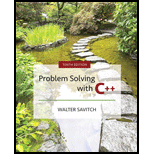
Display 7.10 shows a function called search, which searches an array for a specified integer. Give a function template version of search that can be used to search an array of elements of any type. Give both the function declaration and the function definition for the template. (Hint: It is almost identical to the function given in Display 7.10.)

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Management Information Systems: Managing The Digital Firm (16th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
SURVEY OF OPERATING SYSTEMS
Degarmo's Materials And Processes In Manufacturing
- Write a function that accepts an int array and the array’s size as arguments. The function should create a new array that is two elements larger than the argument array. The first two elements of the new array should be set to 0. Element 0 of the argument array should be copied to element 2 of the array, element 1 of the argument array should be copied to element 3 of the new array, and so forth. The function should return a pointer to the new array. Demonstrate the function in a complete program.arrow_forward1) Write a program in C that stores a list of employee records in an array of structs. Each employee record should contain the following fields: Name (string), Age (int), Salary (float), Department (string). The program should prompt the user to enter the name of an employee, and then search the array of employee records for an employee with a matching name. If a matching employee is found, the program should print out the employee's age, salary, and department. If no matching employee is found, the program should print an error message. The program should use a linear search algorithm to find a matching employee in the array. If the employee's age is greater than 50, their salary should be multiplied by 1.1. If the employee's age is less than or equal to 50, their salary should be divided by 1.2. 2) Write a C program that defines a function called 'multiply_by_two' that takes an integer as an argument and returns the result of multiplying it by 2. The main function of the program…arrow_forwardin c++ Write a user-defined function that takes an array and the size of the array as parameters, and returns the number of elements whose values are between 10 and 20 in the array. Define and initialize an array with 10 elements in main() function and use your user-defined function to display the result.arrow_forward
- C++ Write a function named “getTotalHourAndPayout” that accepts an array ofPayStub object pointers and its size. It will return the total number of hours andpayout amount for all of the PayStub in the given array. For example, if we have two paystubs of 40 hours and $10 pay rate with 50 hoursand $10 pay rate, it will return 90 hours and $950.Please show you would call and test this function.arrow_forwardLAB 16.3 Total Template Write a template for a function called total. The function should keep a running total values entered by user, then return the total. The argument sent into the function should be the number of values the function is to read. Test the template in a simple driver program that sends values of various types as arguments and displays the results.arrow_forwardC++ Write a function that accepts an array and its size as arguments, creates a new array that is a copy of the argument array, and returns a pointer to the new array. Display the array in main(). The function will work as follows: Accept an array and its size as arguments. Dynamically allocate a new array that is the same size as the argument array. Copy the elements of the argument array to the new array. Return a new pointer to the new array. // This program uses a function to duplicate// an int array of any size.#include <iostream>using namespace std;// Function prototype_________________________________________________int main(){ // Define constants for the array sizes. const int SIZE1 = 5, SIZE2 = 7, SIZE3 = 10; // Define three arrays of different sizes. int array1[SIZE1] = { 100, 200, 300, 400, 500 }; int array2[SIZE2] = { 10, 20, 30, 40, 50, 60, 70 }; int array3[SIZE3] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; // Define three pointers for the duplicate arrays. int *dup1 =…arrow_forward
- C++ Write a function, insertAt, that takes four parameters: an array of integers, the number of elements in the array, an integer (say, insertItem), and an integer (say, index). The function should insert insertItem in the array provided at the position specified by index. If index is out of range, output the following: Position of the item to be inserted is out of range or if the index is negative: Position of the item to be inserted must be nonnegative (Note that index must be between 0 and the number of elements in the array; that is, 0 <= index < the number of elements in the array.) Assume that the array is unsorted. int list[ARRAY_SIZE] = {4, 33, 25, 68, 50, 30, 63, 47, 54, 86, 82, 71};arrow_forwardExercise #1: Write a program with a class that contains an array of integers. Initialize the integer array in the constructor of the class. Then create two friend functions to the class to find the largest and smallest integers in the array. Create a destructor that sets all of the elements in the array to 0.arrow_forwardC++ 1. Create a vector of 100 randomly generated integers. Use the count_if function and a lambda function to count the number of even numbers in the vector.2. Repeat Problem 1 for odd numbers.3. Write a function object that prints a number if it is less than 35. Use the function object with the for_each function to display the numbers greater than 50 created in one of the vectors from the problems above.arrow_forward
- Please Help!!!! write in c++ languagearrow_forwardc++ First, write a function which will find the sum of all elements of one-dimensional array: 2.0,4.0,6.0,8.0,10.0 declared and list initialized in main(). Then use a call by reference in main(), and display the value of the sum as the function’s return value.arrow_forwardWrite a void function that prints the list of nonnegative integers in reverse. Write a void function that prints all the numbers stored in the list that are greater than 10. It will also print the number of such numbers found. Write a function that determines the largest value stored in the array and returns that value to the calling function main. Write a function that determines the number of even integers stored in the array and returns that value to the calling function main. Write a function that calculates the average of the values stored in the array and returns that value. to the calling function main. Write the function calls with the appropriate arguments in the function main. Any values returned will be output. Write appropriate block comments for each function header similar to the ones provided. Show your understanding of the program and thus provide useful information to the reader. Test all the functions using appropriate test data. Use the following code and edit it…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
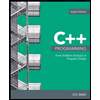