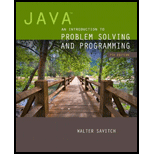
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 6, Problem 11E
Create a class Android whose objects have unique data. The class has the following attributes:
- tag—a static integer that begins at 1 and changes each time an instance is created
- name—a string that is unique for each instance of this class
Android has the following methods:
- Android—default constructor that sets the name to “Bob” concatenated with the value of tag. After setting the name, this constructor changes the value of tag by calling the private method changeTag.
- getName—returns the name portion of the invoking object.
- isPrime(n)—a private static method that returns true if n is prime—that is, if it is not divisible by any number from 2 to n − 1.
- changeTag—a private static method that replaces tag with the next prime number larger than the current value of a tag.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Focus on classes, objects, methods and good programming style
Your task is to create a BankAccount class.
Class name
BankAccount
Attributes
_balance
float
_pin
integer
Methods
init ()
get_pin()
check pin ()
deposit ()
withdraw ()
get_balance ()
The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The
pin should be generated randomly when the account object is created. The initial balance should
be 0.
get_pin () should return the pin.
check_pin (pin) should check the argument against the saved pin and return True if it
matches, False if it does not.
deposit (amount) should receive the amount as the argument, add the amount to the account
and return the new balance.
withraw (amount) should check if the amount can be withdrawn (not more than is in
the account), If so, remove the argument amount from the account and return the new balance if
the transaction was successful. Return False if it was not.
get_balance () should return the current balance.…
Java - Constructors
Create a class named Book that has the following attributes:
Publisher - String Year Published - Integer Price - Double Title - String
Create a constructor for the class Book that has the parameters from the attributes of the class. After, create a method for the class that displays all attributes. Ask the user for inputs for the attributes and use the constructor to create the object. Lastly, print the values of the attributes.
Inputs
1. Publisher
2. Year Published
3. Price
4. Book Title
XYZ Publishing 2020 1000.00 How to Win?
Sample Output
Published: ABC Publishing
Year Published: 2022
Price: 150.00
Book Title: Stooping too low
Stooping too low is published by ABC Publishing on 2022. It sells at the price of Php150.00.
14.23 LAB: Dean's list
Students make the Dean's list if their GPA is 3.5 or higher. Complete the Course class by implementing the get_deans_list() instance
method, which returns a list of students with a GPA of 3.5 or higher.
The file main.py contains:
. The main function for testing the program.
Class Course represents a course, which contains a list of student objects as a course roster. (Type your code in here.)
• Class Student represents a classroom student, which has three attributes: first name, last name, and GPA. (Hint: get_gpa() returns a
student's GPA.)
Note: For testing purposes, different student values will be used.
Ex. For the following students:
Henry Nguyen 3.5
Brenda Stern 2.0
Lynda Robison 3.2
Sonya King 3.9
the output is:
Dean's list:
Henry Nguyen (GPA: 3.5)
Sonya King (GPA: 3.9)
Chapter 6 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 6.1 - If a class is named Student, what name can you use...Ch. 6.1 - When defining a constructor, what do you specify...Ch. 6.1 - What is a default constructor?Ch. 6.1 - Does every class in Java automatically have a...Ch. 6.1 - In the program PetDemo shown in Listing 6 2, you...Ch. 6.2 - Prob. 6STQCh. 6.2 - Can a class contain both instance variables and...Ch. 6.2 - Can you reference a static variable by name within...Ch. 6.2 - Can you reference an instance variable by name...Ch. 6.2 - Can you reference a static variable by name within...
