variable:
- An array variable is declared with [] after the data type.
- The following is the syntax for declaring an array variable.
- elementType[] arrayRefVar;
or
- elementType arrayRefVar[];
- elementType[] arrayRefVar;
- Both the above given statements can be used, but the former one is mostly used.
- The element type can be any data type and the same data type must be used by all the elements.
- The values are given inside the initialization list, the list is given inside the braces.
Example:
int[ ] arr;
char[ ] arr;
Array with initialization list:
int[ ] arr = {1, 2, 3, 4};
Declaring an array reference and creating an array:
- To insert elements to an array, first an array has to be created by using new operator and then the reference variable is assigned.
- The syntax is as follows,
arrayRefVar = new elementType[arraySize]
- From the above statement it is clear that, an array is created using new elementType[arraySize] and then the reference to the newly created array is assigned to the variable arrayRefVar.
- It is possible to declare an array variable, create an array and assign the reference to a variable in a single statement itself.
The syntax will be as follows,
elementType[]arrayRefVar = new elementType[arraySize];
elementType arrayRefVar[]= new elementType[arraySize);
Example:
double myFile = new double[20];
- The statement given above declares an array variable myFile and then creates an array that consists of 20 elements which are of the datatype double and at last assign the reference to myFile.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
char[ ] letters=new char[5];int x = 0;while(x < 10){ar[x]='a';x++;}
Task 3: Statistics using arrays:
by java programming
With the spread of COVID 19, the HR department in a company has decided to conduct some statistics
among the employees in order to determine the number of infections according to some conditions.
For each employee, they have to record the code, name, age, whether he/she was infected or no and
the remaining days of leaves for him/her.
You are requested to write the program that maintains the lists of details for the employees as
mentioned above using the concept of arrays. The program repeats the display of a menu of services
until the user decides to exit.
1. Start by initializing the employee details by reading them from the keyboard.
2. Repeat the display of a menu of 4 services, perform the required task according to the user’s
choice and asks the user whether he/she wants to repeat or no. You need to choose one service
from each category (‘A’,’B’,’C’,’D’)
a. A. Display the total number of employees that were infected
b. B.…
Selective Sum
Code in C language
Chapter 7 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 7.1 - Write statements that create the following arrays:...Ch. 7.1 - Whats wrong with the following array declarations?...Ch. 7.1 - Prob. 7.3CPCh. 7.1 - Prob. 7.4CPCh. 7.1 - Prob. 7.5CPCh. 7.1 - Prob. 7.6CPCh. 7.1 - Prob. 7.7CPCh. 7.1 - Prob. 7.8CPCh. 7.2 - Prob. 7.9CPCh. 7.2 - Prob. 7.10CP
Ch. 7.2 - A program has the following declaration: double[]...Ch. 7.2 - Look at the following statements: int[] a = { 1,...Ch. 7.3 - Prob. 7.13CPCh. 7.3 - Write a method named zero, which accepts an int...Ch. 7.6 - Prob. 7.15CPCh. 7.7 - Recall that we discussed a Rectangle class in...Ch. 7.10 - Prob. 7.17CPCh. 7.11 - What value in an array does the selection sort...Ch. 7.11 - How many times will the selection sort swap the...Ch. 7.11 - Prob. 7.20CPCh. 7.11 - Prob. 7.21CPCh. 7.11 - If a sequential search is performed on an array,...Ch. 7.13 - What import statement must you include in your...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Prob. 7.26CPCh. 7.13 - Prob. 7.27CPCh. 7.13 - Prob. 7.28CPCh. 7.13 - Prob. 7.29CPCh. 7.13 - Prob. 7.30CPCh. 7.13 - Prob. 7.31CPCh. 7 - Prob. 1MCCh. 7 - Prob. 2MCCh. 7 - Prob. 3MCCh. 7 - Prob. 4MCCh. 7 - Array bounds checking happens. a. when the program...Ch. 7 - Prob. 6MCCh. 7 - Prob. 7MCCh. 7 - Prob. 8MCCh. 7 - Prob. 9MCCh. 7 - Prob. 10MCCh. 7 - Prob. 11MCCh. 7 - To delete an item from an ArrayList object, you...Ch. 7 - Prob. 13MCCh. 7 - Prob. 14TFCh. 7 - Prob. 15TFCh. 7 - Prob. 16TFCh. 7 - Prob. 17TFCh. 7 - Prob. 18TFCh. 7 - True or False: The Java compiler does not display...Ch. 7 - Prob. 20TFCh. 7 - True or False: The first size declarator in the...Ch. 7 - Prob. 22TFCh. 7 - Prob. 23TFCh. 7 - int[] collection = new int[-20];Ch. 7 - Prob. 2FTECh. 7 - Prob. 3FTECh. 7 - Prob. 4FTECh. 7 - Prob. 5FTECh. 7 - Prob. 1AWCh. 7 - Prob. 2AWCh. 7 - Prob. 3AWCh. 7 - In a program you need to store the populations of...Ch. 7 - In a program you need to store the identification...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Prob. 9AWCh. 7 - Prob. 10AWCh. 7 - Prob. 11AWCh. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - Prob. 3SACh. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 7SACh. 7 - Prob. 8SACh. 7 - Prob. 9SACh. 7 - Rainfall Class Write a RainFall class that stores...Ch. 7 - Payroll Class Write a Payroll class that uses the...Ch. 7 - Charge Account Validation Create a class with a...Ch. 7 - Charge Account Modification Modify the charge...Ch. 7 - Prob. 5PCCh. 7 - Drivers License Exam The local Drivers License...Ch. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Grade Book A teacher has five students who have...Ch. 7 - Grade Book Modification Modify the grade book...Ch. 7 - Average Steps Taken A Personal Fitness Tracker is...Ch. 7 - Array Operations Write a program with an array...Ch. 7 - 12.1994 Gas Prices In the student sample programs...Ch. 7 - Sorted List of 1994 Gas Prices Note: This...Ch. 7 - Name Search If you have downloaded this books...Ch. 7 - Population Data If you have downloaded this books...Ch. 7 - World Series Champions If you have downloaded this...Ch. 7 - 2D Array Operations Write a program that creates a...Ch. 7 - Prob. 18PCCh. 7 - Trivia Game In this programming challenge, you...Ch. 7 - Prob. 20PC
Knowledge Booster
Similar questions
- Count consecutive summers def count_consecutive_summers(n): Like a majestic wild horse waiting for the rugged hero to tame it, positive integers can be broken down as sums of consecutive positive integers in various ways. For example, the integer 42 often used as placeholder in this kind of discussions can be broken down into such a sum in four different ways: (a) 3 + 4 + 5 + 6 + 7 + 8 + 9, (b) 9 + 10 + 11 + 12, (c) 13 + 14 + 15 and (d) 42. As the last solution (d) shows, any positive integer can always be trivially expressed as a singleton sum that consists of that integer alone. Given a positive integer n, determine how many different ways it can be expressed as a sum of consecutive positive integers, and return that count. The number of ways that a positive integer n can be represented as a sum of consecutive integers is called its politeness, and can also be computed by tallying up the number of odd divisors of that number. However, note that the linked Wikipedia de0inition…arrow_forwardMatch each data operator with its function: OFFSET Returns the number of the elements in an array. TYPE Returns the size of a variables byte. SIZEOF Returns the memory adress of a variable. LENGTHOF Returns number of bytes used in an array.arrow_forwardThe index type of an array can be any data type. True or false?arrow_forward
- TRUE or FALSE IN JAVA You can obtain the number of rows using Array.length, and the number of columns in a specified row using array[row].length.arrow_forwardfunction main() { # ist: input numbers #w: outer for loop index # X: inner for loop index # y: number of parsmeters # min: index for max value at the time of iteration # buf: used for swapping ____________________a _______ #declare local vars let=___________b___ #intialize aary with the parametrs y= _______c___ # find the lenght of lstfor((________________)); do min=________e_____ # intialize main index for ((___________f_______)); do # find index for main value in one line. use a short tets. _________g_____done # swap- two values using two indices, min and outerloop # use buf to hold value when swapping ________________h________ # move min lst [.] to buf ________________i_______ # move lst[.] to lst[.] _________________j_____ # move buf to lst[.]done}main "@" # pass the input parameters to the function main# end of bash script show me the ss when u run chatgpt doesnt give right codearrow_forwardAn array name, can begin with a number. Select one: True Falsearrow_forward
- An array that uses two indices is referred to as a(n) ________________array.arrow_forwardC++ language Write a program named lower-half which takes input for a two dimensional array of 5 rows and5 columns and prints the lower half of the array.For example if user enters the following numbers:34915, 10501, 25781, 71531, 23150Then the output is:5, 01, 781, 1531, 23150arrow_forward8arrow_forward
- Write a function to determine the resultant force vector R of the two forces F and F2 applied to the bracket, where 01 of unit vector along the x and y axis. R must be a vector, for example R = [Rx, R,]. The coordinate system is shown in the figure below: = 30° and 02 = 35°. Write R in terms F1 F2 30% 35°arrow_forwardprogramming used: javaarrow_forwardAn array definition reserves space for the array. true or falsearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
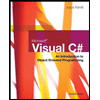
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
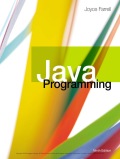
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
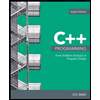
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
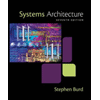
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
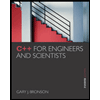
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr