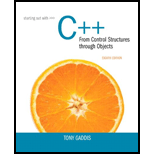
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 24RQE
Program Plan Intro
Standard Template Library (STL) list container:
The STL includes the collection of data types and algorithms which can be used by the programmer in their programs.
The STL “list” container is a template version of “doubly” linked list. The elements of the container can iterate either forward or backward. It can grow at either front or back of the list.
The header file named “#include<list>” is used to implement the list container in a
Syntax:
Syntax to insert values into “STL list” container is as follows:
variable_name.push_back(value);
- “variable_name” is the name of the list which is defined by user.
- “push_back(value)” is a method to add a new element containing a “value” at end of the container.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(1 point)
By dragging statements from the left column to the right column below, give a proof by induction of the following statement:
an
=
= 9" - 1 is a solution to the recurrence relation an = 9an-18 with ao
=
: 0.
The correct proof will use 8 of the statements below.
Statements to choose from:
Note that a₁ =
9a0 + 8.
Now assume that P(n) is true for all n ≥ 0.
Your Proof: Put chosen statements in order in this
column and press the Submit Answers button.
Let P(n) be the predicate, "a = 9″ – 1".
απ = 90 − 1 =
Note that
Let P(n) be the predicate, "an
9" - 1 is a
solution to the recurrence relation an = 9an-1 +8
with ao = 0."
-
Now assume that P(k + 1) is true.
Thus P(k) is true for all k.
Thus P(k+1) is true.
Then ak+1
=
9ak +8, so P(k + 1) is true.
= 1 − 1 = 0, as required.
Then = 9k — 1.
ak
Now assume that P(k) is true for an arbitrary
integer k ≥ 1.
By the recurrence relation, we have
ak+1 =
ak+1 =
= 9ak + 8 = 9(9k − 1) + 8
This simplifies to
9k+19+8 = 9k+1 − 1
Then 9k+1 − 1 = 9(9*…
Given the following request to an AI chatbot, which response is more accurate? (You should use Google to verify that the factual claims in each response are accurate.)
Prompt
Is this true? The first recorded use of the phrase "when pigs fly" in print is in a 1616 play by Ben Jonson called "The Devil is an Ass," in which a character says, "When pigs fly, good-night!!"
Response
Response A
Response B
Yes, that's true! The phrase "when pigs fly" indeed appears in Ben Jonson's 1616 play The Devil is an Ass and was said during the second act in a conversation between Fitzdottrel and Pug. The phrase was first used to express skepticism or disbelief, suggesting that something is as unlikely to happen as the fanciful idea of pigs flying. This usage is often cited as one of the earliest instances of the phrase in print.
The claim that the phrase "when pigs fly" was first recorded in Ben Jonson's 1616 play "The Devil is an Ass" is not accurate. There is no evidence from the…
This is for my Computer Organization & Assembly Language Class
Chapter 17 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Prob. 17.7CPCh. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Prob. 10RQECh. 17 - Prob. 11RQECh. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 19RQECh. 17 - Prob. 20RQECh. 17 - Prob. 21RQECh. 17 - Prob. 22RQECh. 17 - Prob. 23RQECh. 17 - Prob. 24RQECh. 17 - Prob. 25RQECh. 17 - T F The programmer must know in advance how many...Ch. 17 - T F It is not necessary for each node in a linked...Ch. 17 - Prob. 28RQECh. 17 - Prob. 29RQECh. 17 - Prob. 30RQECh. 17 - Prob. 31RQECh. 17 - Prob. 32RQECh. 17 - Prob. 33RQECh. 17 - Prob. 34RQECh. 17 - Prob. 35RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - List Template Create a list class template based...Ch. 17 - Prob. 9PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Prob. 13PCCh. 17 - Prob. 14PCCh. 17 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please answer the homework scenario below and make a JAVA OOP code. You have been hired by GMU to create and manage their course registration portal. Your first task is to develop a program that will create and track different courses in the portal. Each course has the following properties: • a course number ex. IT 106, IT 206, • A course description, ex. Intro to Programming • Total credit hour ex. 3.0, and • current enrollment ex. 30 Each course must have at least a course number and credit hours. The maximum enrollment for each course is 40 students. The current enrollment should be no greater than the maximum enrollment. A course can have a maximum of 4 credit