Ch. 6.2 - Can you reference an instance variable by name...Ch. 6.2 - Is the following valid, given the class...Ch. 6.2 - Prob. 13STQCh. 6.2 - Prob. 14STQCh. 6.2 - Prob. 15STQCh. 6.2 - Is the following valid, given the class...Ch. 6.2 - What values are returned by each of the following?...Ch. 6.2 - Suppose that speed is a variable of type double...Ch. 6.2 - Repeat the previous question, but instead assign...Ch. 6.2 - Suppose that nl is of type int and n2 is of type...Ch. 6.2 - Define a class CircleCalculator that hat only two...Ch. 6.2 - Which of the following statements are legal?...Ch. 6.2 - Write a Java expression to convert the number in...Ch. 6.2 - Consider the variable 5 of type String that...Ch. 6.2 - Repeat the previous question, but accommodate a...Ch. 6.2 - Write Java code to display the largest and...Ch. 6.3 - Prob. 27STQCh. 6.3 - Consider the variable allCents in the method...Ch. 6.3 - What is wrong with a program that starts as...Ch. 6.3 - Prob. 30STQCh. 6.3 - In your definition of the class OutputFormat. In...Ch. 6.4 - Prob. 32STQCh. 6.4 - Prob. 33STQCh. 6.4 - Prob. 34STQCh. 6.4 - Consider the class Species in Listing 5.19 of...Ch. 6.4 - Repeat the previous question for a method...Ch. 6.4 - Still considering the class Species in Listing...Ch. 6.4 - Rewrite the method add in Listing 6.16 so that it...Ch. 6.4 - In Listing 6.16, the set method that has a String...Ch. 6.5 - Give the definitions of three accessor methods...Ch. 6.6 - If cardSuit is an instance of Suit and is assigned...Ch. 6.7 - Suppose you want to use classes in the package...Ch. 6.7 - Prob. 43STQCh. 6.7 - Can a package have any name you might want, or are...Ch. 6.7 - On your system, place the class Pet (Listing 6.1)...Ch. 6.8 - Prob. 46STQCh. 6.8 - Prob. 47STQCh. 6.8 - Prob. 48STQCh. 6.8 - Prob. 49STQCh. 6.8 - Prob. 50STQCh. 6.8 - Prob. 51STQCh. 6.8 - Revise the applet in Listing 6.24 so that the...Ch. 6 - Prob. 1ECh. 6 - Prob. 2ECh. 6 - Write a default constructor and a second...Ch. 6 - Write a constructor for the class...Ch. 6 - Consider a class characteristic that will be used...Ch. 6 - Create a class RoomOccupancy that can be used to...Ch. 6 - Write a program that tests the class RoomOccupancy...Ch. 6 - Sometimes we would like a class that has just a...Ch. 6 - Create a program that tests the class Merlin...Ch. 6 - In the previous chapter, Self-Test Question 16...Ch. 6 - Create a class Android whose objects have unique...Ch. 6 - Prob. 12ECh. 6 - Modify the definition of the class Species in...Ch. 6 - Prob. 2PCh. 6 - Using the class Pet from Listing 6.1, write a...Ch. 6 - Do Practice Program 4 from Chapter 5 except define...Ch. 6 - The following class displays a disclaimer every...Ch. 6 - Do Practice Program 5 from Chapter 5 but add a...Ch. 6 - We can improve the Beer class from the previous...Ch. 6 - Define a utility class for displaying values of...Ch. 6 - Write a new class TruncatedDollarFormat that is...Ch. 6 - Complete and fully test the class Time that...Ch. 6 - Complete and fully test the class Characteristic...Ch. 6 - Write a Java enumeration LetterGrade that...Ch. 6 - Complete and fully test the class Per n that...Ch. 6 - Write a Temperature class that represents...Ch. 6 - Repeat Programming Project 8 of the previous...Ch. 6 - Write and fully test a class that represents...Ch. 6 - Write a program that will record the votes for one...Ch. 6 - Repeat Programming Project 10 from Chapter 5, but...Ch. 6 - Prob. 12PPCh. 6 - Prob. 13PPCh. 6 - Prob. 14PPCh. 6 - Prob. 15PP
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
In this chapter, we use the metaphor of a cookie cutter and cookies that are made from the cookie cutter to des...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
When a variable is said to reference an object, what is actually stored in the variable?
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Describe the primary differences between the conceptual and logical data models.
Modern Database Management (12th Edition)
Identity any errors in the following array declarations: a. int x[4] = {8,7, 6, 4, 3}; b. int x[] = { 8, 7, 6, ...
Problem Solving with C++ (9th Edition)
Use what you've learned about the binary numbering system in this chapter to convert the following binary numbe...
Starting Out with Programming Logic and Design (4th Edition)
The decimal number 17 is equal to the binary number 10010 11000 10001 01001
Digital Fundamentals (11th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- in C# languageDesign a Book class that holds the title, author’s name, and price of the book. Books’s constructor shouldinitialize all of these data members except the price which is set to 500/-. Create a display method thatdisplays all fields.All Books are priced at 500/- unless they are PopularBooks. The PopularBooks subclass replaces theBookprice and sets each Book’s price to 50,000/- through PopularBooks construcor. Override the displaymethod to display all fields.Write a Main () method that declares an array of five Book objects. Ask the user to enter the title andauthor for each of the 5 Books. Consider the Book to be a PopularBook if the author is one of the following:Khaled Hosseini, Oscar Wilde, or Rembrandt. Display the five Books’ details.arrow_forwardIn Javascript, Pet Class: Design a class named Pet, which should have the following fields and methods: name: The name of a pet type: The type of animal the pet is ("Dog", "Cat", "Lizard", etc.) age: The age of the pet. setName: The setter for the name field. getName: The getter for the name field. setType: The setter for the type field. getType: The getter for the type field. setAge: The setter for the age field. getAge: The getter for the age field. Once you have designed the class, create an instance of the class and use the setter and getter methods to store data in the object and display the data.arrow_forwardAssignment:The BankAccount class models an account of a customer. A BankAccount has the followinginstance variables: A unique account id sequentially assigned when the Bank Account is created. A balance which represents the amount of money in the account A date created which is the date on which the account is created.The following methods are defined in the BankAccount class: Withdraw – subtract money from the balance Deposit – add money to the balance Inquiry on:o Balanceo Account ido Date createdarrow_forward
- mau Open Leathing inta... - The class has data members that can hold the name of your cube, length of one of the sides and the color of your cube. - The class has a constructor that accepts the name, length of one of the sides, and color as arguments and sets the data members to those values. - The class has the following methods to set the corresponding data member. setName(string newName) setSide(double newSide) setColor(string newColor) - The class has a method called getVolume() that returns the volume of the cube, calculated by cubing the length of the side. - The class has a method called volumelncrease(double newVolume) that receive the percent the volume should increase, and then the side to the corresponding value. i - For example, 2.5 indicates 2.5% increasing of the volume. So you take the current volume, increase it by 2.5%, and then find the cube root to calculate the new side length. You can use the built in function called cbrt, part of the math library, to find the cube…arrow_forwardShoppingCart.java - Class definition ShoppingCartManager.java - Contains main() method Build the ShoppingCart class with the following specifications. Private fields String customerName - Initialized in default constructor to "none" String currentDate - Initialized in default constructor to "January 1, 2016" ArrayList cartItems Default constructor Parameterized constructor which takes the customer name and date as parameters Public member methods getCustomerName() accessor getDate() accessor addItem() Adds an item to cartItems array. Has a parameter of type ItemToPurchase. Does not return anything. removeItem() Removes item from cartItems array. Has a string (an item's name) parameter. Does not return anything. If item name cannot be found, output a message: Item not found in cart. Nothing removed. modifyItem() Modifies an item's description, price, and/or quantity. Has a parameter of type ItemToPurchase. Does not return anything. If item can be found (by name) in cart,…arrow_forwardA field has access that is somewhere between public and private. static final Opackage Oprotectedarrow_forward
- Using oop in java Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score.arrow_forwardStatic & Not Final Field: Accessed by every object, Changing Non-Static & Final Field: Accessed by object itself, Non-Changing Static & Final: Accessed by every object, Non-Changing Non-Static & Not Final Field: Accessed by object itself, ChangingRead the following situation and decide how the variables should be defined. You have a class named HeartsPlayerA round of Hearts starts with every player having 13 cardsPlayers then choose 3 cards to “trade” with a player (1st you pass left, 2nd you pass right, 3rd you pass across, 4th you keep)Players then strategically play cards in order to have the lowest scoreAt the end of the round, points are cumulatively totaled for each player.If one player’s total is greater than 100, the game ends and the player with the lowest score wins. 1. How should the following data fields be defined (with respect to final and static)?(a) playerPosition (These have values of North, South, East, or West)(b) directionOfPassing(c) totalScore…arrow_forwardCreate an Employee class. Attributes(must be private): Employee has a name and basic_salary Methods:Employee(string, float); //ConstructorAllowances are computed as: float getHouseRent(); //House rent is 45% of the basic pay. float getMedicalAllowance(); //Medical Allowance is 5% of the basic pay. float getConveyanceAllowance(); //Conveyance allowance is 10% of the basic payfloat getGrossPay(); //Salary including rent and allowances{You can implement additional function/s if needed} Then Create an Organization class. Organization has 10 Employees (Hint: You will need an array of pointers to Employee class) Organization can calculate the total amount to be paid to all employees Organization can print the details(name & salary) of all employees Note: Use main,header and functions.cpp to write code.arrow_forward
- Focus on classes, objects, methods and good programming style Your task is to create a BankAccount class(See the pic attached) The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0. get_pin()should return the pin. check_pin(pin) should check the argument against the saved pin and return True if it matches, False if it does not. deposit(amount) should receive the amount as the argument, add the amount to the account and return the new balance. withraw(amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not. get_balance() should return the current balance. Finally, write a main() to demo your bank account class. Present a menu offering a few actions and perform the action the user…arrow_forwardCar Class Project The car classwill havethe following attributes: •year: an integer that holds the car's model year •model: a string that holds the make of the car •make: a string that holds the model of the car •speed: an integer that holds the car's current speed Your class should contain thefollowing: A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class. •A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string. •To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly. •An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed(). •A modifier method called accelerate() which adds 5 to the speed variable…arrow_forwardCreate a RightTriangle class given the following design: RightTriangle variables: base, height methods: 2 constructors, 1 that set default values and another that accepts to parameters. setBase - changes the base. Requires one parameter for base. setHeight - changes the height. Requires one parameter for height. getBase - returns the triangle base. getHeight - returns the triangle height. area - returns the area of the triangle (1/2bh) given the current base and heightarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
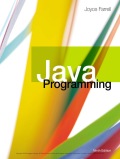
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
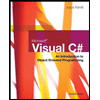
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Memory Management Tutorial in Java | Java Stack vs Heap | Java Training | Edureka; Author: edureka!;https://www.youtube.com/watch?v=fM8yj93X80s;License: Standard YouTube License, CC-BY