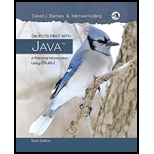
Rewrite getLot so that it does not rely on a lot with a particular number being stored at index (number-1) in the collection. For instance, if lot number 2 has been removed, then lot number 3 will have been moved from index 2 to index 1, and all higher lot numbers will also have been moved by one index position. You may assume that lots are always stored in increasing order according to their lot numbers.

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Programming in C
C++ How to Program (10th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with Java: Early Objects (6th Edition)
- Part 2: Sorting the WorkOrders via dates Another error that will still be showing is that there is not Comparable/compareTo() method setup on the WorkOrder class file. That is something you need to fix and code. Implement the use of the Comparable interface and add the compareTo() method to the WorkOrder class. The compareTo() method will take a little work here. We are going to compare via the date of the work order. The dates of the WorkOrder are saved in a MM-DD-YYYY format. There is a dash '-' in between each part of the date. You will need to split both the current object's date and the date sent through the compareTo() parameters. You will have three things to compare against. You first need to check the year. If the years are the same value then you need to go another step to check the months, otherwise you compare them with less than or greater than and return the corresponding value. If you have to check the months it would be the same for years. If the months are the same you…arrow_forwardImplement a city database using ordered lists. Each database record contains the name of the city (a string of arbitrary length) and the coordinates of the city expressed as integer x and y coordinates. Your database should allow records to be inserted, deleted by name, and searched by name. Another operation that should be supported is to print all records within a given distance of a specified point/coordinate. The order of cities should be alphabetically by city name. Implement the database using: an array- based list implementation. By using JAVA.arrow_forwardWrite a Java application CountryList. In the main method, do the following:1. Create an array list of Strings called countries.2. Add "Canada", "India", "Mexico", "Peru" in that order.3. Use the enhanced for loop to print all countries in the array list, one per line.4. Add "Spain" at index 15. Replace the element at index 2 with "Vietnam". You must use the set method.6. Replace the next to the last element with "Brazil". You must use the set method. You willlose one point if you use 3 in the set method. Do this in a manner that would replace thenext to the last element, no matter the size of the array list.7. Remove the object "Canada" Do not remove at an index. Your code should work if"Canada" was at a different location. There is a version of remove method that willremove a specific object.8. Get and print the first element followed by "***"9. Call method toString() on countries to print all elements on one line.10. Use the enhanced for loop to print all countries in the array list,…arrow_forward
- In this project, you will implement a single linked list structure that represents a list of Tawjihi records and do different operations on the records within that linked list. The inputs for the project will be the two Tawjihi files (One file for West Bank and the other for Gaza records). Each line in those files contains the Tawjihi record of a student (seat number, average, and branch). You may use the code you wrote/implemented during the lectrure to create and store Tawjihi record data into your single Linked List(s). You are free to use as many linked lists as needed. YOU MAY NOT USE ARRAYS IN THIS PROJECT. For good user experience, you will need to implement a user interface. In the user interface there should be a way to select between WestBank or Gaza as well as an option to select between branchs "Literary or Science". According to the previouse selction the following functions will operate in the specfic selected data above: 1. An option to insert new Tawjihi record into the…arrow_forwardThe UnsortedTableMap class is located in the maps folder. In the attachment, you will find a partially implemented class called UnsortedMapOfLists, which extends UnsortedTableMap. The values associated with the keys in UnsortedMapOfLists are arrays. So you have an array with each key. Off course you can save an array in the value of UnsortedTableMap class without the class we are going to develop, but our class makes this easier, as it allows using a syntax like this: myMap["somekey", 1] to reach element at index 1 in the array associated with key "somekey". I already implemented methods __setitem__ and __getitem__. Your task is to: implement method __delitem__ . Deletion will be performed for an element in the array. If index is not given, delete the array associated with the key. implement a function (not a method in the class) to swap the arrays associated with two given keys. Add the required comment in method __getitem__. implement a main to verify that your code is working…arrow_forwardIn this assignment, you will compare the performance of ArrayList and LinkedList. More specifically, your program should measure the time to “get” and “insert” an element in an ArrayList and a LinkedList.You program should 1. Initializei. create an ArrayList of Integers and populate it with 100,000 random numbersii. create a LinkedList of Integers and populate it with 100,000 random numbers2. Measure and print the total time it takes to i. get 100,000 numbers at random positions from the ArrayList 3. Measure and print the total time it takes to i. get 100,000 numbers at random positions from the LinkedList 4. Measure and print the total time it takes to i. insert 100,000 numbers in the beginning of the ArrayList 5. Measure and print the total time it takes to i. insert 100,000 numbers in the beginning of the LinkedList 6. You must print the time in milliseconds (1 millisecond is 1/1000000 second).A sample run will be like this:Time for get in ArrayList(ms): 1Time for get in…arrow_forward