hour. The DDC should calculate the number of seats remaining for the course. Design an object-oriented solution to create a data definition class for the course object. The course class must define all the constructors, mutators with proper validation, accessors, and special purpose methods. The DDC should calculate the…arrow_forwardFor this case study, students will analyze the ethical considerations surrounding artificial intelligence and big data in healthcare, as explored in the case study found in the textbook (pages 34-36) and in the extended version available here There will also be additional articles in this weeks learning module to show both sides of the coin. https://www.delftdesignforvalues.nl/wp-content/uploads/2018/03/Saving-the-life-of-medical-ethics-in-the-age-of-AI-and-Big-Data.pdf Students should refer to the syllabus for specific guidelines regarding length, format, and content requirements. Reflection Questions to Consider: What are the key ethical dilemmas presented in the case? How does AI challenge traditional medical ethics principles such as autonomy, beneficence, and confidentiality? In what ways can responsible innovation help address moral overload in healthcare decision-making? What are the potential risks and benefits of integrating AI-driven decision-making into patient care?…arrow_forwardCan you please solve this. Thanksarrow_forward
- can you solve this pleasearrow_forwardIn the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forwardIn the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forward
- Send me the lexer and parserarrow_forwardHere is my code please draw a transition diagram and nfa on paper public class Lexer { private static final char EOF = 0; private static final int BUFFER_SIZE = 10; private Parser yyparser; // parent parser object private java.io.Reader reader; // input stream public int lineno; // line number public int column; // column // Double buffering implementation private char[] buffer1; private char[] buffer2; private boolean usingBuffer1; private int currentPos; private int bufferLength; private boolean endReached; // Keywords private static final String[] keywords = { "int", "print", "if", "else", "while", "void" }; public Lexer(java.io.Reader reader, Parser yyparser) throws Exception { this.reader = reader; this.yyparser = yyparser; this.lineno = 1; this.column = 0; // Initialize double buffering buffer1 = new char[BUFFER_SIZE]; buffer2 = new char[BUFFER_SIZE]; usingBuffer1 = true; currentPos = 0; bufferLength = 0; endReached = false; // Initial buffer fill fillBuffer(); } private…arrow_forwardIf integer x is divisible by 3, can you prove that ceil(x/2) + floor(x/6) = floor(x/2) + ceil(x/6)arrow_forward
- Draw the NFA for thisarrow_forwardWhat are three examples each of closed-ended, open-ended, and range-of-response questions? thank youarrow_forwardCreate 2 charts using this data. One without using wind speed and one including max speed in mph. Write a Report and a short report explaining your visualizations and design decisions. Include the following: Lead Story: Identify the key story or insight based on your visualizations. Shaffer’s 4C Framework: Describe how you applied Shaffer’s 4C principles in the design of your charts. External Data Integration: Explain the second data and how you integrated it with the Halloween dataset. Compare the two datasets. Attach screenshots of the two charts (Bar graph or Line graph) The Shaffer 4 C’s of Data Visualization Clear - easily seen; sharply defined• who's the audience? what's the message? clarity more important than aestheticsClean - thorough; complete; unadulterated, labels, axis, gridlines, formatting, right chart type, colorchoice, etc.Concise - brief but comprehensive. not minimalist but not verboseCaptivating - to attract and hold by beauty or excellence does it capture…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
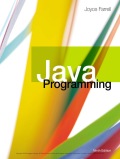
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
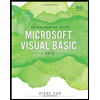
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
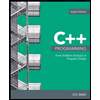
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
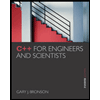
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
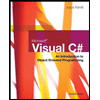
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
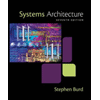
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning