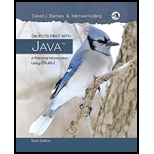
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
6th Edition
ISBN: 9780134477367
Author: David J. Barnes, Michael Kolling
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 4, Problem 12E
Write a method call to remove the third object stored in a collection called dates.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
What should the next three steps be in my machine based home security system after deployment and after the following current steps:
Enhancing Security & Privacy Measures
User Interface (UI) and Experience (UX) Improvement
Machine Learning Model Refinement & Continuous Improvement
I am creating a machine learning home based security system, have completed initial deployment and in the following phases of the project: Expanding device compatibility and integration, preparing for cloud integration, and implementing system reduncancy and disaster recovery. What should the next three phases be?
Hands-On Assignments Part II
Assignment 1-5: Querying the DoGood Donor Database
Review the DoGood Donor data by writing and running SQL statements to perform the following
tasks:
1. List each donor who has made a pledge and indicated a single lump sum payment. Include
first name, last name, pledge date, and pledge amount.
2. List each donor who has made a pledge and indicated monthly payments over one year.
Include first name, last name, pledge date, and pledge amount. Also, display the monthly
payment amount. (Equal monthly payments are made for all pledges paid in monthly
payments.)
3. Display an unduplicated list of projects (ID and name) that have pledges committed. Don't
display all projects defined; list only those that have pledges assigned.
4. Display the number of pledges made by each donor. Include the donor ID, first name, last
name, and number of pledges.
5. Display all pledges made before March 8, 2012. Include all column data from the
DD PLEDGE table.
Chapter 4 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Ch. 4 - Prob. 1ECh. 4 - What happens if you create a new MusicOrganizer...Ch. 4 - Prob. 3ECh. 4 - Prob. 4ECh. 4 - Write a declaration of a local variable called...Ch. 4 - Prob. 6ECh. 4 - Write assignments to the library, cs101. and track...Ch. 4 - If a collection stores 10 objects, what value...Ch. 4 - Write a method call using get to return the fifth...Ch. 4 - Prob. 10E
Ch. 4 - Write a method call to add the object held in the...Ch. 4 - Write a method call to remove the third object...Ch. 4 - Suppose that an object is stored at index 6 in a...Ch. 4 - Add a method called checklndex to the...Ch. 4 - Write an alternative version of checkIndex called...Ch. 4 - Rewrite both the 1istFi1e and removeFi1e methods...Ch. 4 - Prob. 17ECh. 4 - Prob. 18ECh. 4 - We know that the first file name is stored at...Ch. 4 - Prob. 20ECh. 4 - Create a MusicOrganizer and store a few file names...Ch. 4 - Create an ArrayList<String> in the Code Pad by...Ch. 4 - If you wish, you could use the debugger to help...Ch. 4 - Challenge exercise The for-each loop does not use...Ch. 4 - Prob. 25ECh. 4 - Prob. 26ECh. 4 - Prob. 27ECh. 4 - Write out the header of a for-each loop to process...Ch. 4 - Suppose we express the first version of the key...Ch. 4 - Write a while loop (for example, in a method...Ch. 4 - Write a while loop to add up the values 1 to 10...Ch. 4 - Write a method called sum with a while loop that...Ch. 4 - Challenge exercise Write a method isPrime (int n)...Ch. 4 - In the findFirst method, the loop's condition...Ch. 4 - Prob. 35ECh. 4 - Have the MusicOrganizer increment the play count...Ch. 4 - Prob. 37ECh. 4 - Prob. 38ECh. 4 - Prob. 39ECh. 4 - Prob. 40ECh. 4 - Complete the numberOfMembers method to return the...Ch. 4 - Prob. 42ECh. 4 - Prob. 43ECh. 4 - Prob. 44ECh. 4 - Challenge exercise Write a method to play every...Ch. 4 - Prob. 46ECh. 4 - Prob. 47ECh. 4 - Add a close method to the Auction class. This...Ch. 4 - Add a getUnsold method to the Auction class with...Ch. 4 - Suppose the Auction class includes a method that...Ch. 4 - Rewrite getLot so that it does not rely on a lot...Ch. 4 - Prob. 52ECh. 4 - Prob. 53ECh. 4 - Prob. 54ECh. 4 - Prob. 55ECh. 4 - Open the products project and complete the...Ch. 4 - Implement the findProduct method. This should look...Ch. 4 - Implement the numberInStock method. This should...Ch. 4 - Prob. 59ECh. 4 - Challenge exercise Implement a method in...Ch. 4 - Java provides another type of loop: the do-while...Ch. 4 - Prob. 85ECh. 4 - Prob. 86ECh. 4 - Find out about Java's switch-case statement. What...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
What is the difference between a process that is ready and a process that is waiting?
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Write a statement or statements that can be used in a Java program to display the following on the screen: Java...
Java: An Introduction to Problem Solving and Programming (8th Edition)
By doing this, you can hide a classs attribute from code outside the class. a. avoid using the self parameter t...
Starting Out with Python (4th Edition)
Use the following tables for your answers to questions 3.7 through 3.51 : PET_OWNER (OwnerID, OwnerLasst Name, ...
Database Concepts (8th Edition)
A picture is taken of a man performing a pole vault, and the minimum radius of curvature of the pole is estimat...
Mechanics of Materials (10th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a FancyCar class to support basic operations such as drive, add gas, honk horn, and start engine. FancyCar.java is provided with method stubs. Follow each step to gradually complete all methods. Note: This program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. The main() method includes basic method calls. Add statements in main() as methods are completed to support development mode testing. Step 0. Declare private fields for miles driven as shown on the odometer (int), gallons of gas in tank (double), miles per gallon or MPG (double), driving capacity (double), and car model (String). Note the provided final variable indicates the gas tank capacity of 14.0 gallons. Step 1 (2 pts). 1) Complete the default constructor by initializing the odometer to five miles, tank is full of gas, miles per gallon is 24.0, and the model is "Old Clunker". 2)…arrow_forwardFind the error: daily_sales = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(7): daily_sales[i] = float(input('Enter the sales for ' \ + day_of_week[i] + ': ')arrow_forwardFind the error: daily_sales = [0.0, 0,0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(7): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': ')arrow_forward
- Find the error: daily_sales = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(6): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': '))arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it and normalize it? Give two references with your answer.arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Consider that the database offline is not allowed since people are connected to it and personal data might be bridged and not secured. Provide three refernces with you answer.arrow_forward
- Should software manufacturers should be tolerant of the practice of software piracy in third-world countries to allow these countries an opportunity to move more quickly into the information age? Why or why not?arrow_forwardI would like to know about the features of Advanced Threat Protection (ATP), AMD-V, and domain name space (DNS).arrow_forwardPlease show the code for the Tikz figurearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
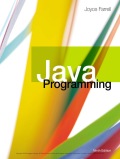
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
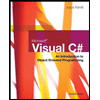
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
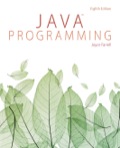
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
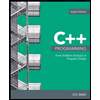
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
Java Math Library; Author: Alex Lee;https://www.youtube.com/watch?v=ufegX5o8uc4;License: Standard YouTube License, CC-BY