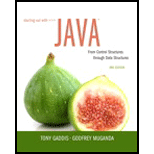
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 7, Problem 5PC
Program Plan Intro
Greater than n
- Import the required packages.
- Declare the class “Main”.
- Declare the “main ()” method.
- Declare an array and initialize it with some numbers.
- Create an object for the scanner class.
- Get a value form the user.
- Call the function “displayGreaterThan ()”.
- Function definition for “displayGreaterThan ()”.
- Loop through the entire array.
- Check if the value of array is greater than the user given number.
- Print the value.
- Check if the value of array is greater than the user given number.
- Loop through the entire array.
- Declare the “main ()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
5. Larger Than n
In a program, write a method that accepts two arguments: an array and a number n. Assume
that the array contains integers. The method should display all of the numbers in the array
that are greater than the number n.
Homework - identical arrays for one-dimensional:
Write a test program which prompts a user to enter the size of two arrays as well as to fill
their elements. After that, the program checks the same contents of the elements of the two
arrays. If these arrays are equal, the program displays message which is "The two arrays are
identical". If not equal, displaying "The two arrays are not identical".
Write an equals method that reads two arrays and displays these messages above.
Use Java programming language
Write a program that asks the user to enter 5 test grades (use an array to store them).
Output the grades entered, the lowest and highest grade, the average grade, how many grades are above the average and how many are below and the letter grade for the average grade.
Create a method that returns the lowest grade.
Create a method that returns the highest grade.
Create a method that returns the average grade.
Create a method that returns how many grades were above the average.
Create a method that returns how many grades were below the average.
Create a method that returns the letter grade of the average (90-100 – A, 80-89 – B, 70-79 – C, < 70 – F)
Chapter 7 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 7.1 - Write statements that create the following arrays:...Ch. 7.1 - Whats wrong with the following array declarations?...Ch. 7.1 - Prob. 7.3CPCh. 7.1 - Prob. 7.4CPCh. 7.1 - Prob. 7.5CPCh. 7.1 - Prob. 7.6CPCh. 7.1 - Prob. 7.7CPCh. 7.1 - Prob. 7.8CPCh. 7.2 - Prob. 7.9CPCh. 7.2 - Prob. 7.10CP
Ch. 7.2 - A program has the following declaration: double[]...Ch. 7.2 - Look at the following statements: int[] a = { 1,...Ch. 7.3 - Prob. 7.13CPCh. 7.3 - Write a method named zero, which accepts an int...Ch. 7.6 - Prob. 7.15CPCh. 7.7 - Recall that we discussed a Rectangle class in...Ch. 7.10 - Prob. 7.17CPCh. 7.11 - What value in an array does the selection sort...Ch. 7.11 - How many times will the selection sort swap the...Ch. 7.11 - Prob. 7.20CPCh. 7.11 - Prob. 7.21CPCh. 7.11 - If a sequential search is performed on an array,...Ch. 7.13 - What import statement must you include in your...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Prob. 7.26CPCh. 7.13 - Prob. 7.27CPCh. 7.13 - Prob. 7.28CPCh. 7.13 - Prob. 7.29CPCh. 7.13 - Prob. 7.30CPCh. 7.13 - Prob. 7.31CPCh. 7 - Prob. 1MCCh. 7 - Prob. 2MCCh. 7 - Prob. 3MCCh. 7 - Prob. 4MCCh. 7 - Array bounds checking happens. a. when the program...Ch. 7 - Prob. 6MCCh. 7 - Prob. 7MCCh. 7 - Prob. 8MCCh. 7 - Prob. 9MCCh. 7 - Prob. 10MCCh. 7 - Prob. 11MCCh. 7 - To delete an item from an ArrayList object, you...Ch. 7 - Prob. 13MCCh. 7 - Prob. 14TFCh. 7 - Prob. 15TFCh. 7 - Prob. 16TFCh. 7 - Prob. 17TFCh. 7 - Prob. 18TFCh. 7 - True or False: The Java compiler does not display...Ch. 7 - Prob. 20TFCh. 7 - True or False: The first size declarator in the...Ch. 7 - Prob. 22TFCh. 7 - Prob. 23TFCh. 7 - int[] collection = new int[-20];Ch. 7 - Prob. 2FTECh. 7 - Prob. 3FTECh. 7 - Prob. 4FTECh. 7 - Prob. 5FTECh. 7 - Prob. 1AWCh. 7 - Prob. 2AWCh. 7 - Prob. 3AWCh. 7 - In a program you need to store the populations of...Ch. 7 - In a program you need to store the identification...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Prob. 9AWCh. 7 - Prob. 10AWCh. 7 - Prob. 11AWCh. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - Prob. 3SACh. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 7SACh. 7 - Prob. 8SACh. 7 - Prob. 9SACh. 7 - Rainfall Class Write a RainFall class that stores...Ch. 7 - Payroll Class Write a Payroll class that uses the...Ch. 7 - Charge Account Validation Create a class with a...Ch. 7 - Charge Account Modification Modify the charge...Ch. 7 - Prob. 5PCCh. 7 - Drivers License Exam The local Drivers License...Ch. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Grade Book A teacher has five students who have...Ch. 7 - Grade Book Modification Modify the grade book...Ch. 7 - Average Steps Taken A Personal Fitness Tracker is...Ch. 7 - Array Operations Write a program with an array...Ch. 7 - 12.1994 Gas Prices In the student sample programs...Ch. 7 - Sorted List of 1994 Gas Prices Note: This...Ch. 7 - Name Search If you have downloaded this books...Ch. 7 - Population Data If you have downloaded this books...Ch. 7 - World Series Champions If you have downloaded this...Ch. 7 - 2D Array Operations Write a program that creates a...Ch. 7 - Prob. 18PCCh. 7 - Trivia Game In this programming challenge, you...Ch. 7 - Prob. 20PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- programming used: javaarrow_forwardTOPIC: Passing Array to a Method and Returning Create a program that will determine the Second Largest number and the Second Smallest Number in an array Display the second largest and second smallest.arrow_forwardThis program calculates the average of 3 test scores and the grade is assigned according to the table below: Average Score Letter Grade 90 - 100 A 80 - 89 B 70 - 79 C 60 - 69 D Below 60 F The program asks the user to enter N number of students. The program should display a letter grade for each student. The program should consist of the following methods: calcAverage - this method accepts N number of students as arguments and returns the array of the average scores for N students. determineGrade - this method should accept the array of the average scores as an argument and display the letter grade for each N student. Input: 3 90 80 70 80 67 88 77 98 67 The first line is the number of students, while the following line is the test score for each student. Expected Output: Grade: B Grade: C Grade: Barrow_forward
- 1. Shift Left k Cells Use Python Consider an array named source. Write a method/function named shiftLeft( source, k) that shifts all the elements of the source array to the left by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] shiftLeft(source,3) After calling shiftLeft(source,3), printing the array should give the output as: [ 40, 50, 60, 0, 0, 0 ]arrow_forwardWrite a C# code that contains an integer array whose size is 6. The following method will assign random values between 10 and 70 to the array will display the values assigned to the array public static void CreateandDisplayElements(int[] a) The following method will find the total number of the elements less than 40. public static int NumbersGreaterThan40(int[] a)arrow_forwardComputer Programming 1 Homework #2 Write a Java program to make two arrays containing random integer values (You can make these arrays with specific sizes using the below makeRandomArray method). The program should sum these two arrays and prints the resulted array (the array that contains the result of the two arrays summing). The program should also show the maximum element of the resulted array. Your program must define and call the following methods to perform the program as illustrated above: 1- A method int [] makeRandomArray(int size ,int a , int b) that makes and returns an array with specific size. The methods fills this array with random integers in a range [a..b] 2- A method public static int findmax(int x[ ]) that finds the maximum value of a number of x array passed to the method. 3- A method public static void printArray(int x[]) that prints the elements of the array x passed to the method. 4- A method public int[ ] sumTwoArrays(int x[ ], int y[ ]) that sums the elements…arrow_forward
- Programming language: Java Write a program do the following: 1- ask student to enter name 2- ask student to enter the number of school subjects 3- Declare an array the same size as the number of school subjects. 4- ask the students to enter the marks in the array. 5- display the student name and the marks.arrow_forward2. The reserve method reserves an array by copying it to a new array. Rewrite the method that reverse the array passed in the argument and returns this array. Write a test program that prompts the user to enter ten numbers, invokes the method to reverse the numbers and displays the numbers.arrow_forwardAssignment: Create a program which does the following: 1. Asks the user for 5 numbers and stores them in an array. 2. Prints out the numbers entered. 3. Prints out the average of all the numbers 100 or less. Cree un programa que haga lo siguiente: 1. Imprime los números ingresados. 2. Imprime los números ingresados. 3. Imprime el promedio de todos los números 100 o menos. An example of possible output is shown below: A continuación se muestra un ejemplo de salida posible. Output You entered the following numbers: 101 100 155 90 65 There were 2 numbers larger than 100 [101, 100, 155, 90, 65] The average of the numbers 100 or smaller is: 85.0arrow_forward
- When you pass an array to a method, the method receives: the address of the array a copy of the first element in the array a copy of the array nothingarrow_forwardComputer sciencearrow_forward2. Rotate Left k cells Use Python Consider an array named source. Write a method/function named rotateLeft( source, k) that rotates all the elements of the source array to the left by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] rotateLeft(source,3) After calling rotateLeft(source,3), printing the array should give the output as: [ 40, 50, 60, 10, 20, 30]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
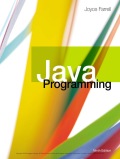
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
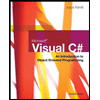
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,