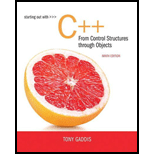
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 20, Problem 10PC
Program Plan Intro
MaxNode function
Program Plan:
“Main.cpp”:
- Include the required header files.
- Declare the necessary function prototype and constants.
- Define the main () function.
- Declare the necessary variables.
- Insert the values into the list.
- Displays to user that the values are inserted.
- Call the method “maximumnode()” to find the largest element of the list.
- The largest valued node gets displayed.
“NumberList.h”:
- Define the template function necessary.
- Define the class “NumberList”.
- Declare the necessary structure variables.
- Define the constructor and destructor.
- Declare the necessary function prototype.
“NumberList.cpp”:
- Include the required header files.
- Declare the necessary function prototype and constants.
- Define the method named “nodeappend()”,
- Declare the necessary variables
- Validate the list using if statement to find the status of the list.
- Use loop to iterate for the contents present in the list and add node to the last of the list.
- Define the method named “viewlist()”,
- Declare the necessary variables.
- Use loop that iterates to display the elements present in the list.
- Define the method named “insertval()”,
- Declare the necessary variables.
- Validate the content of the list using if statement.
- After evaluation of the list the contents the values are inserted at the desired location.
- Loop is used to iterate for the values that are present, to validate whether the particular element is greater or lesser than the elements present in the list.
- After evaluation the value is inserted at the desired location.
- Define the method named “removenode()”,
- Declare the necessary variables.
- Validate the list using if statement in order to position the list based on the elements that is deleted from the list.
- Define the method named “~NumberList()”,
- Declare the necessary variables.
- Loop that iterates to delete the memory allocates.
- Define the method named “sortlist()”,
- Declare the necessary variables.
- Use loop to iterate for the value of the list.
- A condition statement to compare the elements of the list and return the sorted list.
- Define the method named “mergeval()”,
- Loop that is used to iterate to merge the values of the list based on the list values.
- Call the function “sortlist()” to sort the value after being merged.
- Define the method named “maximumnode()”,
- Return the head node to be the maximum node.
- Defined the method named “maxNode()”,
- Use a condition statement to validate the elements of the list to identify the maximum value that is present in the list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
6. What is Race condition? How to prevent it? [2 marks]
7. How many synchronization methods do you know and compare the differences. [2 marks]
8. Explain what are the “mutual exclusion”, “deadlock”, “livelock”, and “eventual entry”, with
the traffic intersection as an example like dinning philosophy. [2 marks]
9. For memory allocation, what are the difference between internal fragmentation and external
fragmentation. Explain with an example. [2 marks]
10. How can the virtual memory map to the physical memory. Explain with an example. [2
marks]
Your answers normally have 50 words. Less than 50 words will not get marks.
1. What is context switch between multiple processes? [2 marks]
2. Draw the memory layout for a C program. [2 marks]
3. How many states does a process has? [2 marks]
4. Compare the non-preemptitve scheduling and preemptive scheduling. [2 marks]
5. Given 4 process and their arrival times and next CPU burst times, what are the average times
and average Turnaround time, for different scheduling algorithms including:
a. First Come, First-Served (FCFS) Scheduling [2 marks]
b. Shortest-Job-First (SJF) Scheduling [2 marks]
c. Shortest-remaining-time-first [2 marks]
d. Priority Scheduling [2 marks]
e. Round Robin (RR) [2 marks]
Process
Arrival Time
Burst Time
P1
0
8
P2
1
9
P3
3
2
P4
5
4
a database with multiple tables from attributes as shown above that are in 3NF,
showing PK, non-key attributes, and FK for each table? Assume the tables are already in
1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]
Chapter 20 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 20.2 - What happens if a recursive function never...Ch. 20.2 - What is a recursive functions base case?Ch. 20.2 - Prob. 20.3CPCh. 20.2 - What is the difference between direct and indirect...Ch. 20 - What is the base case of each of the recursive...Ch. 20 - What type of recursive function do you think would...Ch. 20 - Which repetition approach is less efficient, a...Ch. 20 - When should you choose a recursive algorithm over...Ch. 20 - Explain what is likely to happen when a recursive...Ch. 20 - The _____________ of recursion is the number of...
Ch. 20 - Prob. 7RQECh. 20 - Prob. 8RQECh. 20 - Prob. 9RQECh. 20 - Write a recursive function to return the number of...Ch. 20 - Write a recursive function to return the largest...Ch. 20 - #include iostream using namespace std; int...Ch. 20 - Prob. 13RQECh. 20 - #include iostream #include string using namespace...Ch. 20 - Iterative Factorial Write an iterative version...Ch. 20 - Prob. 2PCCh. 20 - Prob. 3PCCh. 20 - Recursive Array Sum Write a function that accepts...Ch. 20 - Prob. 5PCCh. 20 - Prob. 6PCCh. 20 - Prob. 7PCCh. 20 - Prob. 8PCCh. 20 - Prob. 9PCCh. 20 - Prob. 10PCCh. 20 - Prob. 11PCCh. 20 - Ackermanns Function Ackermanns Function is a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. [Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]arrow_forwardIf a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? Order, Product, store, customer.arrow_forward
- Inheritance & Polymorphism (Ch11) There are 6 classes including Person, Student, Employee, Faculty, and Staff. 4. Problem Description: • • Design a class named Person and its two subclasses named student and Employee. • Make Faculty and Staff subclasses of Employee. • A person has a name, address, phone number, and e-mail address. • • • A person has a class status (freshman, sophomore, junior and senior). Define the status as a constant. An employee has an office, salary, and date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString() method in each class to display the class name and the person's name. 4-1. Explain on how you would code this program. (1 point) 4-2. Implement the program. (2 point) 4-3. Explain your code. (2 point)arrow_forwardSuppose you buy an electronic device that you operate continuously. The device costs you $300 and carries a one-year warranty. The warranty states that if the device fails during its first year of use, you get a new device for no cost, and this new device carries exactly the same warranty. However, if it fails after the first year of use, the warranty is of no value. You plan to use this device for the next six years. Therefore, any time the device fails outside its warranty period, you will pay $300 for another device of the same kind. (We assume the price does not increase during the six-year period.) The time until failure for a device is gamma distributed with parameters α = 2 and β = 0.5. (This implies a mean of one year.) Use @RISK to simulate the six-year period. Include as outputs (1) your total cost, (2) the number of failures during the warranty period, and (3) the number of devices you own during the six-year period. Your expected total cost to the nearest $100 is _________,…arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forward
- Please answer the JAVA OOP Programming Assignment scenario below: Patriot Ships is a new cruise line company which has a fleet of 10 cruise ships, each with a capacity of 300 passengers. To manage its operations efficiently, the company is looking for a program that can help track its fleet, manage bookings, and calculate revenue for each cruise. Each cruise is tracked by a Cruise Identifier (must be 5 characters long), cruise route (e.g. Miami to Nassau), and ticket price. The program should also track how many tickets have been sold for each cruise. Create an object-oriented solution with a menu that allows a user to select one of the following options: 1. Create Cruise – This option allows a user to create a new cruise by entering all necessary details (Cruise ID, route, ticket price). If the maximum number of cruises has already been created, display an error message. 2. Search Cruise – This option allows to search a cruise by the user provided cruise ID. 3. Remove Cruise – This op…arrow_forwardI need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.arrow_forwardSouthern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not). The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15. A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots): The average daily utilization of the aircraft (percentage of total flights that fly) The…arrow_forward
- Why is JAVA OOP is really difficult to study?arrow_forwardMy daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forwardQ4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
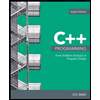
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
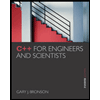
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
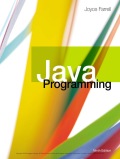
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
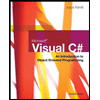
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
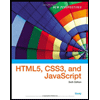
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning