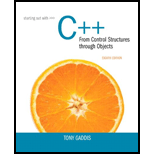
Concept explainers
Purpose of the given code:
The given code is trying to remove a value from the linked list; the value to be removed is stored in the variable “num”.
Given Code:
//Definition of function
void NumberList::deleteNode(double num)//Line 1
{//Line 2
//Declaration of structure pointer variable
ListNode *nodePtr, *previousNode; //Line 3
// If the list is empty, do nothing
if (!head)//Line 4
return;//Line 5
//Determine if the first node is the one
if (head->value == num)//Line 6
//Error #1
//Delete “head” value
delete head;//Line 7
//Error #2
else//Line 8
{//Line 9
// Initialize nodePtr to head of list
nodePtr = head;//Line 10
/* Skip all nodes whose value member is not equal to num */
while (nodePtr->value != num)//Line 11
{//Line 12
previousNode = nodePtr;//Line 13
nodePtr = nodePtr->next;//Line 14
}//Line 15
/* link the previous node to the node after nodePtr, then delete nodePtr */
previousNode->next = nodePtr->next;//Line 16
delete nodePtr;//Line 17
}//Line 18
}//Line 19

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
- Refer to page 75 for graph-related problems. Instructions: • Implement a greedy graph coloring algorithm for the given graph. • Demonstrate the steps to assign colors while minimizing the chromatic number. • Analyze the time complexity and limitations of the approach. Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 150 for problems on socket programming. Instructions: • Develop a client-server application using sockets to exchange messages. • Implement both TCP and UDP communication and highlight their differences. • Test the program under different network conditions and analyze results. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 80 for problems on white-box testing. Instructions: • Perform control flow testing for the given program, drawing the control flow graph (CFG). • Design test cases to achieve statement, branch, and path coverage. • Justify the adequacy of your test cases using the CFG. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]arrow_forward
- Refer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 60 for solving the Knapsack problem using dynamic programming. Instructions: • Implement the dynamic programming approach for the 0/1 Knapsack problem. Clearly define the recurrence relation and show the construction of the DP table. Verify your solution by tracing the selected items for a given weight limit. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 70 for problems related to process synchronization. Instructions: • • Solve a synchronization problem using semaphores or monitors (e.g., Producer-Consumer, Readers-Writers). Write pseudocode for the solution and explain the critical section management. • Ensure the solution avoids deadlock and starvation. Test with an example scenario. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward15 points Save ARS Consider the following scenario in which host 10.0.0.1 is communicating with an external SMTP mail server at IP address 128.119.40.186. NAT translation table WAN side addr LAN side addr (c), 5051 (d), 3031 S: (e),5051 SMTP B D (f.(g) 10.0.0.4 server 138.76.29.7 128.119.40.186 (a) is the source IP address at A, and its value. S: (a),3031 D: (b), 25 10.0.0.1 A 10.0.0.2. 1. 138.76.29.7 10.0.0.3arrow_forward6.3A-3. Multiple Access protocols (3). Consider the figure below, which shows the arrival of 6 messages for transmission at different multiple access wireless nodes at times t=0.1, 1.4, 1.8, 3.2, 3.3, 4.1. Each transmission requires exactly one time unit. 1 t=0.0 2 3 45 t=1.0 t-2.0 t-3.0 6 t=4.0 t-5.0 For the CSMA protocol (without collision detection), indicate which packets are successfully transmitted. You should assume that it takes .2 time units for a signal to propagate from one node to each of the other nodes. You can assume that if a packet experiences a collision or senses the channel busy, then that node will not attempt a retransmission of that packet until sometime after t=5. Hint: consider propagation times carefully here. (Note: You can find more examples of problems similar to this here B.] ☐ U ப 5 - 3 1 4 6 2arrow_forward
- Just wanted to know, if you had a scene graph, how do you get multiple components from a specific scene node within a scene graph? Like if I wanted to get a component from wheel from the scene graph, does that require traversing still? Like if a physics component requires a transform component and these two component are part of the same scene node. How does the physics component knows how to get the scene object's transform it is attached to, this being in a scene graph?arrow_forwardHow to develop a C program that receives the message sent by the provided program and displays the name and email included in the message on the screen?Here is the code of the program that sends the message for reference: typedef struct { long tipo; struct { char nome[50]; char email[40]; } dados;} MsgStruct; int main() { int msg_id, status; msg_id = msgget(1000, 0600 | IPC_CREAT); exit_on_error(msg_id, "Creation/Connection"); MsgStruct msg; msg.tipo = 5; strcpy(msg.dados.nome, "Pedro Silva"); strcpy(msg.dados.email, "pedro@sapo.pt"); status = msgsnd(msg_id, &msg, sizeof(msg.dados), 0); exit_on_error(status, "Send"); printf("Message sent!\n");}arrow_forward9. Let L₁=L(ab*aa), L₂=L(a*bba*). Find a regular expression for (L₁ UL2)*L2. 10. Show that the language is not regular. L= {a":n≥1} 11. Show a derivation tree for the string aabbbb with the grammar S→ABλ, A→aB, B→Sb. Give a verbal description of the language generated by this grammar.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
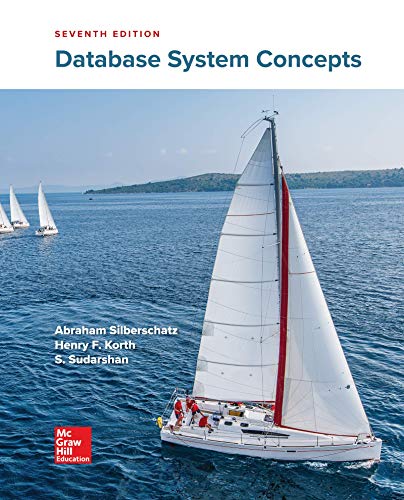
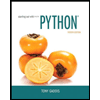
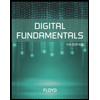
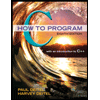
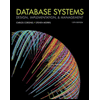
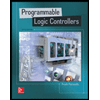