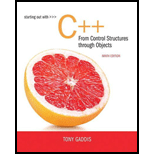
Concept explainers
A)
Analysis of the given code:
The given code is used to print the display statement once. The process is carried out using a “do…while” loop with a “counter” variable initially assigned as “10” and the loop iterates when the value of “counter” is lesser than “1”.
B)
Analysis of the given code:
The given code is used to print the value of “v” variable once. The process is carried out using a “do…while” loop with a “v” variable initially assigned as “10” and the loop iterates when the value of “v” is lesser than “5”.
C)
Analysis of the given code:
The given code is used to print the value of “value” and “count” variable after performing mathematical operations. The process is carried out by defining and declaring the necessary variables and by using a “do…while” loop that iterates to add the value of “number” by “2” and increment the value of the “counter” by “1”, when the value of “count” is less than “limit”. Final process is to display the values of the variables “number” and “count”.

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
- My code is experincing minor issue where the text isn't in the proper place, and to ensure that the frequency cutoff is at the right place. My code: % Define frequency range for the plot f = logspace(1, 5, 500); % Frequency range from 10 Hz to 100 kHz w = 2 * pi * f; % Angular frequency % Parameters for the filters - let's adjust these to get more reasonable cutoffs R = 1e3; % Resistance in ohms (1 kΩ) C = 1e-6; % Capacitance in farads (1 μF) % For bandpass, we need appropriate L value for desired cutoffs L = 0.1; % Inductance in henries - adjusted for better bandpass response % Calculate cutoff frequencies first to verify they're in desired range f_cutoff_RC = 1 / (2 * pi * R * C); f_resonance = 1 / (2 * pi * sqrt(L * C)); Q_factor = (1/R) * sqrt(L/C); f_lower_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) + 1/(2*Q_factor)); f_upper_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) - 1/(2*Q_factor)); % Transfer functions % Low-pass filter (RC) H_low = 1 ./ (1 + 1i * w *…arrow_forwardI would like to know the main features about the following three concepts: 1. Default forwarded 2. WINS Server 3. IP Security (IPSec).arrow_forwardmap the following ER diagram into a relational database schema diagram. you should take into account all the constraints in the ER diagram. Underline the primary key of each relation, and show each foreign key as a directed arrow from the referencing attributes (s) to the referenced relation. NOTE: Need relational database schema diagramarrow_forward
- What is business intelligence? Share the Business intelligence (BI) tools you have used and explain what types of decisions you made.arrow_forwardI need help fixing the minor issue where the text isn't in the proper place, and to ensure that the frequency cutoff is at the right place. My code: % Define frequency range for the plot f = logspace(1, 5, 500); % Frequency range from 10 Hz to 100 kHz w = 2 * pi * f; % Angular frequency % Parameters for the filters - let's adjust these to get more reasonable cutoffs R = 1e3; % Resistance in ohms (1 kΩ) C = 1e-6; % Capacitance in farads (1 μF) % For bandpass, we need appropriate L value for desired cutoffs L = 0.1; % Inductance in henries - adjusted for better bandpass response % Calculate cutoff frequencies first to verify they're in desired range f_cutoff_RC = 1 / (2 * pi * R * C); f_resonance = 1 / (2 * pi * sqrt(L * C)); Q_factor = (1/R) * sqrt(L/C); f_lower_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) + 1/(2*Q_factor)); f_upper_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) - 1/(2*Q_factor)); % Transfer functions % Low-pass filter (RC) H_low = 1 ./ (1 + 1i * w *…arrow_forwardTask 3. i) Compare your results from Tasks 1 and 2. j) Repeat Tasks 1 and 2 for 500 and 5,000 elements. k) Summarize run-time results in the following table: Time/size n String StringBuilder 50 500 5,000arrow_forward
- Can you please solve this without AIarrow_forward1. Create a Vehicle.java file. Implement the public Vehicle and Car classes in Vehicle.java, including all the variables and methods in the UMLS. Vehicle - make: String model: String -year: int + Vehicle(String make, String, model, int, year) + getMake(): String + setMake(String make): void + getModel(): String + setModel(String model): void + getYear(): int + set Year(int year): void +toString(): String Car - numDoors: int + numberOfCar: int + Car(String make, String, model, int, year, int numDoors) + getNumDoors(): int + setNumDoors (int num Doors): void + toString(): String 2. Create a CarTest.java file. Implement a public CarTest class with a main method. In the main method, create one Car object and print the object using System.out.println(). Then, print the numberOfCar. Your printing result must follow the example output: make Toyota, model=Camry, year=2022 numDoors=4 1 Hint: You need to modify the toString methods in the Car class and Vehicle class!arrow_forwardCHATGPT GAVE ME WRONG ANSWER PLEASE HELParrow_forward
- HELP CHAT GPT GAVE ME WRONG ANSWER Consider the following implementation of a container that will be used in a concurrent environment. The container is supposed to be used like an indexed array, but provide thread-safe access to elements. struct concurrent_container { // Assume it’s called for any new instance soon before it’s ever used void concurrent_container() { init_mutex(&lock); } ~concurrent_container() { destroy_mutex(&lock); } // Returns element by its index. int get(int index) { lock.acquire(); if (index < 0 || index >= size) { return -1; } int result = data[index]; lock.release(); return result; } // Sets element by its index. void set(int index, int value) { lock.acquire(); if (index < 0 || index >= size) { resize(size); } data[index] = value; lock.release(); } // Extend maximum capacity of the…arrow_forwardWrite a C program using embedded assembler in which you use your own function to multiply by two without using the product. Tip: Just remember that multiplying by two in binary means shifting the number one place to the left. You can use the sample program from the previous exercise as a basis, which increments a variable. Just replace the INC instruction with SHL.arrow_forwardusing r languagearrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
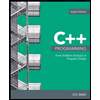
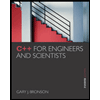
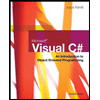
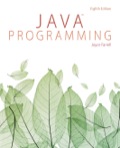
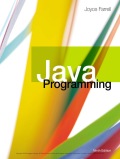