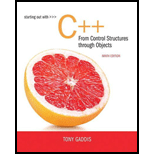
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 20, Problem 9PC
Program Plan Intro
String Reverser
Program Plan:
- Include the required header files.
- Declare the necessary function prototype and constants.
- Define the main () function.
- Declare the necessary variables.
- Get the required input from the user.
- Call the method “reversestring()” to reverse the string.
- Display the string after reversing.
- Define the “reversestring ()” method,
- Condition that validates the length of the string, if the length of the string is less than “0” it gets returned as an empty string.
- If the length of the string is greater than “0”, then given string gets reversed and will be displayed.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Recursive PrintingDesign a recursive function that accepts an integer argument,n , and prints the numbers 1 up through n .
1. Recursive Multiplication
Write a recursive function that accepts two arguments into the parameters x and y. The
function should return the value of x times y. Remember, multiplication can be performed
as repeated addition as follows:
7* 4 = 4 + 4 + 4 +4 + 4 + 4 + 4
PythonDesign a recursive function that accepts an integer argument, n, and prints the number 1 up through n.
Chapter 20 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 20.2 - What happens if a recursive function never...Ch. 20.2 - What is a recursive functions base case?Ch. 20.2 - Prob. 20.3CPCh. 20.2 - What is the difference between direct and indirect...Ch. 20 - What is the base case of each of the recursive...Ch. 20 - What type of recursive function do you think would...Ch. 20 - Which repetition approach is less efficient, a...Ch. 20 - When should you choose a recursive algorithm over...Ch. 20 - Explain what is likely to happen when a recursive...Ch. 20 - The _____________ of recursion is the number of...
Ch. 20 - Prob. 7RQECh. 20 - Prob. 8RQECh. 20 - Prob. 9RQECh. 20 - Write a recursive function to return the number of...Ch. 20 - Write a recursive function to return the largest...Ch. 20 - #include iostream using namespace std; int...Ch. 20 - Prob. 13RQECh. 20 - #include iostream #include string using namespace...Ch. 20 - Iterative Factorial Write an iterative version...Ch. 20 - Prob. 2PCCh. 20 - Prob. 3PCCh. 20 - Recursive Array Sum Write a function that accepts...Ch. 20 - Prob. 5PCCh. 20 - Prob. 6PCCh. 20 - Prob. 7PCCh. 20 - Prob. 8PCCh. 20 - Prob. 9PCCh. 20 - Prob. 10PCCh. 20 - Prob. 11PCCh. 20 - Ackermanns Function Ackermanns Function is a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Exponent y Catherine Arellano mplement a recursive function that returns he exponent given the base and the result. for example, if the base is 2 and the result is 3, then the output should be 3 because the exponent needed for 2 to become 8 is 3 (i.e. 23 = 8) nstructions: 1. In the code editor, you are provided with a main() function that asks the user for two integer inputs: 1. The first integer is the base 2. The second integer is the result 2. Furthermore, you are provided with the getExponent() function. The details of this function are the following: 1. Return type - int 2. Name - getExponent 3. Parameters 1. int - base 2. int - result 4. Description - this recursive function returns the exponent 5. Your task is to add the base case and the general case so it will work Score: 0/5 Overview 1080 main.c exponent.h 1 #include 2 #include "exponent.h" 3 int main(void) { 4 int base, result; 5 6 printf("Enter the base: "); scanf("%d", &base); 7 8 9 printf("Enter the result: ");…arrow_forwardRecursive functions are ones that repeat themselves repeatedly.arrow_forwardRecursive Power FunctionWrite a function that uses recursion to raise a number to a power. The function should accept two arguments: the number to be raised and the exponent. Assume that the exponent is a nonnegative integer. Demonstrate the function in a program. SAMPLE RUN #0: ./recursiveExponent Hide Invisibles Highlight: Show Highlighted Only 2^3=8↵ 2^4=16↵ 3^3=27↵ 6^3=216↵ 7^7=823543↵ 10^9=1000000000↵arrow_forward
- *C Language The greatest common divisor of integers x and y is the largest integer that divides both x and y. Write a recursive function GCD that returns the greatest common divisor of x and y. The GCD of x and y is defined as follows: If y is equal to zero, then GCD(x, y) is x; otherwise GCD(x, y) is GCD(y, x % y) where % is the remainder operator.arrow_forward7. Recursive Power Method In Python, design a function that uses recursion to raise a number to a power. The function should accept two arguments: the number to be raised, and the exponent. Assume the exponent is a nonnegative integer.arrow_forwardCodeWorkout Gym Course Search exercises... Q Search kola shreya@columbus X275: Recursion Programming Exercise: Check Palindrome X275: Recursion Programming Exercise: Check Palindrome Write a recursive function named checkPalindrome that takes a string as input, and returns true if the string is a palindrome and false if it is not a palindrome. A string is a palindrome if it reads the same forwards or backwards. Recall that str.charAt(a) will return the character at position a in str. str.substring(a) will return the substring of str from position a to the end of str,while str.substring(a, b) will return the substring of str starting at position a and continuing to (but not including) the character at position b. Examples: checkPalindrome ("madam") -> true Your Answer: 1 public boolean checkPalindrome (String s) { 4 CodeWorkout © Virginia Tech About License Privacy Contactarrow_forward
- 2. Sum: a recursive function that computes the sum of integers 1, 2, 3, …., n for a given number n. So Sum(6) should return 1 + 2 + 3 + 4 + 5 + 6 , i.e. 21.sum(n) = n + sum(n-1)arrow_forwardPYTHON RECURSIVE FUNCTION Write a python program that lists all ways people can line up for a photo (all permutations of a list of strings). The program will read a list of one word names, then use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Juliaarrow_forwardWrite a recursive function that converts a decimal number into a hex number as a string. The function header is: string decimalToHex(int value) Write a test program that prompts the user to enter a decimal number and displays its hex equivalent.arrow_forward
- Write a recursive function that converts a decimal number into a binary number as a string. The function header is: string decimalToBinary(int value) Write a test program that prompts the user to enter a decimal number and dis- plays its binary equivalent.arrow_forwardComputer science C prog Create a recursive function that finds if a number is palindrome or not(return true or false), A palindromic number is a number (such as 16461) that remains the same when its digits are reversed. In the main function asks the user to enter a number then check if it's palindrome or not using the function you created previously.arrow_forwardC PROGRAMarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
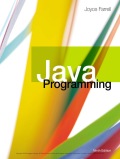
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
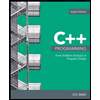
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning