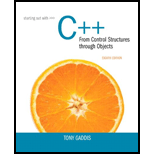
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 1PC
Program Plan Intro
Linked List Operations
Program Plan:
“IntList.h”:
- Include the required specifications into the program.
- Define a class named “IntList”.
- Declare the member variables “value” and “*next” in structure named “ListNode”.
- Declare the constructor, destructor, and member functions in the class.
“IntList.cpp”:
- Include the required header files into the program.
- Define a function named “appendNode()” to insert the node at end of the list.
- Declare the structure pointer variables “newNode” and “dataPtr” for the structure named “ListNode”.
- Assign the value “num” to the variable “newNode” and assign null to the variable “newNode”.
- Using “if…else” condition check whether the list is empty or not, if the “head” is empty then make a new node into “head” pointer. Otherwise, make a loop to find last node in the loop.
- Assign the value of “dataPtr” into the variable “newNode”.
- Define a function named “display()” to print the values in the list.
- Declare the structure pointer “dataPtr” for the structure named “ListNode”.
- Initialize the variable “dataPtr” with the “head” pointer.
- Make a loop “while” to display the values of the list.
- Define a function named “insertNode()” to insert a value into the list.
- Declare the structure pointer variables “newNode”, “dataPtr”, and “prev” for the structure named “ListNode”.
- Make a “newNode” value into the received variable value “num”.
- Use “if…else” condition to check whether the list is empty or not.
- If the list is empty then initialize “head” pointer with the value of “newNode” variable.
- Otherwise, make a “while” loop to test whether the “num” value is less than the list values or not.
- Use “if…else” condition to initialize the value into list.
- Define a function named “deleteNode()” to delete a value from the list.
- Declare the structure pointer variables “dataPtr”, and “prev” for the structure named “ListNode”.
- Use “if…else” condition to check whether the “head” value is equal to “num” or not.
- Initialize the variable “dataPtr” with the value of the variable “head”.
- Remove the value using “delete” operator and reassign the “head” value into the “dataPtr”.
- If the “num” value not equal to the “head” value, then define the “while” loop to assign the “dataPtr” into “prev”.
- Use “if” condition to delete the “prev” pointer.
- Define the destructor to destroy the list values from the memory.
- Declare the structure pointer variables “dataPtr”, and “nextNode” for the structure named “ListNode”.
- Initialize the variable “dataPtr” with the “head” pointer.
- Define a “while” loop to make the links of node into “nextNode” and remove the node using “delete” operator.
“Main.cpp”:
- Include the required header files into the program.
- Declare an object named “obj” for the class “IntList”.
- Make a call to functions for insert, append, and delete operations.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
D. S. Malik, Data Structures Using C++, 2nd Edition, 2010
Methods (Ch6) - Review
1. (The MyRoot method) Below is a manual implementation of the Math.sqrt() method in Java.
There are two methods, method #1 which calculates the square root for positive integers, and
method #2, which calculates the square root of positive doubles (also works for integers).
public class SquareRoot {
public static void main(String[] args) {
}
// implement a loop of your choice here
// Method that calculates the square root of integer variables
public static double myRoot(int number) {
double root;
root=number/2;
double root old;
do {
root old root;
root (root_old+number/root_old)/2;
} while (Math.abs(root_old-root)>1.8E-6);
return root;
}
// Method that calculates the square root of double variables
public static double myRoot(double number) {
double root;
root number/2;
double root_old;
do {
root old root;
root (root_old+number/root_old)/2;
while (Math.abs (root_old-root)>1.0E-6);
return root;
}
}
Program-it-Yourself: In the main method, create a program that…
I would like to know the main features about the following 3 key concepts:1. Backup Domain Controller (BDC)2. Access Control List (ACL)3. Dynamic Memory
Chapter 17 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Prob. 17.7CPCh. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Prob. 10RQECh. 17 - Prob. 11RQECh. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 19RQECh. 17 - Prob. 20RQECh. 17 - Prob. 21RQECh. 17 - Prob. 22RQECh. 17 - Prob. 23RQECh. 17 - Prob. 24RQECh. 17 - Prob. 25RQECh. 17 - T F The programmer must know in advance how many...Ch. 17 - T F It is not necessary for each node in a linked...Ch. 17 - Prob. 28RQECh. 17 - Prob. 29RQECh. 17 - Prob. 30RQECh. 17 - Prob. 31RQECh. 17 - Prob. 32RQECh. 17 - Prob. 33RQECh. 17 - Prob. 34RQECh. 17 - Prob. 35RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - List Template Create a list class template based...Ch. 17 - Prob. 9PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Prob. 13PCCh. 17 - Prob. 14PCCh. 17 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In cell C21, enter a formula to calculate the number of miles you expect to drive each month. Divide the value of number of miles (cell A5 from the Data sheet) by the average MPG for the vehicle multiplied by the price of a gallon of gas (cell A6 from the Data sheet).arrow_forwardMicrosoft Excelarrow_forwardIn cell C16, enter a formula to calculate the price of the vehicle minus your available cash (from cell A3 in the Data worksheet). Use absolute references where appropriate—you will be copying this formula across the row what fomula would i use and how do i solve itarrow_forward
- What types of data visualizations or tools based on data visualizations have you used professionally, whether in a current or past position? What types of data did they involve? What, in your experience, is the value these data views or tools added to your performance or productivity?arrow_forwardQuestion: Finding the smallest element and its row index and column index in 2D Array: 1. Write a public Java class min2D. 2. In min2D, write a main method. 3. In the main method, create a 2-D array myArray with 2 rows and 5 columns: {{10, 21, 20, 13, 1}, {2, 6, 7, 8, 14}}. 4. Then, use a nested for loop to find the smallest element and its row index and column index. 5. Print the smallest element and its row index and column index on Java Consolearrow_forward(using R)The iris data set in R gives the measurements in centimeters of the variables sepal length and width andpetal length and width, respectively, for 50 flowers from each of 3 species of iris, setosa, versicolor, andvirginica. Use the iris data set and the t.test function, test if the mean of pepal length of iris flowers isgreater than the mean of sepal length.The iris data set in R gives the measurements in centimeters of the variables sepal length and width andpetal length and width, respectively, for 50 flowers from each of 3 species of iris, setosa, versicolor, andvirginica. Use the iris data set and the t.test function, test if the mean of pepal length of iris flowers isgreater than the mean of sepal length.arrow_forward
- Recognizing the Use of Steganography in Forensic Evidence (4e)Digital Forensics, Investigation, and Response, Fourth Edition - Lab 02arrow_forwardWrite a Java Program to manage student information of a university. The Javaprogram does the following steps:a) The program must use single-dimensional arrays to store the studentinformation such as Student ID, Name and Major.b) The program asks the user to provide the number of students.c) The program asks the user to enter the Student IDs for the number of studentsand stores them.d) The program asks the user to enter the corresponding names for the numberof students and stores them.e) The program then asks the user to provide the corresponding major for thestudents and stores them.f) The program then should display the following options:1. ID Search2. Major Enrollment3. Exitg) On selecting option 1, the user can search for a student using Student ID. Theprogram asks the user to enter a Student ID. It then should print thecorresponding student’s details such as Name and Major if the user providedStudent ID number is present in the stored data. If the user’s Student IDnumber does not…arrow_forward(a) Algebraically determine the output state |q3q2q1q0> (which is a 4-qubitvector in 16-dimensional Hilbert space). Show all steps of your calculations. (b) Run a Qiskit code which implements the circuit and append threemeasurement gates to measure the (partial) output state |q2q1q0> (which is a 3-qubit vector in 8-dimensional Hilbert space). this is for quantum soft dev class, you can use stuff like Deutsch Jozsa if u wantarrow_forward
- Write a C++ program that will count from 1 to 10 by 1. The default output should be 1, 2, 3, 4, 5, 6 , 7, 8, 9, 10 There should be only a newline after the last number. Each number except the last should be followed by a comma and a space. To make your program more functional, you should parse command line arguments and change behavior based on their values. Argument Parameter Action -f, --first yes, an integer Change place you start counting -l, --last yes, an integer Change place you end counting -s, --skip optional, an integer, 1 if not specified Change the amount you add to the counter each iteration -h, --help none Print a help message including these instructions. -j, --joke none Tell a number based joke. So, if your program is called counter counter -f 10 --last 4 --skip 2 should produce 10, 8, 6, 4 Please use the last supplied argument. If your code is called counter, counter -f 4 -f 5 -f 6 should count from 6. You should count from first to last inclusively.…arrow_forwardWrite a program that will count from 1 to 10 by 1. The default output should be 1, 2, 3, 4, 5, 6 , 7, 8, 9, 10 There should be only a newline after the last number. Each number except the last should be followed by a comma and a space. To make your program more functional, you should parse command line arguments and change behavior based on their values. Argument Parameter Action -f, --first yes, an integer Change place you start counting -l, --last yes, an integer Change place you end counting -s, --skip optional, an integer, 1 if not specified Change the amount you add to the counter each iteration -h, --help none Print a help message including these instructions. -j, --joke none Tell a number based joke. So, if your program is called counter counter -f 10 --last 4 --skip 2 should produce 10, 8, 6, 4 Please use the last supplied argument. If your code is called counter, counter -f 4 -f 5 -f 6 should count from 6. You should count from first to last inclusively. You…arrow_forwardWas What is the deference betwem full At Adber and Hold?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
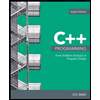
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
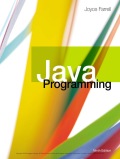
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
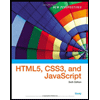
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
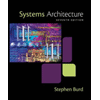
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
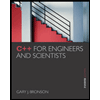
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
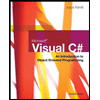
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,