- 1. The codewords will be scrambled words. You will read the words used for the codes from a file and store it into an array. There are 60 words in the file that range in size from 3 characters to 7 characters. The file is called wordlist.txt and can be found attached to the assignment in IvyLearn.2. Start with input for an integer seed for the random number generator. There is no need to put a prompt before the cin operation.3. Ask the Player if they are ready to play and only proceed if they type a Y or y. If the user types an N or n then the program should finish. a. INPUT VALIDATION: Make sure the user has typed either y, Y, n, or N4. Create a variable to keep track of the number of guesses the user has made.5. Use the random number generator to pick a word from the list of words.6. Once you have chosen a word as the codeword you will need to scramble the letters to make it into a code. You should use the random number generator to help you mix up the letters.7. Display to the user…arrow_forwardA for construct is used to build a loop that processes a list of elements in programming. To do this, it continues to operate forever as long as there are objects to process. Is this assertion truthful or false? Explain your response.arrow_forwardIn this programming challenge, you will create a simple trivia game for two players. The program will work like this: • Read the contents of the trivia.txt file into an ArrayList . • Starting with player 1, each player gets a turn at answering one trivia question. (For instance, if there are 10 questions, 5 for each player.) When a question is displayed, four possible answers are also displayed. Only one of the answers is correct, and if the player selects the correct answer, he or she earns a point. • After answers have been selected for all of the questions, the program displays the number of points earned by each player and declares the player with the highest number of points the winner, same as the sample execution. You are to design a Question class to hold the data for a trivia question. The Question class should have String fields for the following data: • A trivia question • Possible answer 1 • Possible answer 2 • Possible answer 3 • Possible answer 4 • The number of the…arrow_forward
- Add methods to the STUDENT class that compare two STUDENT objects. One method should test for equality. The other methods should support the other possible comparisons. In each case, the method returns the result of the comparison of the two students' names. Place several STUDENT objects into a list and shuffle it. Then run the SORT method with this list and display all of the students' information.arrow_forwardTASK 3 Using one of the sorting methods, sort the Car ArrayList based on their ‘City mpg’ feature. Justify your choice of the algorithm used. HINTS: 1. You need to create a class called Car that implements the Comparable interface and load the data from the file to a list. Then somehow convert the arraylist to an array to use the implementations of the algorithms 2. To measure the time it took to run, you can use System.nanoTime() or System.currentTimeMillisbefore and after the method is executed (see here: https://docs.oracle.com/javase/7/docs/api/java/lang/System.html) and subtract. Deliverables: 1. A IntelliJ project implementing the tasks 2. A report on the second exercise showing a graph of running time vs. input size of both algorithms and your conclusions.arrow_forwardThe code given below represents a saveTransaction() method which is used to save data to a database from the Java program. Given the classes in the image as well as an image of the screen which will call the function, modify the given code so that it loops through the items again, this time as it loops through you are to insert the data into the salesdetails table, note that the SalesNumber from the AUTO-INCREMENT field from above is to be inserted here with each record being placed into the salesdetails table. Finally, as you loop through the items the product table must be update because as products are sold the onhand field in the products table must be updated. When multiple tables are to be updated with related data, you should wrap it into a DMBS transaction. The schema for the database is also depicted. public class PosDAO { private Connection connection; public PosDAO(Connection connection) { this.connection = connection; } public void…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
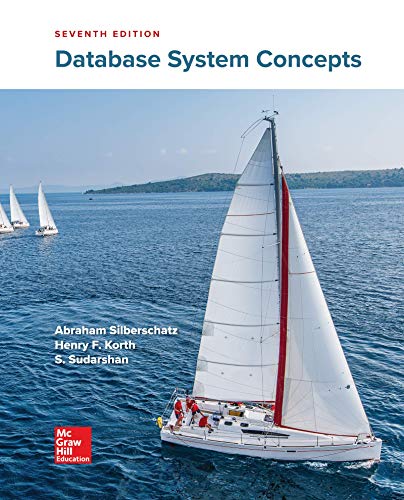
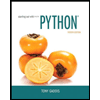
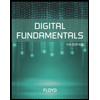
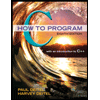
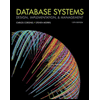
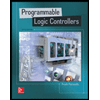