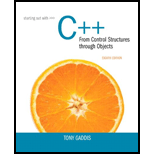
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17.2, Problem 17.10CP
Program Plan Intro
Linked list:
Linked list is a linear and dynamic data structure which is used to organize data; it contains sequence of elements which are connected together in memory to form a chain. The every element of linked list is called as a node.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
The following is code for a disc golf program written in C++:
// player.h
#ifndef PLAYER_H
#define PLAYER_H
#include <string>
#include <iostream>
class Player {
private:
std::string courses[20]; // Array of course names
int scores[20]; // Array of scores
int gameCount; // Number of games played
public:
Player(); // Constructor
void CheckGame(int playerId, const std::string& courseName, int gameScore);
void ReportPlayer(int playerId) const;
};
#endif // PLAYER_H
// player.cpp
#include "player.h"
#include <iomanip>
Player::Player() : gameCount(0) {}
void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) {
for (int i = 0; i < gameCount; ++i) {
if (courses[i] == courseName) {
// If course has been played, then check for minimum score
if (gameScore < scores[i]) {
scores[i] = gameScore; // Update to new minimum…
In this assignment, you will implement a multi-threaded program (using C/C++) that will
check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome
numbers are numbers that their decimal representation can be read from left to right and
from right to left (e.g. 12321, 5995, 1234321).
The program will create T worker threads to check for prime and palindrome numbers in the
given range (T will be passed to the program with the Linux command line). Each of the
threads works on a part of the numbers within the range.
Your program should have some global shared variables:
•
numOfPrimes: which will track the total number of prime numbers found by all
threads.
numOfPalindroms: which will track the total number of palindrome numbers found
by all threads.
numOfPalindromic Primes: which will count the numbers that are BOTH prime and
palindrome found by all threads.
TotalNums: which will count all the processed numbers in the range.
In addition, you need to have arrays…
How do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.
Chapter 17 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Prob. 17.7CPCh. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Prob. 10RQECh. 17 - Prob. 11RQECh. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 19RQECh. 17 - Prob. 20RQECh. 17 - Prob. 21RQECh. 17 - Prob. 22RQECh. 17 - Prob. 23RQECh. 17 - Prob. 24RQECh. 17 - Prob. 25RQECh. 17 - T F The programmer must know in advance how many...Ch. 17 - T F It is not necessary for each node in a linked...Ch. 17 - Prob. 28RQECh. 17 - Prob. 29RQECh. 17 - Prob. 30RQECh. 17 - Prob. 31RQECh. 17 - Prob. 32RQECh. 17 - Prob. 33RQECh. 17 - Prob. 34RQECh. 17 - Prob. 35RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - List Template Create a list class template based...Ch. 17 - Prob. 9PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Prob. 13PCCh. 17 - Prob. 14PCCh. 17 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- You are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forwardDistributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardOperating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
- Artificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 75 for graph-related problems. Instructions: • Implement a greedy graph coloring algorithm for the given graph. • Demonstrate the steps to assign colors while minimizing the chromatic number. • Analyze the time complexity and limitations of the approach. Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 150 for problems on socket programming. Instructions: • Develop a client-server application using sockets to exchange messages. • Implement both TCP and UDP communication and highlight their differences. • Test the program under different network conditions and analyze results. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 80 for problems on white-box testing. Instructions: • Perform control flow testing for the given program, drawing the control flow graph (CFG). • Design test cases to achieve statement, branch, and path coverage. • Justify the adequacy of your test cases using the CFG. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 60 for solving the Knapsack problem using dynamic programming. Instructions: • Implement the dynamic programming approach for the 0/1 Knapsack problem. Clearly define the recurrence relation and show the construction of the DP table. Verify your solution by tracing the selected items for a given weight limit. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 70 for problems related to process synchronization. Instructions: • • Solve a synchronization problem using semaphores or monitors (e.g., Producer-Consumer, Readers-Writers). Write pseudocode for the solution and explain the critical section management. • Ensure the solution avoids deadlock and starvation. Test with an example scenario. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward15 points Save ARS Consider the following scenario in which host 10.0.0.1 is communicating with an external SMTP mail server at IP address 128.119.40.186. NAT translation table WAN side addr LAN side addr (c), 5051 (d), 3031 S: (e),5051 SMTP B D (f.(g) 10.0.0.4 server 138.76.29.7 128.119.40.186 (a) is the source IP address at A, and its value. S: (a),3031 D: (b), 25 10.0.0.1 A 10.0.0.2. 1. 138.76.29.7 10.0.0.3arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
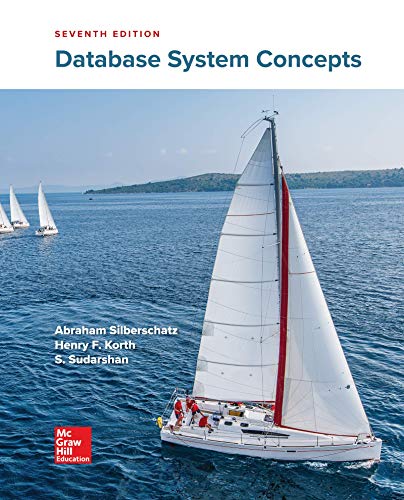
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
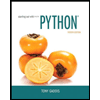
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
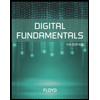
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
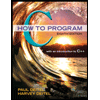
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
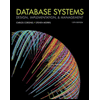
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
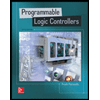
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education