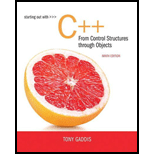
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 5, Problem 45RQE
Write code that does the following: Opens the Numbers.txt file that was created by the code you wrote in Question 44, reads all of the numbers from the file and displays them, then closes the file.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Your answers normally have 50 words. Less than 50 words will not get marks.
1. What is context switch between multiple processes? [2 marks]
2. Draw the memory layout for a C program. [2 marks]
3. How many states does a process has? [2 marks]
4. Compare the non-preemptitve scheduling and preemptive scheduling. [2 marks]
5. Given 4 process and their arrival times and next CPU burst times, what are the average times
and average Turnaround time, for different scheduling algorithms including:
a. First Come, First-Served (FCFS) Scheduling [2 marks]
b. Shortest-Job-First (SJF) Scheduling [2 marks]
c. Shortest-remaining-time-first [2 marks]
d. Priority Scheduling [2 marks]
e. Round Robin (RR) [2 marks]
Process
Arrival Time
Burst Time
P1
0
8
P2
1
9
P3
3
2
P4
5
4
a database with multiple tables from attributes as shown above that are in 3NF,
showing PK, non-key attributes, and FK for each table? Assume the tables are already in
1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]
a database with multiple tables from attributes as shown above that are in 3NF,
showing PK, non-key attributes, and FK for each table? Assume the tables are already in
1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]
Chapter 5 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 5.1 - What will the following program segments display?...Ch. 5.3 - Write an input validation loop that asks the user...Ch. 5.3 - Write an input validation loop that asks the user...Ch. 5.3 - Write an input validation loop that asks the user...Ch. 5.5 - Prob. 5.5CPCh. 5.6 - Name the three expressions that appear inside the...Ch. 5.6 - You want to write a for loop that displays I love...Ch. 5.6 - Prob. 5.8CPCh. 5.6 - Write a for loop that displays your name 10 times.Ch. 5.6 - Write a for loop that displays all of the odd...
Ch. 5.6 - Write a for loop that displays every fifth number,...Ch. 5.8 - Write a for loop that repeats seven times, asking...Ch. 5.8 - In the following program segment, which variable...Ch. 5.8 - Prob. 5.14CPCh. 5.8 - How would you modify Program 5-13 so any negative...Ch. 5.11 - What is an output file? What is an input file?Ch. 5.11 - What three steps must be taken when a file is used...Ch. 5.11 - What is the difference between a text file and a...Ch. 5.11 - Budget Analysis Write a program that asks the user...Ch. 5.11 - Random Number Guessing Game Write a program that...Ch. 5.11 - What type of file stream object do you create if...Ch. 5.11 - Write a short program that uses a for loop to...Ch. 5.11 - Write a short program that opens the file created...Ch. 5 - Why should you indent the statements in the body...Ch. 5 - Describe the difference between pretest loops and...Ch. 5 - Why are the statements in the body of a loop...Ch. 5 - What is the difference between the while loop and...Ch. 5 - Which loop should you use in situations where you...Ch. 5 - Which loop should you use in situations where you...Ch. 5 - Which loop should you use when you know the number...Ch. 5 - Why is it critical that counter variables be...Ch. 5 - Why is it critical that accumulator variables be...Ch. 5 - Why should you be careful not to place a statement...Ch. 5 - What header file do you need to include in a...Ch. 5 - What data type do you use when you want to create...Ch. 5 - What data type do you use when you want to create...Ch. 5 - Why should a program close a file when its...Ch. 5 - What is a files read position? Where is the read...Ch. 5 - To ______ a value means toincreaseit by one,and to...Ch. 5 - Prob. 17RQECh. 5 - Prob. 18RQECh. 5 - The statement or block that is repeated is known...Ch. 5 - Each repetition of a loop is known as a(n)...Ch. 5 - A loop that evaluates its test expression before...Ch. 5 - A loop that evaluates its test expression after...Ch. 5 - A loop that does not have a way of stopping is...Ch. 5 - A(n) _____ is a variablethatcountsthe number of...Ch. 5 - A(n) _____ is a sum of numbers that accumulates...Ch. 5 - A(n) _____ is a variable that is initialized to...Ch. 5 - Prob. 27RQECh. 5 - The _____ loop always iterates at least once.Ch. 5 - The _____ and _____ loops will not iterate at all...Ch. 5 - The _____ loop is ideal for situations that...Ch. 5 - Inside the for loops parentheses, the first...Ch. 5 - A loop that is inside another is called a(n)...Ch. 5 - The ___________ statement causes a loop to...Ch. 5 - The _____ statement causes _____ a loop to skip...Ch. 5 - Write a while loop that lets the user enter a...Ch. 5 - Write a do-while loop that asks the user to enter...Ch. 5 - Write a for loop that displays the following set...Ch. 5 - Write a loop that asks the user to enter a number....Ch. 5 - Write a nested loop that displays 10 rows of #...Ch. 5 - Convert the following while loop to a do-while...Ch. 5 - Convert the following do-while loop to a while...Ch. 5 - Convert the following while loop to a for loop:...Ch. 5 - Convert the following for loop to a while loop:...Ch. 5 - Write code that does the following: Opens an...Ch. 5 - Write code that does the following: Opens the...Ch. 5 - Modify the code that you wrote in Question 45 so...Ch. 5 - T F The operand of the increment and decrement...Ch. 5 - T F The cout statement in the following program...Ch. 5 - T F The cout statement in the following program...Ch. 5 - T F The while loop is a pretest loop.Ch. 5 - T F The do-while loop is a pretest loop.Ch. 5 - T F The for loop is a posttest loop.Ch. 5 - T F It is not necessary to initialize counter...Ch. 5 - T F All three of the for loops expressions may be...Ch. 5 - T F One limitation of the for loop is that only...Ch. 5 - T F Variables may be defined inside the body of a...Ch. 5 - T F A variable may be defined in the...Ch. 5 - T F In a nested loop, the outer loop executes...Ch. 5 - T F In a nested loop, the inner loop goes through...Ch. 5 - T F To calculate the total number of iterations of...Ch. 5 - T F The break statement causes a loop to stop the...Ch. 5 - T F The continue statement causes a terminated...Ch. 5 - T F In a nested loop, the break statement only...Ch. 5 - Prob. 64RQECh. 5 - // Find the error in this program. #include...Ch. 5 - // This program adds two numbers entered by the...Ch. 5 - // This program uses a loop to raise a number to a...Ch. 5 - // This program averages a set of numbers....Ch. 5 - // This program displays the sum of two numbers....Ch. 5 - // This program displays the sum of the numbers...Ch. 5 - Sum of Numbers Write a program that asks the user...Ch. 5 - Characters for the ASCII Codes Write a program...Ch. 5 - Ocean Levels Assuming the oceans level is...Ch. 5 - Calories Burned Running on a particular treadmill...Ch. 5 - Membership Fees Increase A country club, which...Ch. 5 - Distance Traveled The distance a vehicle travels...Ch. 5 - Pennies for Pay Write a program that calculates...Ch. 5 - Math Tutor This program started in Programming...Ch. 5 - Hotel Occupancy Write a program that calculates...Ch. 5 - Average Rainfall Write a program that uses nested...Ch. 5 - Population Write a program that will predict the...Ch. 5 - Celsius to Fahrenheit Table In Programming...Ch. 5 - The Greatest and Least of These Write a program...Ch. 5 - Student Line Up A teacher has asked all her...Ch. 5 - Payroll Report Write a program that displays a...Ch. 5 - Savings Account Balance Write a program that...Ch. 5 - Sales Bar Chart Write a program that asks the user...Ch. 5 - Prob. 18PCCh. 5 - Budget Analysis Write a program that asks the user...Ch. 5 - Random Number Guessing Game Write a program that...Ch. 5 - Random Number Guessing Game Enhancement Enhance...Ch. 5 - Square Display Write a program that asks the user...Ch. 5 - Pattern Displays Write a program that uses a loop...Ch. 5 - Using FilesNumeric Processing If you have...Ch. 5 - Prob. 25PCCh. 5 - Personal Web Page Generator Write a program that...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
This project requires that you complete Programming Project 7 from this chapter and Programming Project 8 from ...
