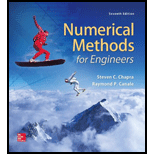
To calculate: The thermocline depth and the flux across the interface by the use of a cubic spline fit where
Depth, m | 0 | 0.5 | 1.0 | 1.5 | 2.0 | 2.5 | 3.0 |
Temperature, Celsius | 70 | 68 | 55 | 22 | 13 | 11 | 10 |
The provided graph shows the relationship between depth and temperature as,

Answer to Problem 6P
Solution:
The value of thermocline depth and the flux across the interface is
Explanation of Solution
Given Information:
The table is given as,
Depth, m | 0 | 0.5 | 1.0 | 1.5 | 2.0 | 2.5 | 3.0 |
Temperature, Celsius | 70 | 68 | 55 | 22 | 13 | 11 | 10 |
The provided graph shows the relationship between depth and temperature as,
Calculation:
Consider the Fourier’s law,
The value of
From the graph, this can be interpreted that curve has zero slope at
Since, the cubic spline fit is required, so this problem can be solved by the Excel VBA(Visual Basic for applications). The steps are,
Step 1. Insert the data in excel as shown below,
Step 2. Press ALT+F11 and write the code as shown below,
Step 3. Press RUN then this dialog box appears.
Step 4. Enter the value of z.
Step 5. This output will appear.
Thus, the value of
Hence, the value of thermocline depth and the flux across the interface is
Want to see more full solutions like this?
Chapter 20 Solutions
Numerical Methods for Engineers
- ind Original: 100-200 = 20,000 200400=20,000 80 602=3600 694761 =4 4x1.3225-6.29 4761/3600 = 1-3225 5.29-1058msy 6). The dose to the body was 200 mSv. Find the dose to the lung in mrem? W 200ms 20 2.15 and 8) A technique of 20 mAs, 40 kV produces a f4 Sy Find the dose to the Thyroid inarrow_forwardoriginal ssD 400x (100) 2 400 x (14)=100 34 10or (2)² = 100 × (0-85) = 100 x = 72.25 100x 40 72.25 =36.13 500 36.13 13.84 O. 7225 12x13.84≈ 166.08mAs 10) The dose of a radiograph was 100 mSv with a technique of: 10 mAs, 180 kV at 200 cm and tabletop. If the technique is changed to: 20 mAs, 153 kV at 100 cm using a 5:1 grid then find the new dose in rem to the Lungs. 11) A radiographic technique produces an exposure index of EI= 300 and TEI=600 at a source- to-image receptor distance (SID) of 200 cm, 100 kV, 5:1 grid using 10 mAs. If the technique is changed to 100 cm and 85 kV and 5 mAs with table top find the new exposure index? What is the value of DI? 10arrow_forward0. 75 and 34 KV arved fromise to 75 halfed NAME Genesis Ward 0 mAs is 40 #2). A technique involves 150 mAs, 40 kV and produces an intensity of 2 R. are gone down KV C15%! In = 200% Find the new intensity in mGya, using 300 mAs and 46 kV? =100 206a 150mAS 40KV 2R kv 150 is so divide 00 mA, 200 alue of 100. Boomts 46KY 4). A technique is taken with 100 mA, 200 ms, 60 kV and produces 200 mSv.arrow_forward
- Fi is 2 O 2 ms, #3). A technique is taken with 100 mA, 200 60 kV and produces an EI value of 100. Find the EI value when this technique is changed to 200 mA, 100 ms, 69 kV? 4) m 2arrow_forward11) A radiographic technique produces an exposure index of EI= 300 and TEI=600 at a source- to-image receptor distance (SID) of 200 cm, 100 kV, 5:1 grid using 10 mAs. If the technique is changed to 100 cm and 85 kV and 5 mAs with table top find the new exposure index? What is the value of DI?arrow_forward5). The dose to the breast was 120 mGy find the entire body dose represented in rad?arrow_forward
- 1000 x= 800mGy to body X= = rad 7). If EI=300, TEI=500 with mAs = 20 and you want to decrease the kV by 15% then 0.8 Gy what is the new mAs value to set DI=0? =0% 100-00 Dorad 8) A te dose of rem w kV? 2 tom i sv 4sv 48rem to thyr. KV+ I formula K In = Fox2 kV (1979) (48 rem = 8.2 = 16. SVx 100 160oren-body 1600.0.03=X I M M Earrow_forward8 If f(x + y) = f(x)f(y) and Σ f (x) = 2, x, y = N, x=1 where N is the set of all natural number, then the f(4) value of is. f(2)arrow_forwardvide 0. OMS its 150MAS 40k 300mts 46KV 4). A technique is taken with 100 mA, 200 ms, 60 kV and produces 200 mSv. Find the intensity in rem when this technique is changed to 200 mA, 400 ms, 69 kV? nd 5). The dose to the body was 200 mSy. Findarrow_forward
- Genesis Ward #9) A good exposure is taken using a technique of 12 mAs, 200 cm SSD,40kv, table top, and produces an EI value of 400 with a TEI value of 500. Find the new mAs value required to set DI=0, if a 100 cm SSD,34kV and a 6:1 grid were substituted. 12 MAS, 200cm SSD, 40kv E1 = 400 TEL = 500 of a radiograph was 100 mSv with a technique of: 10 mAs, 180 kV at 200 cm and 2 IV at 00 cm using a 5:1 grid then find thearrow_forward24.4. For the function g(z) defined in (18.7), show that g(z) = j=0 z2j (−1)³ (2j+1)!" Hence, deduce that the function g(z) is entire. 2 E C.arrow_forwardShow three different pairs of integers, a and b, where at least one example includes a negative integer.arrow_forward
- Algebra & Trigonometry with Analytic GeometryAlgebraISBN:9781133382119Author:SwokowskiPublisher:CengageFunctions and Change: A Modeling Approach to Coll...AlgebraISBN:9781337111348Author:Bruce Crauder, Benny Evans, Alan NoellPublisher:Cengage LearningGlencoe Algebra 1, Student Edition, 9780079039897...AlgebraISBN:9780079039897Author:CarterPublisher:McGraw Hill
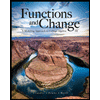
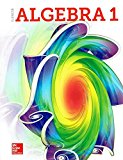
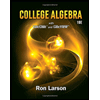