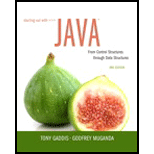
Concept explainers
Explanation of Solution
Complete executable code:
Filename: “Node.java”
Refer “Node.java” from chapter 19 in the textbook
Filename: “Example1LinkedList.java”
//Define "Example1LinkedList" class
public class Example1LinkedList
{
//Define main function
public static void main(String[] args)
{
/* Create a linked list with two string values */
Node colorList = new Node("Yellow", new Node("Purple"));
/* Add a string value to the beginning of the list */
colorList = new Node("Brown", colorList);
/* Display a first string value of "colorList" linked list */
System.out.print(colorList.value);
/* Display a next string value of "colorList" linked list */
System.out.print(colorList.next.value);
/* Display a another next string value of "colorList" linked list */
System.out.print(colorList.next.next.value);
System.out...

Want to see the full answer?
Check out a sample textbook solution
Chapter 20 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- import java.util.Scanner; class InventoryNode { private String item; private int numberOfItems; private InventoryNode nextNodeRef; // Reference to the next node public InventoryNode() { item = ""; numberOfItems = 0; nextNodeRef = null; } public InventoryNode(String itemInit, int numberOfItemsInit) { this.item = itemInit; this.numberOfItems = numberOfItemsInit; this.nextNodeRef = null; } public InventoryNode(String itemInit, int numberOfItemsInit, InventoryNode nextLoc) { this.item = itemInit; this.numberOfItems = numberOfItemsInit; this.nextNodeRef = nextLoc; } // Getter for item public String getItem() { return item; } // Getter for numberOfItems public int getNumberOfItems() { return numberOfItems; } // Getter for nextNodeRef public InventoryNode getNext() { return this.nextNodeRef; } // Setter for nextNodeRef public void…arrow_forward7. What is output by the following code section? QueueInterface aQueue = new QueueReference Based(); int numl, num2; for (int i = 1; i <= 5; i++) { aQueue.enqueue(i); } // end for for (int i = 1; i <= 5; i++) { numl = (Integer) aQueue.dequeue (); num2 (Integer) aQueue.dequeue (); aQueue.enqueue (numl + num2); aQueue.enqueue (num2 - numl); } // end for while (!aQueue.isEmpty()) { System.out.print (aQueue.dequeue () + } // end forarrow_forwardstarter code: in java pls and thank you! public class LinkedList { private Node head; private Node tail; public void add(String item) { Node newItem = new Node(item); // handles the case where the new item // is the only thing in the list if (head == null) { head = newItem; tail = newItem; return; } tail.next = newItem; tail = newItem; } public void print() { Node current = head; while (current != null) { System.out.println(current.item); current = current.next; } } public void printWithSkips() { // TODO your code here } class Node { String item; Node next; public Node(String item) { this.item = item; this.next = null; } } } and public class Driver { public static void…arrow_forward
- write this code below as algorithim to determine the leaf node reclusively ? public static void printLeafNodes(TreeNode node) { // base case if (node == null) { return; } if (node.left == null && node.right == null) { System.out.printf("%d ", node.value); } printLeafNodes(node.left); printLeafNodes(node.right); }arrow_forwardFor any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forwardExplain the following code : public static void printLeafNodes(TreeNode node) { // base case if (node == null) { return; } if (node.left == null && node.right == null) { System.out.printf("%d ", node.value); } printLeafNodes(node.left); printLeafNodes(node.right); }arrow_forward
- Public class DLL{ public DLLNode first; public DLLNode last; public DLL() {this.first=Null; this.last=Null;} Public void Display() {if (first==Null) {s.o.p(“ the list is empty”);} else {for (DLLNode curr=first; curr!=Null; curr=curr.succ) {System.out.println(“the element is”+ curr.element); }} Public void AddBegining(DLLNode Node) {Node.succ=first; First.prev=Node First=Node; Node.prev=Null; if(first ==null && last==null) Last=node; } Public void Append(DLLNode Node) {Node.succ=Null; if(first==Null) {first=Node; Node.prev=Null} Public void insetAfter(int obj, DLLNode Node) {DLLNode curr=first; while((curr!=Null)&&( curr.element!=obj)) { curr=curr.succ; } If(curr.element==obj) {Node.succ=curr.succ; Curr.succ.prev=Node; Curr.succ=Node; Node.prev=curr;} Else System.out.println(“the element doesn’t exist”); Public void delete(DLLNode del) { if(first==Null) {System.out.println(“the list is empty”); } Else if(del==first)…arrow_forwardclass WaterSort { Character top = null; // create constants for colors static Character red= new Character('r'); static Character blue = new Character('b'); static Character yellow= new Character('g'); // Bottles declaration public static void showAll( StackAsMyArrayList bottles[]) { for (int i = 0; i<=4; i++) { System.out.println("Bottle "+ i+ ": " + bottles[i]); } } public static void main(String args[]) { //part 1 //create the bottle StackAsMyArrayList bottleONE = new StackAsMyArrayList<Character>(); System.out.println("\nbottleONE:"+ bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());…arrow_forwardWhat is the running time for this code ?arrow_forward
- C++arrow_forwardmkfifo("mypipe"); if (!fork()) { fp = popen("echo 37 > mypipe", "w"); %D status = pclose(fp); } else { printf("14"); sleep(1); fp = popen("cat mypipe", "r"); fgets(buffer, buffer_size, fp); printf("%s", buffer); status = pclose(fp); %3D } Consider the above code fragment, what's the output to stdout?arrow_forward#include <iostream>#include <cstdlib>using namespace std; class IntNode {public: IntNode(int dataInit = 0, IntNode* nextLoc = nullptr); void InsertAfter(IntNode* nodeLoc); IntNode* GetNext(); void PrintNodeData(); int GetDataVal();private: int dataVal; IntNode* nextNodePtr;}; // ConstructorIntNode::IntNode(int dataInit, IntNode* nextLoc) { this->dataVal = dataInit; this->nextNodePtr = nextLoc;} /* Insert node after this node. * Before: this -- next * After: this -- node -- next */void IntNode::InsertAfter(IntNode* nodeLoc) { IntNode* tmpNext = nullptr; tmpNext = this->nextNodePtr; // Remember next this->nextNodePtr = nodeLoc; // this -- node -- ? nodeLoc->nextNodePtr = tmpNext; // this -- node -- next} // Print dataValvoid IntNode::PrintNodeData() { cout << this->dataVal << endl;} // Grab location pointed by nextNodePtrIntNode* IntNode::GetNext() { return this->nextNodePtr;} int IntNode::GetDataVal() {…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
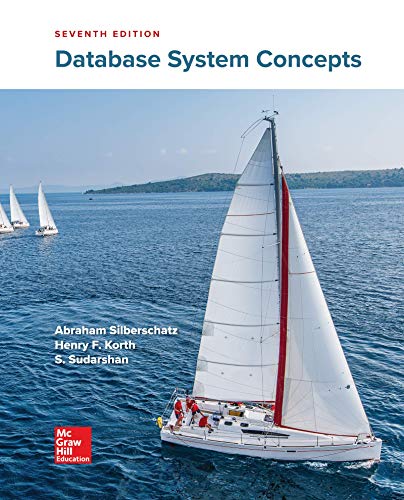
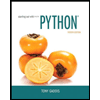
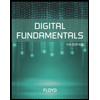
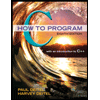
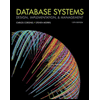
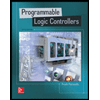