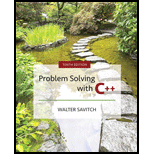
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17, Problem 3P
Program Plan Intro
Recursive Binary Search
Program Plan:
- Include the required header files.
- Declare the global variables.
- Declare the function template.
- Define main function.
- Declare the required variables.
- Get the array of elements.
- Display the given display.
- Sort the array in ascending order.
- Display the sorted array.
- Call the “search” function with the arguments.
- If the “found” value is true, display the search element location. Otherwise display, “the element is not found”.
- Define the “search” function
- Declare the variable
- If “first” is greater than “last”, set “found” value as “false”.
- Otherwise find the “mid” value.
- If the “key” value is equal to “a [mid]”, set “found” value as “true” and also set “location” as “mid”.
- If the “key” is less than “a [mid]”, call the “search” function with the arguments.
- Otherwise, call the “search” function with the arguments.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Need help with this in python!
Need help with this in python!
Help! How do I turn the flowchart that searches for a name in an array of names into structured and spaced pseudocode?
Chapter 17 Solutions
Problem Solving with C++ (10th Edition)
Ch. 17.1 - Write a function template named maximum. The...Ch. 17.1 - Prob. 2STECh. 17.1 - Define or characterize the template facility for...Ch. 17.1 - Prob. 4STECh. 17.1 - Display 7.10 shows a function called search, which...Ch. 17.1 - Prob. 6STECh. 17.2 - Give the definition for the member function...Ch. 17.2 - Give the definition for the constructor with zero...Ch. 17.2 - Give the definition of a template class called...Ch. 17.2 - Is the following true or false? Friends are used...
Ch. 17 - Write a function template for a function that has...Ch. 17 - Prob. 2PCh. 17 - Prob. 3PCh. 17 - Redo Programming Project 3 in Chapter 7, but this...Ch. 17 - Display 17.3 gives a template function for sorting...Ch. 17 - (This project requires that you know what a stack...Ch. 17 - Prob. 6PPCh. 17 - Prob. 7PPCh. 17 - This project requires that you complete...
Knowledge Booster
Similar questions
- The mail merge process has ____ steps. Question 19Select one: a. five b. six c. seven d. eightarrow_forwardIf you created a main document based on an existing document entitled "Confirmation Letter," what default filename would Word give the main document? Question 14Select one: a. Confirmation Letter-1 b. Confirmation Letter-merge c. Document1 d. MergedDocument1arrow_forwardClick the ____ option button in the Mail Merge task pane to use an Outlook contact list as a data source for a merge. Question 11Select one: a. Use Outlook contacts list b. Select from Outlook contacts c. Select Contacts d. Mail Merge Recipientsarrow_forward
- A(n) ____ cannot be selected as the document type in the Mail Merge task pane. Question 9Select one: a. Letter b. Directory c. Fax d. E-mail messagearrow_forwardConsider a Superstore Database which consists of 3 tables, Orders, Returns, and Managers. The CSV files have been provided along with this DOC file in the Midterm 2 Link in the Moodle. Answer the questions as below: Use the created table as in the provided SQL query file, solve the problems as mentioned below. You will have to import the respective CSV files of the above created tables as without them, it is impossible to solve the questions below. If you are not able to upload the files successfully, do not leave the query questions. Just write the query to the best of your knowledge. Do not copy. To be graded for the screenshot answer, you must upload the CSV properly and paste the resulting screenshot of the queries as asked. Write Query to Find out which Product Sub-Category has a sum of Shipping Cost to sum of Sales ratio > 0.03.arrow_forwardI need to render an image of a car continuously for a smooth visual experience in C# WinForms. It gets the location array (that has all the x,y of the tiles it should visit) from another function - assume it is already written.arrow_forward
- write c program with features: Register a Bunny: Store the bunny's name, poem, and initialize the egg count to 0. Modify an Entry: Change the bunny's poem or update the egg count. Delete a Bunny: Remove a registered bunny from the list. List All Bunnies: Display all registered bunnies and their details. Save & Load Data: Store bunny data in a file to persist between runs. Use a struct to represent a bunny contestant. Store data in a binary file (bunnies.dat) for persistence. Use file I/O functions (fopen, fwrite, fread, etc.) to manage data. Implement a menu-driven interface for user interaction.arrow_forwardHelp, how do I write the pseudocode for the findMean function and flowchart for this?arrow_forwardNeed help drawing a flowchart for the findMax function herearrow_forward
- Need help writing the pseudocode for the findMin function with attachedarrow_forwardCreate a static function in C# where poachers appear and attempt to hunt animals. It gets the location of the closest animal to itself. Take account of that the animal also move too, so it should update the closest location (x, y) everytime it moves to a new location. Use winforms to show the movements of poachers.arrow_forwardCreate a static function in C# where poachers appear and attempt to hunt animals. It gets the location of the closest animal to itself. Take account of that the animal also moves too, so it should update the closest location (x, y) everytime it moves to a new location. Use winforms to show to movementsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
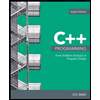
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
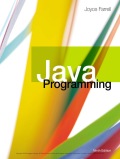
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
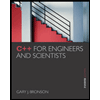
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
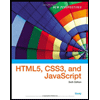
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning