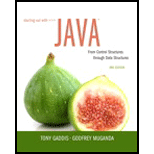
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 20, Problem 5MC
Program Description Answer
When remove a node that is not the head of a singly linked list, user need to contain a reference to the node that precedes it.
Hence, the correct answer is option “A”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7).
A code breaker manages to factories 247 = 13 x 19
Determine Alice's secret key.
To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.
Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.
Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnn
Chapter 20 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 20.1 - Prob. 20.1CPCh. 20.1 - Prob. 20.2CPCh. 20.3 - Prob. 20.4CPCh. 20 - A list is a collection that _____. a. associates...Ch. 20 - Prob. 2MCCh. 20 - Prob. 3MCCh. 20 - Prob. 4MCCh. 20 - Prob. 5MCCh. 20 - Prob. 6MCCh. 20 - Prob. 7MC
Ch. 20 - Prob. 11TFCh. 20 - Prob. 12TFCh. 20 - Prob. 13TFCh. 20 - Prob. 14TFCh. 20 - Prob. 15TFCh. 20 - Prob. 16TFCh. 20 - Prob. 17TFCh. 20 - Prob. 18TFCh. 20 - Prob. 29TFCh. 20 - Prob. 20TFCh. 20 - Prob. 1FTECh. 20 - Prob. 2FTECh. 20 - Prob. 3FTECh. 20 - Prob. 4FTECh. 20 - Prob. 5FTECh. 20 - Prob. 1AWCh. 20 - Prob. 2AWCh. 20 - Prob. 3AWCh. 20 - Prob. 4AWCh. 20 - Prob. 3SACh. 20 - Prob. 4SACh. 20 - Prob. 5SACh. 20 - Consult the online Java documentation and...Ch. 20 - Prob. 1PCCh. 20 - Prob. 2PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
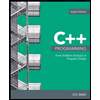
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning