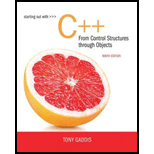
Starting Out with C++ from Control Structures to Objects Plus MyLab Programming with Pearson eText -- Access Card Package (9th Edition)
9th Edition
ISBN: 9780134544847
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 30RQE
Program Plan Intro
Linked list:
Linked list is a linear and dynamic data structure which is used to organize data; it contains sequence of elements which are connected together in memory to form a chain. The every element of linked list is called as a node.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
struct insert_at_back_of_dll {
// Function takes a constant Book as a parameter, inserts that book at the
// back of a doubly linked list, and returns nothing.
void operator()(const Book& book) {
/ // TO-DO (2) ||||
// Write the lines of code to insert "book" at the back of "my_dll".
//
// // END-TO-DO (2) |||
}
std::list& my_dll;
};
C++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow
#include "stack.h"
#include "linkedlist.h"
// SLLStack means Singly Linked List (SLL) Stack
class SLLStack : public Stack {
LinkedList* list;
public:
SLLStack() {
list = new LinkedList();
}
void push(char e) {
list->add(e);
return;
}
char pop() {
char elem;
elem = list->removeTail();
return elem;
}
char top() {
char elem;
elem = list->get(size());
return elem;
}
int size() {
return list->size();
}
bool isEmpty() {
return list->isEmpty();
}
};
The definition of linked list is given as follows:
struct Node {
ElementType Element ;
struct Node *Next ;
} ;
typedef struct Node *PtrToNode, *List, *Position;
If L is head pointer of a linked list, then the data type of L should be ??
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects Plus MyLab Programming with Pearson eText -- Access Card Package (9th Edition)
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - Prob. 18.2CPCh. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.2 - Prob. 18.5CPCh. 18.2 - Prob. 18.6CPCh. 18.2 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - Prob. 3RQECh. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - Prob. 6RQECh. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - T F The programmer must know in advance how many...Ch. 18 - T F It is not necessary for each node in a linked...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 33RQECh. 18 - Prob. 34RQECh. 18 - Prob. 35RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - List Template Create a list class template based...Ch. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- struct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forwardC++The List class represents a linked list of dynamically allocated elements. The list has only one member variable head which is a pointer that leads to the first element. See the following code for the copy constructor to List. List (const List & list) { for (int i = 0; i <list.size (); i ++) { push_back (list [i]); } } What problems does the copy constructor have? Select one or more options: 1. List (const List & list) {} does not define a copy constructor. 2. The list parameter should not be constant. 3. The new elements will be added to the wrong list. 4. Copy becomes shallow rather than deep. 5. The copy will create dangling pointers. 6. Copying will create memory leaks. 7. The condition must be: i <size () 8. head is never initialized.arrow_forwardC++ DOUBLY LINKED LIST: Implement the remove_between function for my code: Incomplete code: #include <cstdlib>#include <iostream>using namespace std; class DoublyLinkedList : public List { node* head; node* tail; int index; node* create_node(int num, node* predecessor, node* successor) { node* n = (node*) malloc( sizeof(node) ); n->element = num; n->next = successor; n->prev = predecessor; return n; } void add_between(int num, node* predecessor, node* successor) { node* newest = create_node(num, predecessor, successor); predecessor->next = newest; successor->prev = newest; index++; } void remove_between(int num, node* predecessor, node* successor) { ///// CODE HERE }arrow_forward
- C++ The List class represents a linked list of dynamically allocated elements. The list has only one member variable head which is a pointer that leads to the first element. See the following code for the destructor to List. ~ List () { for (int i = 0; i <size (); i ++) { pop_back (); } } What problems does the destructor have? Select one or more options: 1. There are no parameters for the destructor. 2. The return value from pop_back (if any) is nerver handled. 3. The destructor will create a stack overflow. 4. The destructor will create dangling pointers. 5.The destructor will create memory leaks. 6.The destructor will create undefined behavior (equivalent to zero pointer exception). 7.The condition must be: i <size () - 1 8. There is at least one problem with the destructor, but none of the above.arrow_forwardstruct remove_from_front_of_dll { // Function takes no parameters, removes the book at the front of a doubly // linked list, and returns nothing. void operator()(const Book& unused) { //// TO-DO (13) |||| // Write the lines of code to remove the book at the front of "my_dll", // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. ///// END-TO-DO (13) //// } std::list& my_dll; };arrow_forwardC++ function Linked list Write a function, to be included in an unsorted linked list class, called replaceItem, that will receive two parameters, one called olditem, the other called new item. The function will replace all occurrences of old item with new item (if old item exists !!) and it will return the number of replacements done.arrow_forward
- C Programming language Part 1: You need to define a data structure for a doubly linked list and a binary search tree. Also, you need to implement the following functions: Insert Sorted LINKEDLIST insertSorted(LINKEDLIST head, int num): head points to the first node in the sorted linked list; num is a number to be inserted in in correct place in the linked list pointed at “head”. The linked list should be sorted after inserting “num”. This function returns the head of the modified head. BSTREE insert(BSTREE root, int num): root points to a node in a binary search tree; num is a number to be inserted in the tree rooted at “root”. This function returns the root of the modified tree. Find an element LINKEDLIST find(LINKEDLIST head,int num): head points to the first node of a linked list; num is a number to be searched for in the linked list started at “head”. This function returns a pointer to the node containing “num” or NULL if num is not found BSTREE find(BSTREE root,int…arrow_forwardC++ Queue LinkedList, Refer to the codes I've made as a guide and add functions where it can add/insert/remove function to get the output of the program, refer to the image as an example of input & output. Put a comment or explain how the program works/flow.arrow_forwardC++ Function to remove the first node of a linked listarrow_forward
- IMPLEMENT FUNCTIONS C++ #include "node.h"#include "list.h"#include <cstdlib>#include <iostream>using namespace std; class DoublyLinkedList : public List { node* head; node* tail; int index; node* create_node(int num, node* predecessor, node* successor) { node* n = (node*) malloc( sizeof(node) ); n->element = num; n->next = successor; n->prev = predecessor; return n; } void add_between(int num, node* predecessor, node* successor) { node* newest = create_node(num, predecessor, successor); predecessor->next = newest; successor->prev = newest; index++; } public: DoublyLinkedList() { index = 0; head = create_node(0, NULL, NULL); tail = create_node(0, head, NULL); head->next = tail; } ~DoublyLinkedList() { clear(); } void addHead(int num) { add_between(num, head,…arrow_forwardfor c++ please thank you 4, List search Modify the linked listv class you created in the previous programming challange to include a member function named search that returns the position of a specific value, x, in the lined list. the first node in the list is at position 0, the second node is at position 1, and so on. if x is not found on the list, the search should return -1 test the new member function using an approprate driver program. here is my previous programming challange. #include <iostream> using namespace std; struct node { int data; node *next; }; class list { private: node *head,*tail; public: list() { head = NULL; tail = NULL; } ~list() { } void append() { int value; cout<<"Enter the value to append: "; cin>>value; node *temp=new node; node *s; temp->data = value; temp->next = NULL; s = head; if(head==NULL) head=temp; else { while (s->next != NULL) s = s->next; s->next = temp; } } void insert() { int value,pos,i,count=0;…arrow_forward2-) In a double linked list, the structure of a node is defined as follows: struct node { int employeeNo; char name[20]; struct node *next; structnode *prev; }node; the nodes in the list are sorted according to employeeNo in ascending order (from smaller to larger ). Write a function to insert a node with a given name and employeeNo into the list so that the list will remain the sorted. employeeNo değerine göre kiüçükten büyüğe sıralanmış bir çift bağlı liste olsun. Verilen bir isim ve employeeNo değerine sahip düğümü listeye sıra bozulmayacak şekilde ekleyen bir fonksyion yazınız.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
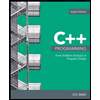
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning