2. Write a function that has one parameter which is an array of type double with 50 elements. The function should find the last negative number in the array and return its position.
C++ CODE


#include <iostream>
using namespace std;
// Function to find the last negative element in the array
int lastNegativeElement(double arr[50])
{
int position = -1;
for(int i=0;i<50;i++)
{
if(arr[i] < 0)
position = i;
}
return position;
}
int main() {
// Sample data
double arr[50] = {-948630,-578534.56,-649554.02,49130.57,334363.1,-923244.29,763984,836428.24,-462928.55,265469.19,134502.9,575783.55,-980748.56,708466.06,-317969.25,-623110.81,891693.06,628348.37,-130653.19,468568.65,-984079.47,514914.37,-540028.5,-431829.17,-107169.59,-625668.97,92070.96,703979.73,724532,439440.94,305218.09,-126932,90748.16,-623916.1,-256320.85,-13132.66,-860979.45,-320049.2,-204178.51,-230405.18,-684693,613560.3,-270117.21,-924169.47,640937.26,-481748.62,187378,-557640.3,787299.52,282284.32};
int position = lastNegativeElement(arr);
// Check position if there is a negative element or not
if(position != -1){
cout<<"\n Postion of last negative element : "<<position+1;
}
else
{
cout<<"\n No negative element present in the array.";
}
return 0;
}
Step by step
Solved in 2 steps with 1 images

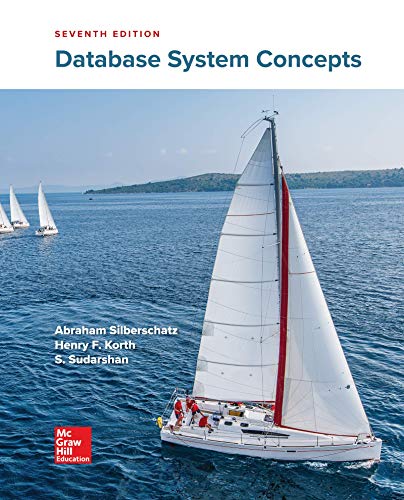
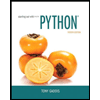
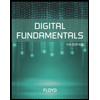
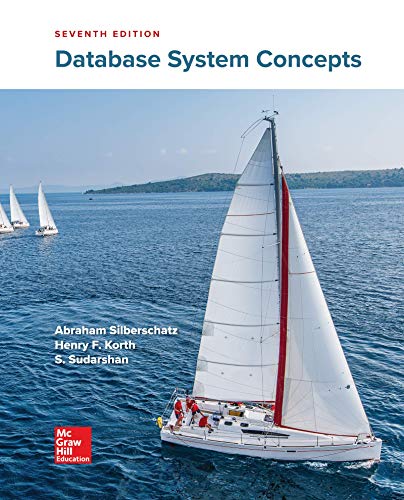
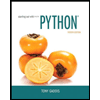
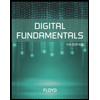
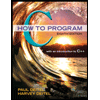
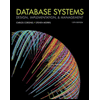
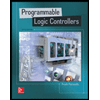