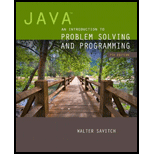
Write a program that will record the votes for one of two candidates by using the class VoteRecorder, which you will design and create. Vote Recorder will have static variables to keep track of the total votes for candidates and instance variables to keep track of the votes made by a single person. It will have the following attributes.
- nameCandidatePresident11—a static String that holds the name of the first candidate for president
- nameCandidatePresident2—a static string that holds the name of the second candidate for president
- nameCandidateVicePresident1 —a static string that holds the name of the first candidate for Vice president
- nameCandidateVicePresident2—a static string that holds the name of the second candidate for Vice president
- votesCondidatePresident1—a static integer that holds the number of votes for the first candidate for president
- votesCandidatePresident2—a static integer that holds the number of voles for the second candidate for president
- votesCandidateVicePresident1—a static integer that holds the number of votes for the first candidate for Vice president
- votesCandidateVicePresident2—a static integer that holds the number of rates for the second candidate for Vice president
- myVoteForPresident—an integer that holds the vote of a single individual for president (0 for no choice, 1 for the first candidate, and 2 for the second candidate)
- myVoteForVicePresident—an integer that holds the vote of a single individual for vice president (0 for no choice, 1 for the first candidate, and 2 for the second candidate)
In addition to appropriate constructors, VotRecorder has the following methods:
- setCandidaterPresident(String name1, String name2)—a static method that sets the names of the names of the two candidates for president
- getCandicateoVicePresident (String name1, string name2)—a static method that sets the names of the two candidates for vice president
- resetVotes—a static method that resets the vote counts to zero
- getCurrentVotePresident—a static method that returns a string with a current total number of votes for both presidential candidates
- getAndConfirmVotes—a non-static method that gets an individual’s votes, confirms then, and then records them
- getAVote(String name1, string name2)—a private method that returns a vote choice for a single race from a individual (0 for no choice, 1 for the first candidate, and 2 for the second candidate)
- getVotes—a private method that returns a vote choice for president and vice president from an individual
- confirmVotes—a private method that displays a person’s vote for president and vice president, asks whether the voter is happy with these choices, and returns true or false according to a yes-or-no response
- recordVotes—a private method that will add an individual’s votes to the appropriate static variables
Create a program that will candidates an election. The candidates for president are Annie and Bob. The candidates for vice president are John and Susan. Use a loop to record the votes of many voters. Create a new Vote Recorder object for each voter. After all the voters are done, present the results.

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Additional Engineering Textbook Solutions
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Mechanics of Materials (10th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Modern Database Management
SURVEY OF OPERATING SYSTEMS
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
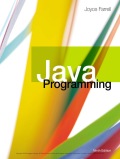
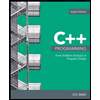
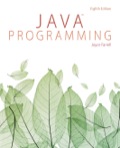
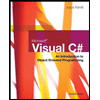