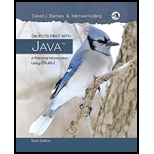
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
6th Edition
ISBN: 9780134477367
Author: David J. Barnes, Michael Kolling
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 4, Problem 54E
Program Plan Intro
To define a method in the Club class.
Write a program in BlueJ to define a method in the Club class named:
public int (joinedlnMonthfint month)
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 4 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Ch. 4 - Prob. 1ECh. 4 - What happens if you create a new MusicOrganizer...Ch. 4 - Prob. 3ECh. 4 - Prob. 4ECh. 4 - Write a declaration of a local variable called...Ch. 4 - Prob. 6ECh. 4 - Write assignments to the library, cs101. and track...Ch. 4 - If a collection stores 10 objects, what value...Ch. 4 - Write a method call using get to return the fifth...Ch. 4 - Prob. 10E
Ch. 4 - Write a method call to add the object held in the...Ch. 4 - Write a method call to remove the third object...Ch. 4 - Suppose that an object is stored at index 6 in a...Ch. 4 - Add a method called checklndex to the...Ch. 4 - Write an alternative version of checkIndex called...Ch. 4 - Rewrite both the 1istFi1e and removeFi1e methods...Ch. 4 - Prob. 17ECh. 4 - Prob. 18ECh. 4 - We know that the first file name is stored at...Ch. 4 - Prob. 20ECh. 4 - Create a MusicOrganizer and store a few file names...Ch. 4 - Create an ArrayList<String> in the Code Pad by...Ch. 4 - If you wish, you could use the debugger to help...Ch. 4 - Challenge exercise The for-each loop does not use...Ch. 4 - Prob. 25ECh. 4 - Prob. 26ECh. 4 - Prob. 27ECh. 4 - Write out the header of a for-each loop to process...Ch. 4 - Suppose we express the first version of the key...Ch. 4 - Write a while loop (for example, in a method...Ch. 4 - Write a while loop to add up the values 1 to 10...Ch. 4 - Write a method called sum with a while loop that...Ch. 4 - Challenge exercise Write a method isPrime (int n)...Ch. 4 - In the findFirst method, the loop's condition...Ch. 4 - Prob. 35ECh. 4 - Have the MusicOrganizer increment the play count...Ch. 4 - Prob. 37ECh. 4 - Prob. 38ECh. 4 - Prob. 39ECh. 4 - Prob. 40ECh. 4 - Complete the numberOfMembers method to return the...Ch. 4 - Prob. 42ECh. 4 - Prob. 43ECh. 4 - Prob. 44ECh. 4 - Challenge exercise Write a method to play every...Ch. 4 - Prob. 46ECh. 4 - Prob. 47ECh. 4 - Add a close method to the Auction class. This...Ch. 4 - Add a getUnsold method to the Auction class with...Ch. 4 - Suppose the Auction class includes a method that...Ch. 4 - Rewrite getLot so that it does not rely on a lot...Ch. 4 - Prob. 52ECh. 4 - Prob. 53ECh. 4 - Prob. 54ECh. 4 - Prob. 55ECh. 4 - Open the products project and complete the...Ch. 4 - Implement the findProduct method. This should look...Ch. 4 - Implement the numberInStock method. This should...Ch. 4 - Prob. 59ECh. 4 - Challenge exercise Implement a method in...Ch. 4 - Java provides another type of loop: the do-while...Ch. 4 - Prob. 85ECh. 4 - Prob. 86ECh. 4 - Find out about Java's switch-case statement. What...
Knowledge Booster
Similar questions
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
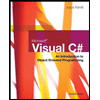
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
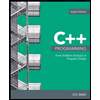
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
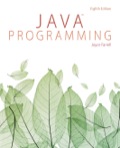
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
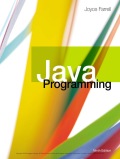
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT