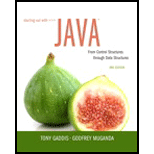
Concept explainers
Explanation of Solution
Purpose of the given code:
The given code is used to remove the last node from a nonempty doubly linked list “myList”.
Given code:
/* The reference last points to the last node in a nonempty doubly linked list. Remove the last node from the list */
last = last.prev;
Error in the given code:
First, user needs to check if the list is nonempty, and also have to undo the removed node, and then set the next reference of the new last node to null.
/* If the last node is equal to "null", then */
if (last == null)
/* Set "first" to "null" */
first = null;
//Otherwise
else
{
/* Set the reference "prev" of last node to "null" */
last.next.prev = null;
/* Set next node of last to "null" */
last.next = null;
}
Corrected code:
/* The reference last points to the last node in a nonempty doubly linked list...

Want to see the full answer?
Check out a sample textbook solution
Chapter 20 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- What is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forward
- Determine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward• Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forward
- Write .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward• Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forward
- Question No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forwardData communıcatıon digital data is transmitted via analog ASK and PSK are used together to increase the number of bits transmitted a)For m=8,suggest a solution and define signal elements , and then draw signals for the following sent data data = 0 1 0 1 1 0 0 0 1 0 1 1arrow_forwardDatacommunicationData = 1 1 0 0 1 0 0 1 0 1 1 1 1 0 0a) how many bıts can be detected and corrected by this coding why prove?b)what wıll be the decision of the reciever if it recieve the following codewords why?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
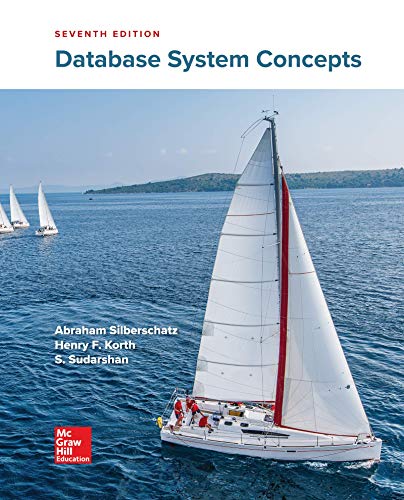
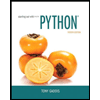
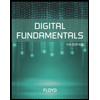
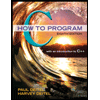
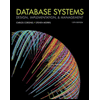
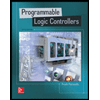