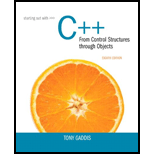
Concept explainers
Purpose of the given code:
The given code is trying to insert the value of the variable “num” into the end of a linked list.
Given Code:
//Definition of function
void NumberList::appendNode(double num)//Line 1
{//Line 2
//Declaration of structure pointer variable
ListNode *newNode, *nodePtr;//Line 3
//Allocate a new node & store num
//Error #1
newNode = new listNode;//Line 4
newNode->value = num;//Line 5
// If there are no nodes in the list
// make newNode the first node
if (!head)//Line 6
head = newNode;//Line 7
// Otherwise, insert newNode at end
else //Line 8
{//Line 9
//Error #2
// Find the last node in the list
while (nodePtr->next)//Line 10
nodePtr = nodePtr->next;//Line 11
// Insert newNode as the last node
nodePtr->next = newNode;//Line 12
}//Line 13
}//Line 14

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
- As described in Learning from Mistakes, the failure of the A380 to reach its sales goals was due to Multiple Choice: a) misunderstanding of supplier demands. b) good selection of hotel in the sky amenities. c) changes in customer demands. d) lack of production capacity.arrow_forwardNumerous equally balanced competitors selling products that lack differentiation in a slow growth industry are most likely to experience high: a) intensity of rivalry among competitors. b) threat of substitute products. c) threat of new entrants. d) bargaining power of suppliers.arrow_forwardA Dia file has been created for you to extend and can be found on Company.dia represents a completed ER schema which, models some of the information implemented in the system, as a starting point for this exercise. Understanding the ER schema for the Company database. To demonstrate that you understand the information represented by the schema, explain using EMPLOYEE, DEPARTMENT, PROJECT and DEPENDENT as examples: attributes, entities and relationships cardinality & participation constraints on relationships You should explain questions a and b using the schema you have been given to more easily explain your answers. Creating and Extending Entity Relationship (EER) Diagrams. To demonstrate you can create entity relationship diagrams extend the ER as described in Company.dia by modelling new requirements as follows: Create subclasses to extend Employee. The employee type may be distinguished further based on the job type (SECRETARY, ENGINEER, MANAGER, and TECHNICIAN) and based…arrow_forward
- Computer programs can be very complex, containing thousands (or millions) of lines of code and performing millions of operations per second. Given this, how can we possibly know that a particular computer program's results are correct? Do some research on this topic then think carefully about your response. Also, explain how YOU would approach testing a large problem. Your answer must be thoughtful and give some insight into why you believe your steps would be helpful when testing a large program.arrow_forwardCould you fix this? My marker has commented, What's missing? The input list is the link below. https://gmierzwinski.github.io/bishops/cs321/resources/CS321_Assignment_1_Input.txt result.put(true, dishwasherSum); result.put(false, sinkSum); return result; }}arrow_forwardPLEG136: Week 5 Portofolio Project Motion to Compelarrow_forward
- B A E H Figure 1 K Questions 1. List the shortest paths between all node pairs. Indicate the number of shortest paths that pass through each edge. Explain how this information helps determine edge betweenness. 2. Compute the edge betweenness for each configuration of DFS. 3. Remove the edge(s) with the highest betweenness and redraw the graph. Recompute the edge betweenness centrality for the new graph. Explain how the network structure changes after removing the edge. 4. Iteratively remove edges until at least two communities form. Provide step-by-step calculations for each removal. Explain how edge betweenness changes dynamically during the process. 5. How many communities do you detect in the final step? Compare the detected communities with the original graph structure. Discuss whether the Girvan- Newman algorithm successfully captures meaningful subgroups. 6. If you were to use degree centrality instead of edge betweenness for community detection, how would the results change?arrow_forwardUnit 1 Assignment 1 – Loops and Methods (25 points) Task: You are working for Kean University and given the task of building an Email Registration System. Your objective is to generate a Kean email ID and temporary password for every new user. The system will prompt for user information and generate corresponding credentials. You will develop a complete Java program that consists of the following modules: Instructions: 1. Main Method: ○ The main method should include a loop (of your choice) that asks for input from five users. For each user, you will prompt for their first name and last name and generate the email and password by calling two separate methods. Example о Enter your first name: Joe Enter your last name: Rowling 2.generateEmail() Method: This method will take the user's first and last name as parameters and return the corresponding Kean University email address. The format of the email is: • First letter of the first name (lowercase) + Full last name (lowercase) +…arrow_forwardI have attached my code, under I want you to show me how to enhance it and make it more cooler and better in graphics with following the instructions.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
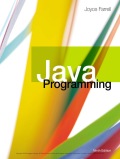
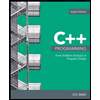
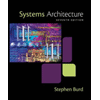
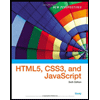
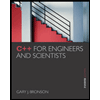