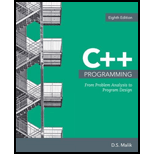
Concept explainers
Explanation of Solution
Below, the program execution and the output (if applicable) for relevant blocks of code are explained:
#include <iostream>
#include <string>
using namespace std;
const double CONVERSION = 3.5;
Explanation:
In the above lines of code, the constant CONVERSION is declared and initialized.
int main() {
const int TEMP = 23;
string name;
int id;
int num;
double decNum;
double mysteryNum;
Explanation:
In the above lines, inside the main function, the declaration and initialization of the constant TEMP is followed by the declaration of the variables name, id, num, decNum and mysteryNum.
cout << "Enter last name: ";
Explanation:
The stream insertion operator << and cout is used to print a string "Enter last name: ". The insertion point remains in the same line as the string output on the console. So the output is:
Enter last name:
cin >> name;
Explanation:
The stream extraction operator >> and cin is used to accept the user input of a string ("Miller") which are assigned to the variable name. The insertion point remains in the same line as the string which was typed as input at the console.
cout << endl;
Explanation:
The stream insertion operator cout is used to move the insertion point to a new line through the use of the manipulator endl.
cout << "Enter a two digit integer: ";
Explanation:
The stream insertion operator << and cout are used to print a string "Enter a two digit integer: ". The insertion point remains in the same line as the string output on the console. So the output is,
Enter a two digit integer:
cin >> id;
Explanation:
The stream extraction operator >> and cin are used to accept the user input of a two-digit number (34) which is assigned to the variable id. The insertion point remains in the same line as the string which was typed as input at the console.
cout << endl;
Explanation:
The stream insertion operator << and cout are used to move the insertion point to a new line through the use of the manipulator endl.
num = (id * TEMP) % (static_cast<int>(CONVERSION));
Explanation:
The assignment statement assigns a new value to the variable num after evaluating the right-hand-side arithmetic expression. The expression is evaluated as follows,
(id * TEMP) % (static_cast<int>(CONVERSION))
= (id * TEMP) % (static_cast<int>(CONVERSION))
= (34 * 23) % (static_cast<int>(3.5)) (value substitution)
= (34 * 23) % (3) (static_cast operator converts the floating point
number into int type discarding the decimal portion)
= 782 % 3 (parentheses has highest precedence, * operator
evaluated)
= 2 (modulus operator - 782 / 3 leaves 2 as remainder)
cout << "Enter a decimal number: ";
Explanation:
The stream insertion operator cout is used to print a string "Enter a decimal number: "...

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
C++ Programming: From Problem Analysis to Program Design
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
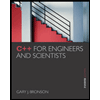
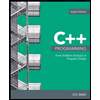
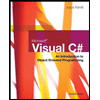
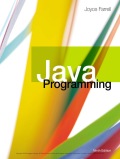