Problem Solving with C++ (10th Edition)
A ______ structure can execute a set of statements only under certain circumstances. a. sequence b. circumstant...
Starting Out with Python (4th Edition)
A cylinder having a mass of 250 kg is to be supported by the cord that wraps over the pipe. Determine the small...
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Distinguish among data definition commands, data manipulation commands, and data control commands.
Modern Database Management
Big data Big data describes datasets with huge volumes that are beyond the ability of typical database manageme...
Management Information Systems: Managing The Digital Firm (16th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? Order, Product, store, customer.arrow_forwardInheritance & Polymorphism (Ch11) There are 6 classes including Person, Student, Employee, Faculty, and Staff. 4. Problem Description: • • Design a class named Person and its two subclasses named student and Employee. • Make Faculty and Staff subclasses of Employee. • A person has a name, address, phone number, and e-mail address. • • • A person has a class status (freshman, sophomore, junior and senior). Define the status as a constant. An employee has an office, salary, and date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString() method in each class to display the class name and the person's name. 4-1. Explain on how you would code this program. (1 point) 4-2. Implement the program. (2 point) 4-3. Explain your code. (2 point)arrow_forward
- Suppose you buy an electronic device that you operate continuously. The device costs you $300 and carries a one-year warranty. The warranty states that if the device fails during its first year of use, you get a new device for no cost, and this new device carries exactly the same warranty. However, if it fails after the first year of use, the warranty is of no value. You plan to use this device for the next six years. Therefore, any time the device fails outside its warranty period, you will pay $300 for another device of the same kind. (We assume the price does not increase during the six-year period.) The time until failure for a device is gamma distributed with parameters α = 2 and β = 0.5. (This implies a mean of one year.) Use @RISK to simulate the six-year period. Include as outputs (1) your total cost, (2) the number of failures during the warranty period, and (3) the number of devices you own during the six-year period. Your expected total cost to the nearest $100 is _________,…arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardPlease answer the JAVA OOP Programming Assignment scenario below: Patriot Ships is a new cruise line company which has a fleet of 10 cruise ships, each with a capacity of 300 passengers. To manage its operations efficiently, the company is looking for a program that can help track its fleet, manage bookings, and calculate revenue for each cruise. Each cruise is tracked by a Cruise Identifier (must be 5 characters long), cruise route (e.g. Miami to Nassau), and ticket price. The program should also track how many tickets have been sold for each cruise. Create an object-oriented solution with a menu that allows a user to select one of the following options: 1. Create Cruise – This option allows a user to create a new cruise by entering all necessary details (Cruise ID, route, ticket price). If the maximum number of cruises has already been created, display an error message. 2. Search Cruise – This option allows to search a cruise by the user provided cruise ID. 3. Remove Cruise – This op…arrow_forward
- I need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.arrow_forwardSouthern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not). The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15. A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots): The average daily utilization of the aircraft (percentage of total flights that fly) The…arrow_forwardWhy is JAVA OOP is really difficult to study?arrow_forward
- My daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forwardQ4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forwarda. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 1 1 0 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
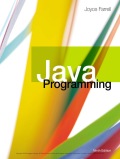
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
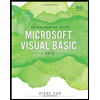
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
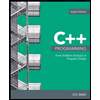
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
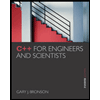
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
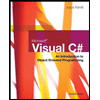
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
C - File I/O; Author: Tutorials Point (India) Ltd.;https://www.youtube.com/watch?v=cEfuwpbGi1k;License: Standard YouTube License, CC-BY
file handling functions in c | fprintf, fscanf, fread, fwrite |; Author: Education 4u;https://www.youtube.com/watch?v=aqeXS1bJihA;License: Standard Youtube License