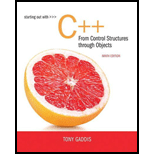
Concept explainers
Savings Account Balance
Write a
A) Ask the user for the amount deposited into the account during the month. (Do not accept negative numbers.) This amount should be added to the balance.
B) Ask the user for the amount withdrawn from the account during the month. (Do not accept negative numbers.) This amount should be subtracted from the balance.
C) Calculate the monthly interest. The monthly interest rate is the annual interest rate divided by 12. Multiply the monthly interest rate by the balance, and add the result to the balance.
After the last iteration, the program should display the ending balance, the total amount of deposits, the total amount of withdrawals, and the total interest earned.

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Modern Database Management
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Concepts Of Programming Languages
Electric Circuits. (11th Edition)
Degarmo's Materials And Processes In Manufacturing
- "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forwardSolve this "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forwardSpecifications: Part-1Part-1: DescriptionIn this part of the lab you will build a single operation ALU. This ALU will implement a bitwise left rotation. Forthis lab assignment you are not allowed to use Digital's Arithmetic components.IF YOU ARE FOUND USING THEM, YOU WILL RECEIVE A ZERO FOR LAB2!The ALU you will be implementing consists of two 4-bit inputs (named inA and inB) and one 4-bit output (named out). Your ALU must rotate the bits in inA by the amount given by inB (i.e. 0-15).Part-1: User InterfaceYou are provided an interface file lab2_part1.dig; start Part-1 from this file.NOTE: You are not permitted to edit the content inside the dotted lines rectangle. Part-1: ExampleIn the figure above, the input values that we have selected to test are inA = {inA_3, inA_2, inA_1, inA_0} = {0, 1, 0,0} and inB = {inB_3, inB_2, inB_1, inB_0} = {0, 0, 1, 0}. Therefore, we must rotate the bus 0100 bitwise left by00102, or 2 in base 10, to get {0, 0, 0, 1}. Please note that a rotation left is…arrow_forwardSolve this "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- Solve this "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forwardSolve this "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
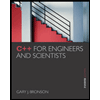
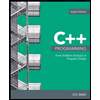
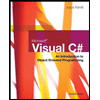
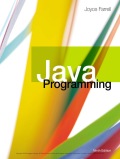
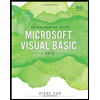