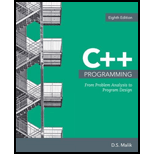
Concept explainers
Explanation of Solution
Errors in the program have been pointed out below each line of code.
#include<ioStream>
ioStream should be iostream as C++ is case sensitive.
using std;
The statement is supposed to specify the namespace. Correct statement:
using namespace std;
const double DECIMAL# = 5.50;
The name of the identifier (variable) should not contain # as only letters, digits, and underscores are allowed. Correct statement:
const double DECIMAL = 5.50;
const string blanks = " "
The statement should terminate with a semi-colon. Correct statement:
const string blanks = " ";
int ()main
The brackets should come after the word main as main is a function in C++ and any function identifier is followed by brackets (empty or otherwise). Correct statement:
int main()
{
int height, weight;
(no error)
double 10%discount
The name of the identifier (variable) should not contain # as only letters, digits, and underscores are allowed. It should begin with an underscore or letters and not digits. Also the statement should be terminated with a semi-colon. Correct statement:
double discount;
double billingAmount$;
double bonus;
int hoursWorked = 45;
(no error)
height = 6.2;
The above statement does not have a syntax error. However, since the variable is of int type implicit conversion to int type will take place and the value of 6.2 would be truncated to 6, the intended functionality would not be accomplished. However, since there is no syntax error, we will keep the statement as it is.
weight = 156;
(no error)
cout << height << " " << weight << end;
The above statement ends with a wrong manipulator. It should be endl and not end. Correct statement:
cout << height << " " << weight << endl;
discount = (2 * height + weight) % 10.0
The statement has multiple errors. The % operator expects only integer operands and hence, it should be 10 and not 10.0. The semi-colon terminator is missing. Correct statement:
discount = (2 * height + weight) % 10;
price = 49.99;
The variable has not been declared properly as the data type is missing. Since the variable intends to hold a floating point value, it would be appropriate to declare it as a double type...

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
C++ Programming: From Problem Analysis to Program Design
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
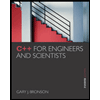
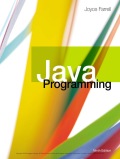
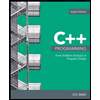
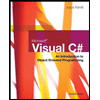
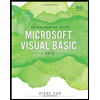