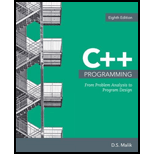
Concept explainers
Implementation of a
Program Plan:
Write a C++ program with a main function and the required set of statements to accomplish the following:
Write C++ statements that include the header files iostream and string
Write a C++ statement that allows you to use cin, cout, and endl without the prefix std::.
Write C++ statements that declare the following variables: name of type string and studyHours of type double
Write C++ statements that prompt and input a string into name and a double value into studyHours
Write a C++ statement that outputs the values of name and studyHours with the appropriate text. For example, if the value of name is "Donald" and the value of studyHours is 4.5, the output is,
Hello, Donald! on Saturday, you need to study 4.5 hours for the exam.
Compile and run your program

Trending nowThis is a popular solution!

Chapter 2 Solutions
C++ Programming: From Problem Analysis to Program Design
- This is a C++ computer programming question Write C++ statements to perform the following string operations String Operation C++ Statement Declare string1 and string2 to initialize your first name and last name Make a string3 after concatenate string1 and string2 Update the string3 by inserting a single space between first name and last name using build in function Calculate and show the total length of string3 Replace a single space between first name and last name with underscore using build in functionarrow_forwardWrite a c++ program that uses a structure named Movie Data to store the following information about a movie. Title (string) Director (string) Year Released (int) Running Time (in minut) (int) The program should create two MovieDate variables; store values in their members, and display the information about movie.arrow_forwardInstructions: In C++ Programming, write a program that calculates and prints the monthly paycheck for an employee. The net pay is calculated after taking the following deductions: Federal Income Tax: 15% State Tax: 3.5% Social Security Tax: 5.75% Medicare/Medicaid Tax: 2.75% Pension Plan: 5% Health Insurance: $75.00 Your program should prompt the user to input the gross amount and the employee name. The output will be stored in a file. Format your output to have two decimal places. A sample output follows: Bill Robinson Gross Amount: .......................... $3575.00 Federal Tax: ................................ $ 536.25 State Tax: .....................................$ 125.13 Social Security Tax: ................... $ 205.56 Medicare/Medicaid Tax: ......... $ 98.31 Pension Plan: .............................. $ 178.75 Health Insurance: .......................$ 75.00 Net Pay: ....................................... $2356.00arrow_forward
- in c++ add the request for the month number and read it in, add the request for the year and read it in, Ask what format the user would like to see the date in and write the if statement that will use the value in (format variable) to print out the date in the correct format. */ #include <iostream> using namespace std; int main() { short day, month, year, format; // Request day of the month (as a number) and put in day variable cout << "Enter a day of the month (1, 12, 30, etc): "; cin >> day; cout << "Formats are (1) YYYY/MM/DD, (2) DD/MM/YYYY, and (3) MM/DD/YYYY" << endl; cout << "Enter the number for the format you want (1, 2, or 3): "; cin >> format; }arrow_forwardin c++ Write a program to generate a random number between 1 - 100, and then display which quartile the number falls in. First quartile is 1 - 25 Second quartile is 26 - 50 Third quartile is 51 - 75 Fourth quartile is 76 - 100 To generate a random number, follow these steps: include necessary header files #include <cstdlib> //for random functions #include <ctime> //for time functions set constants for the minimum and maximum values of the desired range const int MIN_VALUE = 1; //minimum range value const int MAX_VALUE = 100; //maximum range value seed the random number generator (RNG) with a unique unsigned int value - system time! unsigned seed = time(0); //system time in seconds since 1/1/1970 srand(seed); //seed the RNG get a random number in the desired range int num = (rand() % (MAX_VALUE - MIN_VALUE + 1)) + MIN_VALUE; The program should: contain header comments as shown in class display a "hello" message (more descriptive than shown in sample)…arrow_forwardExercise #3: Character Counts Write a c++ program that prompts the user to enter a string, calls the function lowerUpperDigits( ) which takes a string and counts the number of lower, upper, and digit characters in the string. The main program prints the number of found characters. int lowerUpperDigits(string, int&, int&); Sample input / output:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
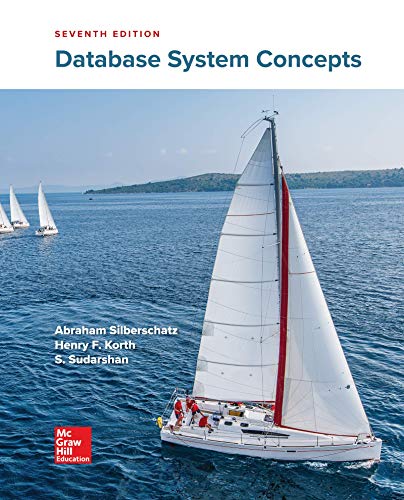
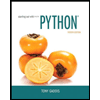
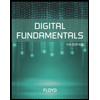
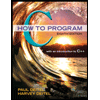
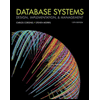
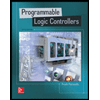