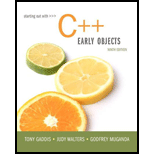
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 2PC
Program Plan Intro
Tree Size
Program Plan:
- Define a class BTreeNode to create nodes for binary search tree items.
- Include all the required header files.
- Initialize a value to the node, and set the left child of node to leftp and right child of node to rightp .
- Define a class to create a Binary Search Tree.
- Create a function int size that returns no. of items present in the tree.
- Create a function bool search to search a particular item in tree.
- Create function void insert to insert nodes into the tree.
- Create a function void inorder to sort items in inorder traversal.
- Declare the main function.
- Prompt the user to enter 5 numbers to be inserted into tree.
- Sort the items present in the tree in inorder traversal and return the number of items present in the tree.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Python binary search tree: a function that takes in a root, p, and checks whether the tree rooted in p is a binary search tree or not. What is the time complexity of your function?
def is_bst(self, p: Node):
struct insert_into_bst {
// Function takes a constant Book as a parameter, inserts that book indexed by
// the book's ISBN into a binary search tree, and returns nothing.
void operator()(const Book& book) {
// // TO-DO (7) |||
/////
// Write the lines of code to insert the key (book's ISBN) and value
// ("book") pair into "my_bst".
END-TO-DO (7) |
}
std::map& my_bst;
};
Get the Longest Path
Write a member function called DLList BST::
get longest_path() that returns a DLL of the longest path in
the tree. For example, the red nodes in the following tree are on
the longest path and should be added to the list. In case there
are multiple longest paths, retrieve any of them.
Chapter 19 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 19.1 - Prob. 19.1CPCh. 19.1 - Prob. 19.2CPCh. 19.1 - Prob. 19.3CPCh. 19.1 - Prob. 19.4CPCh. 19.1 - Prob. 19.5CPCh. 19.1 - Prob. 19.6CPCh. 19.2 - Prob. 19.7CPCh. 19.2 - Prob. 19.8CPCh. 19.2 - Prob. 19.9CPCh. 19.2 - Prob. 19.10CP
Ch. 19.2 - Prob. 19.11CPCh. 19.2 - Prob. 19.12CPCh. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - Prob. 3RQECh. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - Prob. 6RQECh. 19 - Prob. 7RQECh. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - Prob. 17RQECh. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Prob. 6PCCh. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a recursive function called "GetParent" in Binary Search Tree of the given node. You can take as many arguments as you like. Use the following structure definition. struct TNode{ char NodeName[10]; TNode *LeftT, *RightT; } root;arrow_forwardGiven the following struct that represents a binary tree: struct Node { int key: Node "parent; Node "left; Node "right; Nodelint k) : key(k), parent(nullptr), left(nullptr), right(nullptr) (I: 1: Write a recursive function that prints out the nodes in a tree stored using the above structure in order to cout. The function prints the depth (root depth is at 0) and key of that node separated by a colon (Example "O: 10\n" for root with key 10). Your function CAN NOT create any local variables and can only use what is passed to the function. Use the below function signature (NOTE: this is not a class method). void inorderAndDepth(Node "node, int depth)arrow_forwardTask: Complete the function getMinDepth (Node *root), the function takes the root of a tree and returns the minimum depth of the tree. int getMinDepth(Node *root){ //write your code here } Constraints: The number of nodes in the tree is in the range [0, 100000]. -1000 <= Node.val <= 1000arrow_forward
- 4. Complete the fuction definition given below that takes the root node of a tree as a parameter and returns the count of nodes. int count_nodes(Node *root){ // complete the function }arrow_forwardPlease help with C++ question in image. Thank you.arrow_forwardwrite a recursive function called "getparent" in binary search tree of the given node. you can take as many arguments as you like use the following structure definition struct TNode{ int data; TNode *LeftT, *RightT; }root;arrow_forward
- C++ DATA STRUCTURES Implement the TNode and Tree classes. The TNode class will include a data item name of type string,which will represent a person’s name. Yes, you got it right, we are going to implement a family tree!Please note that this is not a Binary Tree. Write the methods for inserting nodes into the tree,searching for a node in the tree, and performing pre-order and post-order traversals.The insert method should take two strings as input. The second string will be added as a child node tothe parent node represented by the first string. Hint: The TNode class will need to have two TNode pointers in addition to the name data member:TNode *sibling will point to the next sibling of this node, and TNode *child will represent the first child ofthis node. You see two linked lists here??? Yes! You’ll need to use the linked listsarrow_forwardCourse : Data Structure and Algorithms Write a java/c++ code or an algorithm to solve the following problem. After that dry run and show output of algorithm using an example binary tree. . Write a recursive function to write the parent of the all the nodes while traversing. Like if you traverse the root node write the current node a, parent null. Then if you go to left sub tree, your code will show, current node : b , parent a and so on.arrow_forwardWrite a function that creates a binary search tree from elements in a given sequence. Function must return the pointer back to root of tree. Please explain the code. TNODEPTR makeBST (int sequence[], int n) // n = number of elements Binary tree root. struct node { } int info; struct tnode *left, *right; typedef struct node *TNODEPTR;arrow_forward
- python: In a binary search tree, write another way of function that takes in a root, p, and checks whether the tree rooted in p is a binary search tree or not. What is the time complexity of your function? def is_bst(self, p): root=p def helper(root, l, r): if not root: return True if not (left<root.val and root.val<right): return False return helper(root.l, l, root.val) and helper(root.r, root.val, r) return helper(root, float('-inf'), float('inf'))arrow_forwardT/F Suffix array is space efficient and faster than the suffix tree.arrow_forwardHelp on the following question A co-worker emails you and said she developed a recursive version for doing search in a binary search tree. Here’s the code for the function: public boolean searchRecursive(Node current, int searchValue) { if (current == null) return false; if (current.data == searchValue) return true; else if (current.data > searchValue) return searchRecursive(current.right, searchValue); else return searchRecursive(current.left, searchValue); } She’s not sure if there is an error or not because the code does compile. You analyze the code and respond to her as follows: Draw a picture of what a binary search tree would look like after inserting values of 10, 15, 18, 13, 5, 1, and 8 in that order Next, if you believe there is no error with the code, then show her how the code executes when searching for different values using the tree you made in step 1) Or, if you believe there is…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
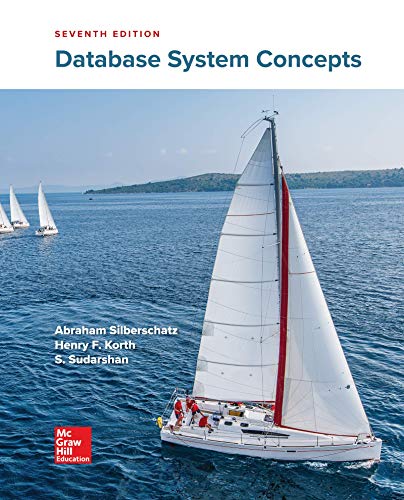
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
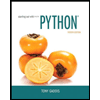
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
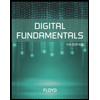
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
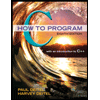
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
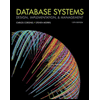
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
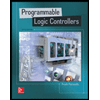
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education