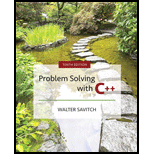
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 16.1, Problem 7STE
Program Plan Intro
Exception:
An exception is a problem that creates during the execution of a program; it offers a method to transfer control from one part to another part of a program.
An exception handling is created by using the following three keywords such as try, catch and throw.
- The “try” block have the program for the basic
algorithm that says the computer what to do when all goes well. - The “throw” keyword throws an error statement to the “catch” block.
- The “catch” block will catch the exception or handling the exception.
Generally, the compiler executes “try” block. In the “try” block, if the statements cause an exception, it throws an error statement to the “catch” block using the keyword “throw”. The “catch” block then handles the error based upon the type of exception.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Using C++
Write a void function GetYear that prompts for and inputs the year the operator was born (type int) from standard input. The function returns the user’s birth year through the parameter list (use pass by reference) unless the user enters an invalid year, in which case a BadYear exception is thrown. To test for a bad year, think about the range of acceptable years. It must be 4 digits (i.e. 1982) and it cannot be greater than the current year (the user obviously cannot be born in a calander year that has yet to happen!
What is the value stored at x, given the statements?
int x;
x = 6 / static_cast<int> (4.5 + 6.4);
Consider the following code:
def test(*x):
for k in x:
print(type(k))
what will be the output if test function is culled with 876?
Oa.
O b.
O c.
O d. 876
Chapter 16 Solutions
Problem Solving with C++ (10th Edition)
Ch. 16.1 - Prob. 1STECh. 16.1 - What would be the output produced by the code in...Ch. 16.1 - Prob. 3STECh. 16.1 - What happens when a throw statement is executed?...Ch. 16.1 - In the code given in Self-Test Exercise 1, what is...Ch. 16.1 - Prob. 6STECh. 16.1 - Prob. 7STECh. 16.1 - What is the output produced by the following...Ch. 16.1 - What is the output produced by the program in...Ch. 16.2 - Prob. 10STE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Which of the following members take values in test object: * Class Test (int xyzı; Static int xyz;; Static int sum(){} Void av(){} }test; а-хуz1 b-хуz2 C- sum d- av e- both a&barrow_forwardPF Assignment 3 BS ERP 105903 Submission date: 18th April, 2021 via LMS Assignment should include:Hand Wriiten Coding + Screenshot of Output (a) Create a method GetMax() which takes two integer (int) parameters as an arguments and returns maximal of the two numbers. (b) Write a program that reads three numbers from the console and prints the biggest of them. Use the GetMax() method you just created. Write a test program that validates that the methods works correctly. Write a method that returns the digits of a given decimal number in a reversed order. For example 256, must be printed as 652. Write a program in C to check a given number is positive or negative using method. Write a program that calculates and prints the n! for any n in the range [1…10] number should be taken as input from user through main and should pass as an argument to the method. Write a program in C# Sharp to create a function to input a string and return count of number of spaces are in the…arrow_forward6. Which of the below code contains correct overloading code? Why would the other won't work? Code 1 double myMultiply. (int x){ return 10 x; Code 2 int awMultielx (int x){ return 10 x; } double yMultirly. (double x){ double yMultiely (int x){ return 20 x; return 2e * x; 7. What would be the output for the following program and why ( explain in short): Bublis static void nain(String acsal))( String."; String si - "Testing Java Program String s2 - sukstriui13); 8. Write the code in Java for the following class from the UML diagram below: Vehicle -passengers: int -maxSpeed: double -color: String +Vehiclelint,double.String) +setColor(): void +getColor(): String +findCaracityl): double The findCapacityl) method return the capacity of the vehicle by the following formula: Capacity = passengers * 75 L0.20 * maxSpeed)arrow_forward
- Java Language How can I create a test in JUnit for this setter? public void setAge(int age) throws IllegalArgumentException{ if ( age < 0 || age > 80 ) throw new IllegalArgumentException("Invalid age entered"); this.age = age; }arrow_forwardjava code quation using ExceptionProgramme Leader of ITMB wants to check whether the student number and GSM numberentered by the student for the programming contest is valid or not.Write a Java program to read the Student ID and GSM Number of a student. Use a methodcalled ValidityDetails () for checking the validity of details entered.If the Student ID ends with the characters ST and contains more than 6 letters or if the MobileNumber does not contain exactly 8 digits, throw a user defined exceptionInvalidDetailsException.If the details entered are valid, display the message ‘”correct details are entered!!!” otherwisedisplay “Entered invalid details!!!!”arrow_forwardConsider the following code: public static void test_b(int n) { if (n>0) test_b(n-2); System.out.println(n + " "); } What is printed by the call test_b(6)?arrow_forward
- What the output forthe code fragments? int i = 7; do{ i = i/2; System.out.println(i); } while (i >0);arrow_forwardEnglish (e what is the output of the following program? #include int test (int &, int); main () { int a=10, b=15; test (b, a); test (a,b); cout<arrow_forward2- Trace the following code and write the output: class Test1 { Test1(int x) { System.out.println("Test Calls " + x); class Test2 { Testi t1 = new Test1(10); Test2(int i) { t1 = new Test1(i); } public static void main(String[] args) { Test2 t2 = new Test2(5);arrow_forwardYou are working on problem set: HW2 - loops (Pause) Pause) ? variableScope ♡ Language/Type: Java expressions for % A variable's scope is the part of a program in which it exists. In Java, the scope of a variable starts when it is declared and ends when the closing curly brace for the block that contains it is reached. A variable is said to be in scope where it is accessible. Consider the following program: public class Example { public static void main(String[] args) { performTest (); } } public static void performTest() { int count = 12: for (int i = 1; i <= 12; i++) { runSample(); K } } } System.out.print(count); public static void runSample() { System.out.print("sample"); 7 с M 31 In which of these blocks is the variable count in scope for the entirety of the block? runSample method a. b. main method c. for loop d. O performTest method (order shuffled) In which of these blocks is the variable i in scope for the entirety of the block? a. O main method b. O runSample method c. O…arrow_forwardComplete the code below according to the instructions below and the example test: method withdraw throws an exception if amount is greater than balance. For example: Test Result Account account = new Account("Acct-001","Juan dela Cruz", 5000.0); account.withdraw(5500.0); System.out.println("Balance: "+account.getBalance()); Insufficient: Insufficient funds. Balance: 5000.0 Account account = new Account("Acct-001","Juan dela Cruz", 5000.0); account.withdraw(500.0); System.out.println("Balance: "+account.getBalance()); Balance: 4500.0 Incomplete java code: public class Account{ private String accntNumber; private String accntName; private double balance; public Account(){} public Account(String num, String name, double bal){ accntNumber = num; accntName = name; balance = bal; } public double getBalance(){ return balance;}}//your class herearrow_forwardEvaluating Outputarrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
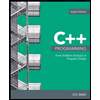
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
.2: Function Parameters and Arguments - p5.js Tutorial; Author: The Coding Train;https://www.youtube.com/watch?v=zkc417YapfE;License: Standard Youtube License