C++ PROGRAMMING LMS MINDTAP
8th Edition
ISBN: 9781337696173
Author: Malik
Publisher: Cengage Learning
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 2, Problem 3PE
Program Plan Intro
Implementation of a
Program Plan:
Write a C++ program with a main function and the required set of statements to accomplish the following:
Write a C++ statement that includes the header file iostream
Write a C++ statement that allows you to use cin, cout, and endl without the prefix std::.
Write C++ statement(s) that declare the following variables: num1, num2, num3, and average of type int.
Write C++ statements that store 125 into num1, 28 into num2, and -25 into num3
Write a C++ statement that stores the average of num1, num2, and num3 into average
Write C++ statement(s) that output the values of num1, num2, num3, and average
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
reminder it an exercice not a grading work
GETTING STARTED
Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website.
Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”.
If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically.
With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet.
If cell B6 does not display your name, delete the file and download a new copy from the SAM website.
Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…
Need help completing this algorithm here in coding! 2
Whats wrong the pseudocode here??
Chapter 2 Solutions
C++ PROGRAMMING LMS MINDTAP
Ch. 2 - 1. Mark the following statements as true or...Ch. 2 - Prob. 2MCCh. 2 - Which of the following is not a reserved word in...Ch. 2 - Prob. 4SACh. 2 - 5. Are the identifiers quizNo1 and quiznol the...Ch. 2 - 6. Evaluate the following expressions. (3,...Ch. 2 - If int x = 10;, int y = 7;, double z = 4.5;, and...Ch. 2 - Prob. 8CPCh. 2 - 9. Suppose that x, y, z, and w are int variables....Ch. 2 - Prob. 10SA
Ch. 2 - Which of the following are valid C++ assignment...Ch. 2 - Write C++ statements that accomplish the...Ch. 2 - Write each of the following as a C++ expression....Ch. 2 - Prob. 14SACh. 2 - Suppose x, y, and z are int variables and wandt...Ch. 2 - 16. Suppose x, y, and z are int variables and x =...Ch. 2 - Suppose a and b are int variables, c is a double...Ch. 2 - 18. Write C++ statements that accomplish the...Ch. 2 - Which of the following are correct C++ statements?...Ch. 2 - Give meaningful identifiers for the following...Ch. 2 - 21. Write C++ statements to do the following....Ch. 2 - Prob. 22SACh. 2 - The following program has syntax errors. Correct...Ch. 2 - Prob. 24SACh. 2 - Prob. 25SACh. 2 - Preprocessor directives begin with which of the...Ch. 2 - 27. Write equivalent compound statements if...Ch. 2 - 28. Write the following compound statements as...Ch. 2 - 29. Suppose a, b, and c are int variables and a =...Ch. 2 - Suppose a, b, and sum are int variables and c is a...Ch. 2 - Prob. 31SACh. 2 - Prob. 32SACh. 2 - Prob. 33SACh. 2 - Prob. 34SACh. 2 - 1. Write a program that produces the following...Ch. 2 - Prob. 2PECh. 2 - Prob. 3PECh. 2 - 4. Repeat Programming Exercise 3 by declaring...Ch. 2 - Prob. 5PECh. 2 - Prob. 6PECh. 2 - 7. Write a program that prompts the user to input...Ch. 2 - Prob. 8PECh. 2 - 9. Write a program that prompts the user to enter...Ch. 2 - 10. Write a program that prompts the user to input...Ch. 2 - 11. Write a program that prompts the capacity, in...Ch. 2 - 12. Write a C++ program that prompts the user to...Ch. 2 - 13. To make a profit, a local store marks up the...Ch. 2 - 14. (Hard drive storage capacity) If you buy a 40...Ch. 2 - 15. Write a program to implement and test the...Ch. 2 - 16. A milk carton can hold 3.78 liters of milk....Ch. 2 - 17. Redo Programming Exercise 16 so that the user...Ch. 2 - Prob. 18PECh. 2 - 19. Write a program that prompts the user to input...Ch. 2 - 20. For each used car a salesperson sells, the...Ch. 2 - 21. Newton's law states that the force, , between...Ch. 2 - 22. One metric ton is approximately 2,205 pounds....Ch. 2 - 23. Cindy uses the services of a brokerage firm to...Ch. 2 - 24. A piece of wire is to be bent in the form of a...Ch. 2 - 25. Repeat Programming Exercise 24, but the wire...Ch. 2 - 26. A room has one door, two windows, and a...Ch. 2 - Prob. 27PECh. 2 - 28. In an elementary school, a mixture of equal...Ch. 2 - 29. A contractor orders, say, 30 cubic yards of...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Help! how do I fix my python coding question for this? (my code also provided)arrow_forwardNeed help with coding in this in python!arrow_forwardIn the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forward
- Please provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forwardWhy I need ?arrow_forwardHere are two diagrams. Make them very explicit, similar to Example Diagram 3 (the Architecture of MSCTNN). graph LR subgraph Teacher_Model_B [Teacher Model (Pretrained)] Input_Teacher_B[Input C (Complete Data)] --> Teacher_Encoder_B[Transformer Encoder T] Teacher_Encoder_B --> Teacher_Prediction_B[Teacher Prediction y_T] Teacher_Encoder_B --> Teacher_Features_B[Internal Features F_T] end subgraph Student_B_Model [Student Model B (Handles Missing Labels)] Input_Student_B[Input C (Complete Data)] --> Student_B_Encoder[Transformer Encoder E_B] Student_B_Encoder --> Student_B_Prediction[Student B Prediction y_B] end subgraph Knowledge_Distillation_B [Knowledge Distillation (Student B)] Teacher_Prediction_B -- Logits Distillation Loss (L_logits_B) --> Total_Loss_B Teacher_Features_B -- Feature Alignment Loss (L_feature_B) --> Total_Loss_B Partial_Labels_B[Partial Labels y_p] -- Prediction Loss (L_pred_B) --> Total_Loss_B Total_Loss_B -- Backpropagation -->…arrow_forward
- Please provide me with the output image of both of them . below are the diagrams code I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder --> Student_A_Features[Student A Features F_A] end subgraph Knowledge_Distillation_A [Knowledge Distillation (Student A)] Teacher_Prediction -- Logits Distillation Loss (L_logits_A) --> Total_Loss_A Teacher_Features -- Feature Alignment Loss (L_feature_A) --> Total_Loss_A Ground_Truth_A[Ground Truth y_gt] -- Prediction Loss (L_pred_A)…arrow_forwardI'm reposting my question again please make sure to avoid any copy paste from the previous answer because those answer did not satisfy or responded to the need that's why I'm asking again The knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges…arrow_forwardThe knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges (missing values vs. missing labels), they should not be combined in the same diagram. Instead, create two separate diagrams for clarity. For reference, I will attach a second image…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
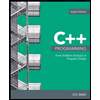
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
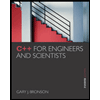
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
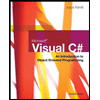
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
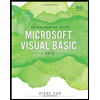
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
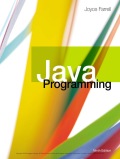
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT